Innovative Web Development: Crafting Responsive Websites with Flutter
The world of web development has been evolving rapidly, and every day, new technologies and libraries are being introduced. Among them, one that has gained significant attention and popularity is Flutter. Traditionally known for its proficiency in mobile app development, Flutter has recently emerged as a powerful tool for web development, allowing developers to build stunning, highly responsive websites. This surge in popularity has led to an increasing demand to hire Flutter developers who can utilize its full potential.
In this blog post, we will delve into the world of Flutter for web development, exploring how it can be used to build responsive websites. This comprehensive guide will include examples to help you understand its concepts and applications better. By the end, not only will you gain a thorough understanding of building responsive websites using Flutter, but you’ll also learn why businesses are keen to hire Flutter developers for creating powerful web solutions.
What is Flutter?
Developed by Google, Flutter is an open-source UI toolkit that allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase. It offers a rich set of fully customizable widgets that you can use to develop native interfaces in minutes.
Flutter for Web Development
Although Flutter was initially designed for mobile, the release of Flutter 1.9 in 2019 brought experimental support for web development. With Flutter 2.0, which came out in 2021, Flutter for web moved from experimental to stable, making it an excellent choice for building full-featured, high-quality websites. This steady evolution of Flutter and its expanding capabilities have made it an attractive platform for businesses looking to hire Flutter developers.
The beauty of Flutter for web lies in its ability to offer a highly responsive layout, which can automatically adjust itself to various screen sizes and orientations. Thus, it significantly simplifies the development of responsive websites. As a result, more businesses are inclined to hire Flutter developers who can leverage these functionalities to create web applications that deliver a consistent and adaptive user experience across multiple devices.
Building Responsive Websites with Flutter
Building responsive websites with Flutter involves thinking about the layout and design of the website on different screen sizes. Luckily, Flutter provides several widgets and techniques to make this process easier.
Let’s break down the steps and methods to create a responsive website using Flutter.
1. MediaQuery:
`MediaQuery` is a widget that can be used to determine the physical size of the device screen. Here’s a simple example:
```dart var screenSize = MediaQuery.of(context).size; ```
In this example, `screenSize` would hold the width and height of the screen. You can use this data to conditionally render different layouts depending on the screen size.
2. LayoutBuilder:
`LayoutBuilder` is another handy widget that gives the maximum width and height that the parent widget can provide. Here’s how you can use it:
```dart LayoutBuilder( builder: (BuildContext context, BoxConstraints constraints) { if (constraints.maxWidth > 600) { return _buildWideContainers(); } else { return _buildNarrowContainers(); } }, ); ```
In this example, if the maximum width provided by the parent widget is more than 600 pixels, `_buildWideContainers()` is called. Otherwise, `_buildNarrowContainers()` is called.
3. Responsive Frameworks:
There are also third-party packages, like `responsive_framework`, that provide more comprehensive solutions for creating responsive websites in Flutter. Here’s a quick example of how to use it:
```dart ResponsiveWrapper.builder( ClampingScrollWrapper.builder(context, ListView.builder( itemCount: 100, itemBuilder: (context, index) => Text("Item $index"), )), maxWidth: 1200, minWidth: 450, defaultScale: true, breakpoints: [ ResponsiveBreakpoint.resize(450, name: MOBILE), ResponsiveBreakpoint.autoScale(800, name: TABLET), ResponsiveBreakpoint.autoScale(1000, name: TABLET), ResponsiveBreakpoint.resize(2460, name: "4K"), ], ); ```
In this example, `ResponsiveWrapper.builder` is used to create a responsive `ListView`. The list will adjust its layout based on the breakpoints defined.
Case Study: Building a Blog Website
Let’s assume we’re building a simple blog website. The website should have a sidebar on large screens, and a bottom navigation bar on small screens.
First, we’d need to set up the project using Flutter CLI:
```sh flutter create blog_website cd blog_website flutter run ```
After setting up the project, we can define the layout for our website:
```dart class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Blog Website', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(title: 'Flutter Blog Website'), ); } } class MyHomePage extends StatelessWidget { MyHomePage({Key key, this.title}) : super(key: key); final String title; @override Widget build(BuildContext context) { var screenSize = MediaQuery.of(context).size; return Scaffold( appBar: AppBar( title: Text(title), ), body: (screenSize.width < 600) ? Column( children: <Widget>[ Expanded(child: BlogPostList()), BottomNavigationBar(), ], ) : Row( children: <Widget>[ Sidebar(), Expanded(child: BlogPostList()), ], ), ); } } class BlogPostList extends StatelessWidget { @override Widget build(BuildContext context) { // This widget is the root of your application. return ListView.builder( itemCount: 10, itemBuilder: (context, index) => ListTile( title: Text('Blog Post $index'), ), ); } } ```
In the above example, `BlogPostList` is a widget that displays a list of blog posts. Depending on the width of the screen, it will either be displayed with a `BottomNavigationBar` below it or with a `Sidebar` beside it.
Conclusion
Flutter for web development provides a seamless, consistent, and efficient way of building high-quality, responsive websites. Its rich set of features and widgets, along with its single codebase philosophy, offers a promising and versatile tool for developers. The ease of building responsive designs is particularly noteworthy, with several tools and techniques at developers’ disposal, from MediaQuery to LayoutBuilder, and even third-party packages like `responsive_framework`. This wide array of capabilities has heightened the demand to hire Flutter developers who can adeptly navigate this platform.
By leveraging Flutter for web development, you can ensure a robust, visually appealing, and responsive web presence, adaptable across a range of devices, thereby enhancing your user experience manifold. Organizations looking to capitalize on these benefits often choose to hire Flutter developers, as they bring the expertise to efficiently use this platform and deliver optimal web solutions. The trend towards hiring Flutter developers is only set to rise, as more businesses recognize the potential Flutter holds for revolutionizing their web presence.
Table of Contents
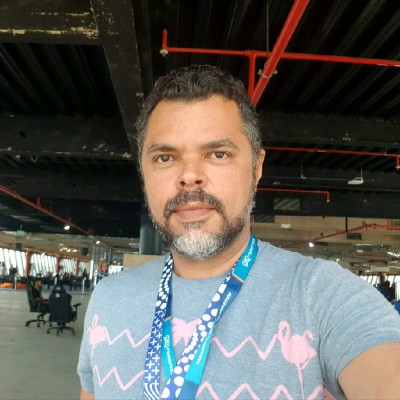
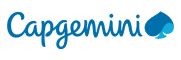