Building a Restaurant Finder App with Flutter: Maps and Reviews
Flutter is an open-source UI toolkit developed by Google that enables the creation of natively compiled applications for mobile, web, and desktop from a single codebase. This article will guide you through the process of building a restaurant finder app using Flutter, focusing on integrating interactive maps and user reviews.
Understanding the Restaurant Finder App
A restaurant finder app allows users to search for dining options, view restaurant details, and read reviews. By leveraging Flutter, you can create a sleek and responsive app that offers a seamless user experience.
Using Flutter for Building the App
Flutter provides a robust framework and a rich set of libraries to facilitate app development. Here’s how you can utilize Flutter for building a restaurant finder app with maps and reviews:
1. Integrating Maps into Your App
Maps are crucial for a restaurant finder app, allowing users to locate restaurants easily.
Example: Using the `google_maps_flutter` Plugin
- Add the Plugin to Your Project
Open your `pubspec.yaml` file and add the `google_maps_flutter` dependency:
```yaml dependencies: google_maps_flutter: ^2.1.1 ```
- Configure Google Maps API
Obtain an API key from the [Google Cloud Console](https://console.cloud.google.com/) and enable the Maps SDK for Android and iOS.
- Implement the Map Widget
Use the `GoogleMap` widget to display a map:
```dart GoogleMap( initialCameraPosition: CameraPosition( target: LatLng(37.7749, -122.4194), zoom: 14.0, ), ) ```
- Add Markers for Restaurants
Use markers to indicate restaurant locations:
```dart Marker( markerId: MarkerId('restaurant1'), position: LatLng(37.7749, -122.4194), infoWindow: InfoWindow(title: 'Restaurant Name'), ) ```
2. Implementing User Reviews
User reviews provide valuable feedback and help others make informed decisions.
Example: Using the `flutter_rating_bar` Plugin
- Add the Plugin to Your Project
Add `flutter_rating_bar` to your `pubspec.yaml` file:
```yaml dependencies: flutter_rating_bar: ^4.0.0 ```
- Display Ratings and Reviews
Use the `RatingBar` widget to show ratings:
```dart RatingBar.builder( initialRating: 3, minRating: 1, direction: Axis.horizontal, allowHalfRating: true, itemCount: 5, itemPadding: EdgeInsets.symmetric(horizontal: 4.0), itemBuilder: (context, _) => Icon( Icons.star, color: Colors.amber, ), onRatingUpdate: (rating) { print(rating); }, ) ```
- Integrate Review Submission
Implement a form for users to submit reviews:
```dart TextFormField( decoration: InputDecoration( labelText: 'Write a review', ), maxLines: 3, ) ```
3. Fetching and Displaying Data
To provide real-time data, integrate your app with a backend service or database.
Example: Using Firebase Firestore
- Add Firebase Dependencies
Add Firebase dependencies to your `pubspec.yaml` file:
```yaml dependencies: firebase_core: ^2.0.0 cloud_firestore: ^4.0.0 ```
- Configure Firebase
Follow the [Firebase documentation](https://firebase.google.com/docs/flutter/setup) to set up Firebase for your Flutter project.
- Fetch and Display Data
Fetch restaurant data from Firestore and display it in your app:
```dart StreamBuilder( stream: FirebaseFirestore.instance.collection('restaurants').snapshots(), builder: (context, snapshot) { if (!snapshot.hasData) return CircularProgressIndicator(); // Build your list view here }, ) ```
Conclusion
Building a restaurant finder app with Flutter involves integrating maps for location-based services, implementing user reviews for feedback, and fetching data from a backend. By following these steps, you can create an engaging and functional app that helps users discover dining options and make informed choices.
Further Reading:
Table of Contents
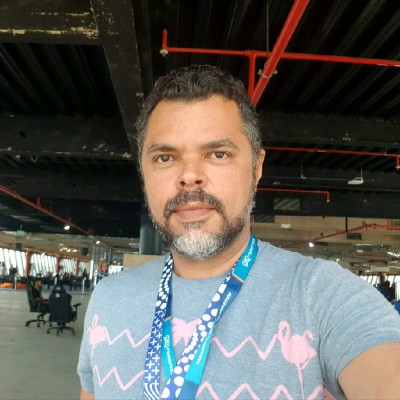
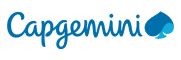