Building a Recipe-Sharing Community with Flutter: User-generated Content
A recipe-sharing community app allows users to submit their own recipes, explore recipes submitted by others, and engage with the content through likes, comments, and shares. Leveraging Flutter, you can build a highly interactive and visually appealing app that supports user-generated content and community interaction.
Building the Recipe-Sharing App with Flutter
Flutter’s flexibility and robust features make it an ideal choice for developing a recipe-sharing community app. Here are key components and examples of how to implement user-generated content and community features in your Flutter app.
1. Designing the User Interface
The first step in building your app is designing an engaging user interface that allows users to browse, submit, and interact with recipes.
Example: Creating Recipe Cards and Submission Forms
- Design Recipe Cards:
– Use Flutter’s `ListView` or `GridView` widgets to display recipes in a card format.
– Implement custom widgets for recipe cards that include images, titles, ingredients, and instructions.
- Create Submission Forms:
– Use `TextField` and `Form` widgets to build forms where users can enter recipe details.
– Implement form validation to ensure that all required fields are completed before submission.
2. Implementing User-Generated Content
Allowing users to generate and share content is central to your app. Flutter provides various tools and packages to manage user-generated content effectively.
Example: Using Firebase for Data Storage
- Integrate Firebase:
– Add Firebase to your Flutter project by following the [Firebase setup guide](https://firebase.google.com/docs/flutter/setup).
- Store Recipes:
– Use Firebase Firestore to store recipe data. Create a collection for recipes and add documents for each recipe submitted by users.
- Manage User Authentication:
– Implement Firebase Authentication to manage user sign-ups and logins. This ensures that each recipe is associated with a specific user.
3. Enabling Recipe Discovery and Interaction
Enhancing user engagement involves providing features for discovering new recipes and interacting with content.
Example: Adding Search and Filtering
- Implement Search Functionality:
– Use the `SearchDelegate` class to create a search bar that allows users to find recipes by keywords.
- Add Filters:
– Implement filter options such as cuisine type, preparation time, or dietary restrictions using dropdown menus or checkboxes.
Example: Adding Social Features
- Likes and Comments:
– Implement like and comment features using Firebase Realtime Database or Firestore. Allow users to interact with recipes and share their feedback.
- Recipe Sharing:
– Add functionality for users to share recipes on social media or through messaging apps.
4. Visualizing Recipe Data
Visualizing recipe data helps users understand trends and discover popular recipes.
Example: Using Charts for Recipe Trends
- Integrate Charts:
– Use packages like [fl_chart](https://pub.dev/packages/fl_chart) to create charts that display recipe popularity, user engagement, or other metrics.
- Show Trends:
– Display graphs or pie charts that show trends in recipe submissions or user interactions over time.
Conclusion
Building a recipe-sharing community with Flutter enables you to create a dynamic and interactive platform where users can share and discover recipes. By focusing on a well-designed UI, implementing robust data management, and adding engaging features, you can develop an app that fosters a vibrant recipe-sharing community.
Further Reading:
Table of Contents
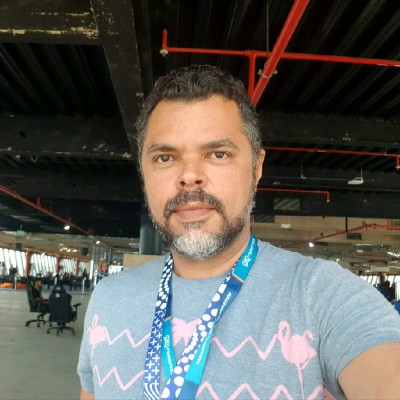
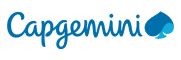