Localization and Internationalization in Flutter: Reaching Global Users
In today’s interconnected world, reaching a global audience is a priority for app developers. Flutter, Google’s UI toolkit, makes this process easier by providing robust support for localization and internationalization. In this comprehensive guide, we’ll explore the concepts, best practices, and code samples you need to effectively localize and internationalize your Flutter app, ensuring it resonates with users worldwide.
Table of Contents
1. Understanding Localization and Internationalization
1.1. What is Localization?
Localization, often abbreviated as “l10n,” is the process of adapting your app to a specific locale or region, including translating its content, such as text and images, into the target language(s). Localization goes beyond just language; it considers cultural differences, date formats, currency, and other region-specific aspects, ensuring that your app feels native to users from different regions.
1.2. What is Internationalization?
Internationalization, abbreviated as “i18n,” is the process of designing your app in a way that makes it easy to adapt and support multiple languages and regions without changing the core code. It involves separating the app’s code from its content, allowing for easy translation and adaptation for different locales. Internationalization sets the foundation for localization.
1.3. Why Localization and Internationalization Matter
Expanding your app’s reach to international markets comes with several benefits:
- Increased User Base: By supporting multiple languages and regions, you open the doors to a broader audience, potentially increasing your app’s user base significantly.
- Improved User Experience: Users are more likely to engage with an app that speaks their language and follows their cultural norms. Localization enhances the overall user experience.
- Global Branding: Successful internationalization and localization can help establish your app as a global brand, boosting its reputation and credibility.
- Market Expansion: Entering new markets can lead to increased revenue and business growth. Effective localization can make your app more appealing to users in these markets.
Now that we understand the importance of localization and internationalization, let’s dive into how you can implement these concepts in your Flutter app.
2. Localization in Flutter
Flutter simplifies the localization process by providing the intl package and tools like flutter_localizations. Here’s how you can get started with localization in Flutter:
2.1. Adding Dependencies
First, add the necessary dependencies to your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter flutter_localizations: sdk: flutter intl: ^0.17.0
2.2. Configure Localizations
In your main.dart file, configure your app to use Flutter’s localization support:
dart import 'package:flutter/material.dart'; import 'package:flutter_localizations/flutter_localizations.dart'; import 'package:intl/intl.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( localizationsDelegates: [ GlobalMaterialLocalizations.delegate, GlobalWidgetsLocalizations.delegate, ], supportedLocales: [ const Locale('en', 'US'), // English const Locale('es', 'ES'), // Spanish // Add more locales as needed ], home: MyHomePage(), ); } }
In this example, we’ve added support for English (United States) and Spanish (Spain). You can extend the supportedLocales list to include other languages.
2.3. Implement Localization
Now, let’s create a simple localized text widget using the intl package:
dart class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text( Intl.message('Hello, World!', name: 'helloWorld', desc: 'The traditional greeting'), ), ), body: Center( child: Text( Intl.message('Welcome to Flutter Localization', name: 'welcomeMessage', desc: 'A welcome message'), ), ), ); } }
In this example, we’re using the Intl.message constructor to define localized messages with names and descriptions. These names are used during the translation process.
2.4. Generating Translation Files
To generate translation files for different languages, use the intl_translation package. First, add it to your pubspec.yaml:
yaml dev_dependencies: flutter_test: sdk: flutter intl_translation: ^0.17.10
Next, run the following command in your project directory:
bash flutter pub pub run intl_translation:extract_to_arb --output-dir=lib/l10n lib/localizations.dart
This command extracts messages marked with Intl.message into an ARB (Application Resource Bundle) file. You’ll find these ARB files in the specified output-dir.
2.5. Translating Your App
Share the ARB files with translators who can provide translations for each locale. Once you have the translated files, use the following command to generate Dart files for each locale:
bash flutter pub pub run intl_translation:generate_from_arb --output-dir=lib/l10n --no-use-deferred-loading lib/localizations.dart lib/l10n/intl_*.arb
This command generates Dart files containing translations for each locale.
2.6. Loading Translations
To load translations dynamically, use the intl package and the Intl class:
dart import 'package:flutter/material.dart'; import 'package:intl/intl.dart'; class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text( Intl.message('Hello, World!', name: 'helloWorld', desc: 'The traditional greeting'), ), ), body: Center( child: Text( Intl.message('Welcome to Flutter Localization', name: 'welcomeMessage', desc: 'A welcome message'), ), ), ); } }
With this setup, your app will automatically load the appropriate translations based on the user’s device locale.
3. Internationalization in Flutter
Internationalization in Flutter involves designing your app to be language and region-agnostic. Here are some key practices to follow:
3.1. Keep UI Separate from Content
Separate your app’s user interface (UI) from its content. Avoid hardcoding text directly into widgets. Instead, use localized strings. This allows for easier translation and adaptation to different languages.
3.2. Use Flexible Layouts
Different languages can have varying text lengths. Ensure that your app’s layouts are flexible enough to accommodate longer or shorter text without breaking the design.
3.3. Support Right-to-Left Languages
Some languages, like Arabic and Hebrew, are written from right to left (RTL). Make sure your app’s UI can adapt to RTL layouts when needed.
3.4. Handle Dates and Times Properly
Dates, times, and calendars can differ from one region to another. Use Flutter’s date and time formatting utilities to display them correctly based on the user’s locale.
3.5. Currency and Number Formats
Similarly, currency and number formats can vary. Use Flutter’s NumberFormat class to format numbers and currency based on the user’s locale.
3.6. Test with Different Locales
Thoroughly test your app with different locales to ensure that text, layouts, and functionality work as expected. Flutter’s Locale class allows you to simulate different locales during development.
4. Best Practices for Effective Localization and Internationalization
To ensure a smooth localization and internationalization process in Flutter, consider the following best practices:
4.1. Start Early
Begin planning for localization and internationalization from the early stages of development. This makes it easier to design your app with these considerations in mind.
4.2. Use Keys for Widgets
Assign keys to widgets that need to be updated when the language changes. This helps Flutter’s widget tree update correctly when the locale changes.
4.3. Collaborate with Translators
Work closely with professional translators who are familiar with the nuances of each language. Effective communication with translators ensures accurate and culturally sensitive translations.
4.4. Maintain Consistency
Maintain consistency in terminology and tone across different languages. This creates a unified brand image and helps users transition between languages seamlessly.
4.5. Update Content Regularly
As your app evolves, keep translations up to date. New features, changes, or bug fixes may require updates to the translated content.
4.6. Handle Plurals and Gender Variations
Languages often have different rules for plurals and gender-specific terms. Flutter’s intl package provides support for handling these variations.
Conclusion
Expanding your Flutter app’s reach to global users is not just about translating text; it’s about creating a seamless and culturally sensitive user experience. Localization and internationalization are essential steps in achieving this goal. By following best practices and utilizing Flutter’s built-in tools, you can effectively localize and internationalize your app, ensuring that it resonates with users worldwide.
Remember that localization and internationalization are ongoing processes. As your app continues to grow and reach new markets, staying committed to providing a great user experience for all your users, regardless of their language or region, is key to your app’s success.
So, start planning, coding, and collaborating with translators today, and watch your app flourish on the global stage. Happy coding, global developer!
Table of Contents
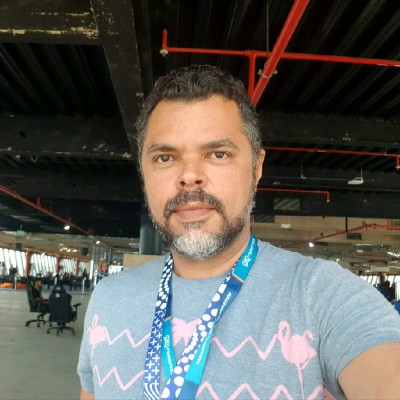
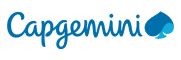