Building a Social Networking App with Flutter: Profiles and Friendships
Social networking apps are increasingly popular, offering users platforms to connect, share, and interact. Flutter, with its rich set of widgets and cross-platform capabilities, is an excellent framework for building engaging social networking applications. This blog explores how Flutter can be used to develop essential features like user profiles and friendships, providing practical examples and insights.
Understanding Social Networking Features
A social networking app typically includes features such as user profiles, friend connections, and interaction capabilities. Implementing these features requires careful design and efficient coding practices to ensure a smooth user experience.
Using Flutter for Social Networking Features
Flutter offers a fast development cycle and a rich set of pre-designed widgets that can be customized for building social networking apps. Below are some key aspects and code examples demonstrating how Flutter can be utilized for profiles and friendships.
1. Building User Profiles
User profiles are central to social networking apps. In Flutter, you can create a profile screen using widgets like `ListView`, `Container`, and `Column` to display user information and interaction options.
Example: Creating a User Profile Screen
Here’s how you might build a simple profile screen displaying user details and a list of their friends.
```dart import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: ProfileScreen(), ); } } class ProfileScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('User Profile')), body: Column( children: <Widget>[ Container( padding: EdgeInsets.all(16.0), child: Row( children: <Widget>[ CircleAvatar(radius: 40, backgroundImage: AssetImage('assets/profile.jpg')), SizedBox(width: 16), Column( crossAxisAlignment: CrossAxisAlignment.start, children: <Widget>[ Text('John Doe', style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold)), Text('Software Engineer', style: TextStyle(fontSize: 16, color: Colors.grey)), ], ), ], ), ), Expanded(child: FriendList()), ], ), ); } } class FriendList extends StatelessWidget { @override Widget build(BuildContext context) { // Example friend list final friends = ['Alice', 'Bob', 'Charlie']; return ListView.builder( itemCount: friends.length, itemBuilder: (context, index) { return ListTile(title: Text(friends[index])); }, ); } } ```
2. Managing Friendships
Friendship management involves functionalities like sending friend requests, accepting or rejecting requests, and viewing a list of friends. In Flutter, you can use state management solutions like `Provider` or `Riverpod` to handle the dynamic nature of friendship interactions.
Example: Implementing Friend Request Functionality
Here’s how you might create a simple UI for sending and managing friend requests.
```dart import 'package:flutter/material.dart'; import 'package:provider/provider.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return ChangeNotifierProvider( create: (_) => FriendRequestManager(), child: MaterialApp( home: FriendRequestScreen(), ), ); } } class FriendRequestManager extends ChangeNotifier { List<String> _requests = []; List<String> get requests => _requests; void addRequest(String user) { _requests.add(user); notifyListeners(); } void removeRequest(String user) { _requests.remove(user); notifyListeners(); } } class FriendRequestScreen extends StatelessWidget { @override Widget build(BuildContext context) { final requestManager = Provider.of<FriendRequestManager>(context); return Scaffold( appBar: AppBar(title: Text('Friend Requests')), body: Column( children: <Widget>[ Expanded( child: ListView.builder( itemCount: requestManager.requests.length, itemBuilder: (context, index) { final user = requestManager.requests[index]; return ListTile( title: Text(user), trailing: IconButton( icon: Icon(Icons.check), onPressed: () { requestManager.removeRequest(user); }, ), ); }, ), ), RaisedButton( onPressed: () { // Simulate adding a friend request requestManager.addRequest('New User'); }, child: Text('Add Friend Request'), ), ], ), ); } } ```
3. Creating a Friendship Network
Visualizing a network of friends can help users understand their connections better. In Flutter, you can use packages like `graphview` to create and display graphical representations of friend networks.
Example: Visualizing Friendships with GraphView
Here’s an example of how you might use `graphview` to visualize a simple friendship network.
```dart import 'package:flutter/material.dart'; import 'package:graphview/GraphView.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: NetworkScreen(), ); } } class NetworkScreen extends StatelessWidget { @override Widget build(BuildContext context) { final graph = Graph(); final nodeA = Node.Id('Alice'); final nodeB = Node.Id('Bob'); final nodeC = Node.Id('Charlie'); graph.addEdge(nodeA, nodeB); graph.addEdge(nodeB, nodeC); return Scaffold( appBar: AppBar(title: Text('Friendship Network')), body: Center( child: GraphView( graph: graph, algorithm: BuchheimWalkerAlgorithm( BuchheimWalkerConfiguration() ..nodeAlign = NodeAlign.top ..siblingSeparation = (10) ..levelSeparation = (50), ), builder: (Node node) { return RectangleAvatar(node.id); }, ), ), ); } } class RectangleAvatar extends StatelessWidget { final String id; RectangleAvatar(this.id); @override Widget build(BuildContext context) { return Container( padding: EdgeInsets.all(8.0), decoration: BoxDecoration( color: Colors.blue, borderRadius: BorderRadius.circular(8.0), ), child: Text(id, style: TextStyle(color: Colors.white)), ); } } ```
4. Integrating with Backend Services
Integrating your app with backend services allows for dynamic user interactions and data management. You can use packages like `http` or `dio` to handle network requests and sync data with a backend server.
Example: Fetching User Data from a Backend
```dart import 'package:flutter/material.dart'; import 'package:http/http.dart' as http; import 'dart:convert'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: UserListScreen(), ); } } class UserListScreen extends StatefulWidget { @override _UserListScreenState createState() => _UserListScreenState(); } class _UserListScreenState extends State<UserListScreen> { Future<List<String>> fetchUsers() async { final response = await http.get('https://api.example.com/users'); final data = json.decode(response.body) as List; return data.map((user) => user['name'].toString()).toList(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('User List')), body: FutureBuilder<List<String>>( future: fetchUsers(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) { return Center(child: CircularProgressIndicator()); } else if (snapshot.hasError) { return Center(child: Text('Error: ${snapshot.error}')); } else if (!snapshot.hasData || snapshot.data!.isEmpty) { return Center(child: Text('No users found.')); } else { return ListView( children: snapshot.data!.map((user) => ListTile(title: Text(user))).toList(), ); } }, ), ); } } ```
Conclusion
Flutter provides a powerful and flexible framework for building social networking apps, offering rich features and capabilities to handle user profiles, friendships, and interactions. By leveraging Flutter’s widgets, state management solutions, and integration capabilities, you can create engaging and functional social networking applications that enhance user connectivity and interaction.
Further Reading:
Table of Contents
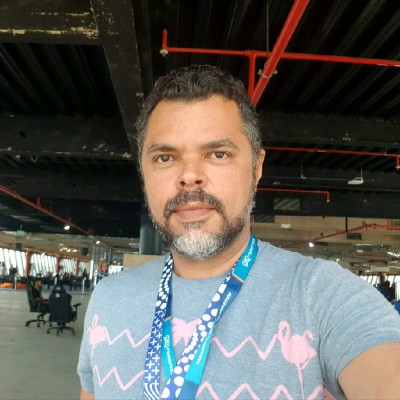
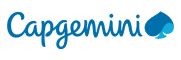