Creating a Job Search App with Flutter: Filters and Job Listings
Flutter is an excellent framework for building cross-platform mobile applications with a single codebase. In this article, we’ll explore how to create a job search app using Flutter, focusing on implementing filters and displaying job listings. This guide will help you develop a dynamic and user-friendly job search application.
Understanding the Job Search App
A job search app typically involves several core features, including the ability to search for jobs, apply filters to refine search results, and view detailed job listings. Flutter’s rich set of widgets and libraries makes it an ideal choice for building such an app.
Building the Job Search App with Flutter
Here’s a step-by-step guide to building a job search app with Flutter, focusing on filters and job listings:
1. Setting Up Your Flutter Project
Before you start coding, ensure you have Flutter installed and set up. Create a new Flutter project using the following command:
```bash flutter create job_search_app ```
Navigate to the project directory:
```bash cd job_search_app ```
2. Designing the User Interface
Design the user interface to include a search bar, filter options, and a list to display job listings. Flutter’s `ListView` and `DropdownButton` widgets are useful for these purposes.
Example: Creating a Search Bar
```dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: JobSearchPage(), ); } } class JobSearchPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Job Search')), body: Column( children: <Widget>[ Padding( padding: const EdgeInsets.all(16.0), child: TextField( decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Search Jobs', ), ), ), // Add filter and job listing widgets here ], ), ); } } ```
3. Implementing Filters
Filters allow users to refine job searches based on criteria like job type, location, or experience level. You can use Flutter’s `DropdownButton` or `Checkbox` widgets for filters.
Example: Adding a Location Filter
```dart class JobSearchPage extends StatefulWidget { @override _JobSearchPageState createState() => _JobSearchPageState(); } class _JobSearchPageState extends State<JobSearchPage> { String _selectedLocation = 'All Locations'; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Job Search')), body: Column( children: <Widget>[ Padding( padding: const EdgeInsets.all(16.0), child: DropdownButton<String>( value: _selectedLocation, onChanged: (String? newValue) { setState(() { _selectedLocation = newValue!; }); }, items: <String>['All Locations', 'New York', 'San Francisco', 'Los Angeles'] .map<DropdownMenuItem<String>>((String value) { return DropdownMenuItem<String>( value: value, child: Text(value), ); }).toList(), ), ), // Add job listings widget here ], ), ); } } ```
4. Displaying Job Listings
To display job listings, use the `ListView.builder` widget to create a dynamic list of job entries.
Example: Displaying Job Listings
```dart class JobSearchPage extends StatelessWidget { final List<String> jobListings = ['Software Engineer', 'Product Manager', 'UI/UX Designer']; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Job Search')), body: Column( children: <Widget>[ // Search bar and filter widgets Expanded( child: ListView.builder( itemCount: jobListings.length, itemBuilder: (context, index) { return ListTile( title: Text(jobListings[index]), onTap: () { // Navigate to job details page }, ); }, ), ), ], ), ); } } ```
5. Implementing Search and Filter Logic
Add functionality to filter job listings based on user input. This involves updating the list of job listings according to the selected filters and search terms.
Example: Filtering Job Listings
```dart class _JobSearchPageState extends State<JobSearchPage> { String _selectedLocation = 'All Locations'; List<String> _filteredJobListings = ['Software Engineer', 'Product Manager', 'UI/UX Designer']; void _filterJobs() { // Implement filtering logic based on _selectedLocation // Update _filteredJobListings accordingly } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Job Search')), body: Column( children: <Widget>[ // Search bar and filter widgets Expanded( child: ListView.builder( itemCount: _filteredJobListings.length, itemBuilder: (context, index) { return ListTile( title: Text(_filteredJobListings[index]), onTap: () { // Navigate to job details page }, ); }, ), ), ], ), ); } } ```
Conclusion
Creating a job search app with Flutter involves designing an intuitive interface, implementing effective filters, and displaying job listings dynamically. Flutter’s powerful widgets and libraries make it easier to build a functional and user-friendly job search application.
Further Reading:
Table of Contents
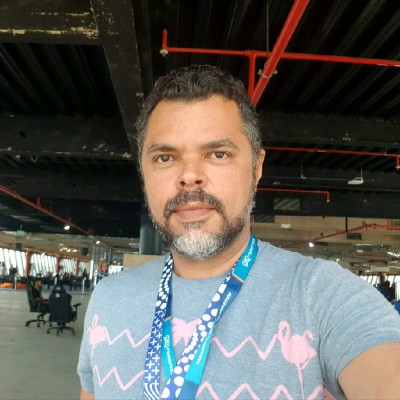
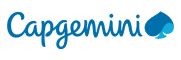