Securing Flutter Apps: Authentication and Authorization
Securing mobile applications is paramount in today’s digital landscape. Flutter, a popular framework for building natively compiled applications for mobile, web, and desktop from a single codebase, provides several tools and packages to implement robust security measures. This article explores how to secure Flutter apps through effective authentication and authorization strategies, ensuring that user data and application resources are well-protected.
Understanding Authentication and Authorization
Authentication and authorization are fundamental aspects of application security. Authentication verifies the identity of users, while authorization determines what resources or actions a user is permitted to access or perform.
– Authentication: The process of verifying who a user is.
– Authorization: The process of determining what a user can do.
Using Flutter for Authentication
Flutter offers various packages to implement authentication in your applications. Below are some key methods and code examples demonstrating how to set up authentication in Flutter apps.
1. Firebase Authentication
Firebase provides a comprehensive authentication service with support for email/password, phone authentication, and social login providers.
Example: Setting Up Email/Password Authentication with Firebase
First, add the necessary Firebase dependencies to your `pubspec.yaml`:
```yaml dependencies: firebase_core: latest_version firebase_auth: latest_version ```
Initialize Firebase in your app:
```dart import 'package:firebase_core/firebase_core.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); runApp(MyApp()); } ```
Implement email/password authentication:
```dart import 'package:firebase_auth/firebase_auth.dart'; import 'package:flutter/material.dart'; class SignInPage extends StatefulWidget { @override _SignInPageState createState() => _SignInPageState(); } class _SignInPageState extends State<SignInPage> { final _emailController = TextEditingController(); final _passwordController = TextEditingController(); final _auth = FirebaseAuth.instance; Future<void> _signIn() async { try { await _auth.signInWithEmailAndPassword( email: _emailController.text, password: _passwordController.text, ); // Navigate to the home screen } catch (e) { print('Error: $e'); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Sign In')), body: Column( children: [ TextField(controller: _emailController, decoration: InputDecoration(labelText: 'Email')), TextField(controller: _passwordController, obscureText: true, decoration: InputDecoration(labelText: 'Password')), ElevatedButton(onPressed: _signIn, child: Text('Sign In')), ], ), ); } } ```
2. OAuth2 Authentication
For OAuth2 authentication, consider using packages like `flutter_auth_buttons` or `google_sign_in` for Google authentication.
Example: Google Sign-In Integration
Add dependencies:
```yaml dependencies: google_sign_in: latest_version ```
Implement Google Sign-In:
```dart import 'package:google_sign_in/google_sign_in.dart'; import 'package:flutter/material.dart'; class GoogleSignInPage extends StatefulWidget { @override _GoogleSignInPageState createState() => _GoogleSignInPageState(); } class _GoogleSignInPageState extends State<GoogleSignInPage> { final GoogleSignIn _googleSignIn = GoogleSignIn(); Future<void> _signIn() async { try { final user = await _googleSignIn.signIn(); print('User signed in: ${user.displayName}'); // Navigate to the home screen } catch (e) { print('Error: $e'); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Google Sign-In')), body: Center( child: ElevatedButton(onPressed: _signIn, child: Text('Sign In with Google')), ), ); } } ```
Implementing Authorization in Flutter
Authorization ensures users have the right permissions for accessing specific resources. Below are strategies for implementing authorization:
1. Role-Based Access Control (RBAC)
Define user roles and permissions, and check these roles to manage access to different parts of your app.
Example: Role-Based Access Control
Assume user roles are fetched from Firebase or another backend:
```dart import 'package:flutter/material.dart'; class HomePage extends StatelessWidget { final String userRole; HomePage(this.userRole); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Home')), body: userRole == 'admin' ? AdminPanel() : UserPanel(), ); } } class AdminPanel extends StatelessWidget { @override Widget build(BuildContext context) { return Center(child: Text('Admin Dashboard')); } } class UserPanel extends StatelessWidget { @override Widget build(BuildContext context) { return Center(child: Text('User Dashboard')); } } ```
2. Feature-Based Access Control
Control access to specific features based on user roles or subscription levels.
Example: Feature Access Control
```dart class FeaturePage extends StatelessWidget { final bool hasPremiumAccess; FeaturePage(this.hasPremiumAccess); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Feature')), body: hasPremiumAccess ? PremiumFeature() : UpgradePrompt(), ); } } class PremiumFeature extends StatelessWidget { @override Widget build(BuildContext context) { return Center(child: Text('Premium Feature Access')); } } class UpgradePrompt extends StatelessWidget { @override Widget build(BuildContext context) { return Center(child: Text('Upgrade to Premium for Access')); } } ```
Conclusion
Securing Flutter apps through robust authentication and authorization strategies is essential for protecting user data and managing access to resources effectively. By utilizing Firebase Authentication, OAuth2, and implementing role-based or feature-based access controls, you can ensure a secure and reliable user experience.
Further Reading:
Table of Contents
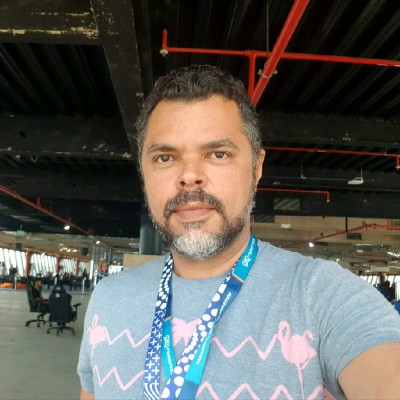
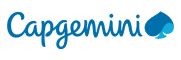