Flutter Q & A
What is the purpose of the SingleChildScrollView widget in Flutter?
The `SingleChildScrollView` widget in Flutter serves a crucial role in managing scrollable content within an application. Its primary purpose is to allow users to scroll through a child widget, providing a way to view content that exceeds the screen size. Here are key points and examples illustrating the significance of the `SingleChildScrollView` widget:
- Vertical Scrolling: The widget enables vertical scrolling, allowing users to navigate through content that extends beyond the visible area of the screen.
- Content Overflow: When the child widget’s content is too large to fit on the screen, the `SingleChildScrollView` allows smooth and efficient scrolling.
- Avoiding Render Overflow Errors: In scenarios where the content exceeds the screen space and isn’t accommodated properly, Flutter may trigger a render overflow error. The `SingleChildScrollView` resolves this issue by providing a scrolling capability.
- Flexible Content: It accommodates a variety of child widgets, making it suitable for scenarios where the content’s height is dynamic or varies based on user input or data.
Example:
```dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('SingleChildScrollView Example'), ), body: SingleChildScrollView( child: Column( children: List.generate( 20, (index) => Container( height: 100, width: double.infinity, color: index % 2 == 0 ? Colors.blue : Colors.green, child: Center( child: Text( 'Item $index', style: TextStyle(fontSize: 20, color: Colors.white), ), ), ), ), ), ), ), ); } } ```
In this example, a `SingleChildScrollView` wraps a `Column` containing dynamically generated `Container` widgets. As the number of items increases, the scrolling capability of `SingleChildScrollView` ensures that all items remain accessible, preventing any overflow issues on the screen.
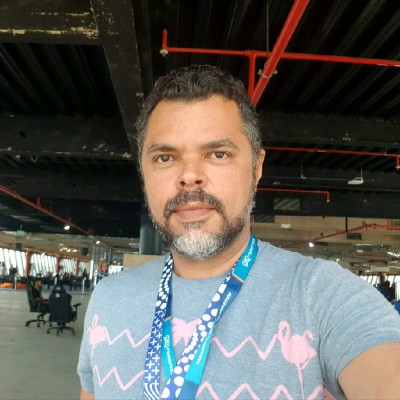
Previously at
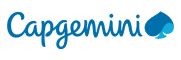
Full Stack Systems Analyst with a strong focus on Flutter development. Over 5 years of expertise in Flutter, creating mobile applications with a user-centric approach.