What is a Flutter package and how do you use it?
A Flutter package is a self-contained and reusable unit of code that encapsulates a specific set of functionalities, making it easy to share, distribute, and reuse code across different Flutter projects. These packages can include everything from UI components and themes to utility functions and API integrations. The use of packages is a fundamental aspect of Flutter development, promoting modular and maintainable code.
To use a Flutter package in your project, you typically declare the dependency in the `pubspec.yaml` file, which is a configuration file used by the Dart package manager, Pub. For example, if you wanted to include a popular package like `http` for making HTTP requests, you would add the following to your `pubspec.yaml`:
```yaml dependencies: http: ^0.13.3 ```
Here, `http` is the package name, and `^0.13.3` specifies the version constraint, indicating that your project can use any version of the `http` package that is 0.13.3 or newer.
Once you’ve declared your dependencies, you run `flutter pub get` in the terminal, and Flutter fetches the specified packages, making them available for use in your project. You can then import the package in your Dart code, for example:
```dart import 'package:http/http.dart' as http; // Example HTTP request using the http package void fetchData() async { final response = await http.get(Uri.parse('https://api.example.com/data')); if (response.statusCode == 200) { print('Data: ${response.body}'); } else { print('Failed to fetch data: ${response.statusCode}'); } } ```
Key points to remember about Flutter packages include their role in code organization, ease of sharing code between projects, and the vibrant Flutter community that actively contributes and maintains a wide variety of packages. Leveraging packages enhances productivity, allowing developers to focus on building features rather than reinventing the wheel.
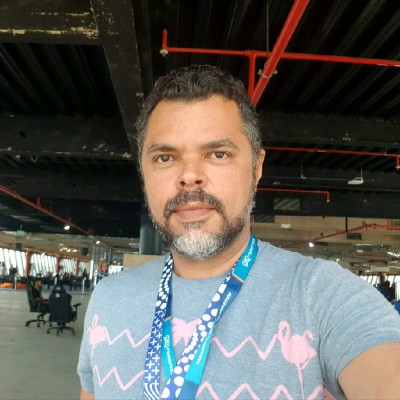
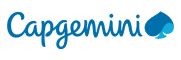