How is internationalization handled in Flutter applications?
Internationalization (i18n) in Flutter is the process of adapting an application to support multiple languages and cultural conventions, providing a seamless experience for users worldwide. Flutter simplifies this with the `intl` package and the use of `arb` (Application Resource Bundle) files.
To enable internationalization, developers typically follow these key steps:
- Import the `intl` package:
In your `pubspec.yaml` file, include the `intl` package. This package provides localization and internationalization support.
```yaml dependencies: flutter: sdk: flutter intl: ^0.17.0 ```
- Define localization delegate:
Create a `LocalizationsDelegate` that loads translated messages. Flutter’s `Intl` library includes the `IntlDelegate` for this purpose.
```dart class MyLocalizationsDelegate extends LocalizationsDelegate<MyLocalizations> { // ... } ```
- Create arb files:
For each supported language, create an arb file containing translated messages. For example, `strings_en.arb` for English and `strings_fr.arb` for French.
```json { "greet": "Hello, world!", "welcome": "Welcome, {name}!", } ```
- Load and display localized messages:
In your app, load and display messages based on the user’s locale.
```dart String greeting = MyLocalizations.of(context).greet; ```
You can also interpolate values dynamically, such as the user’s name in the welcome message.
```dart String welcomeMessage = MyLocalizations.of(context).welcome(name: 'John'); ```
- Change app locale:
Allow users to switch between supported languages by changing the app’s locale.
```dart MyApp.setLocale(BuildContext context, Locale newLocale) { setState(() { _locale = newLocale; }); } ```
By following these steps, Flutter applications can seamlessly adapt to different languages, fostering a more inclusive and accessible user experience on a global scale. Internationalization in Flutter is a crucial aspect of creating applications that resonate with diverse audiences.
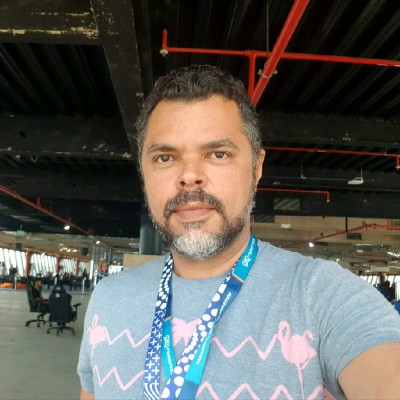
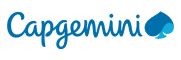