Building a Meditation App with Flutter: Audio Playback and Timer
Meditation apps provide users with a serene experience, often incorporating features like audio playback and timers to facilitate relaxation and mindfulness. This article will guide you through the process of building a meditation app with Flutter, focusing on implementing audio playback and timer functionalities to enhance the user experience.
Understanding Meditation App Features
Meditation apps typically include features such as guided meditation audio, ambient sounds, and timers to help users track their meditation sessions. Flutter, with its rich set of libraries and widgets, makes it straightforward to implement these features in your app.
Implementing Audio Playback in Flutter
To deliver a seamless audio experience, you’ll need to integrate audio playback functionalities into your Flutter app. Flutter provides several packages to handle audio playback effectively.
Example: Adding Audio Playback with `audioplayers` Package
- Add Dependency
Add the `audioplayers` package to your `pubspec.yaml` file:
```yaml dependencies: flutter: sdk: flutter audioplayers: ^0.20.1 ```
- Import the Package
Import the `audioplayers` package in your Dart file:
```dart import 'package:audioplayers/audioplayers.dart'; ```
- Initialize and Play Audio
Create an instance of `AudioPlayer` and use it to play your meditation audio:
```dart AudioPlayer audioPlayer = AudioPlayer(); audioPlayer.play('https://example.com/meditation-audio.mp3'); ```
- Control Playback
Implement controls for play, pause, and stop functionalities:
```dart audioPlayer.pause(); audioPlayer.stop(); ```
For further reading on the `audioplayers` package, visit the [audioplayers documentation](https://pub.dev/packages/audioplayers).
Setting Up a Timer
A timer is essential for tracking meditation session lengths. Flutter provides various ways to implement a timer feature.
Example: Adding a Timer with `flutter_timer` Package
- Add Dependency
Add the `flutter_timer` package to your `pubspec.yaml` file:
```yaml dependencies: flutter: sdk: flutter flutter_timer: ^1.0.0 ```
- Import the Package
Import the `flutter_timer` package in your Dart file:
```dart import 'package:flutter_timer/flutter_timer.dart'; ```
- Initialize and Use Timer
Implement a countdown timer in your app:
```dart TimerController timerController = TimerController(); timerController.startCountdown(duration: Duration(minutes: 10)); ```
- Display Timer
Create a widget to display the remaining time:
```dart TimerWidget(controller: timerController); ```
For further reading on implementing timers, visit the [flutter_timer documentation](https://pub.dev/packages/flutter_timer).
Enhancing User Experience with UI Design
To make your meditation app visually appealing, focus on designing a clean and calming interface. Use Flutter’s rich set of widgets and customization options to create a soothing experience.
Example: Customizing UI
- Use Soft Colors
Choose a color palette that promotes relaxation, such as soft blues and greens.
```dart Color backgroundColor = Colors.lightBlue.shade50; ```
- Implement Relaxing Animations
Add animations to enhance the user experience:
```dart AnimatedContainer( duration: Duration(seconds: 2), color: Colors.lightGreen, ); ```
Conclusion
Building a meditation app with Flutter involves implementing audio playback and timer functionalities to provide users with a calming and effective meditation experience. By utilizing Flutter’s packages and customizing your app’s design, you can create an engaging and relaxing meditation app.
Further Reading:
Table of Contents
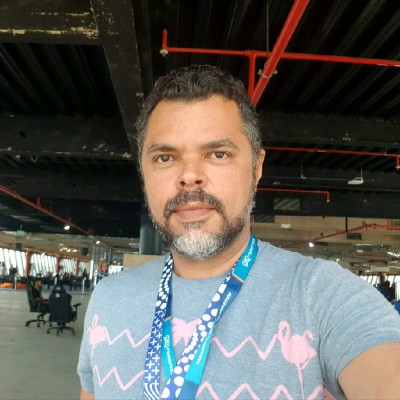
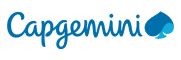