How do you implement a splash screen in Flutter?
Implementing a splash screen in Flutter involves creating a visually appealing introductory screen that appears while the application initializes. Follow these key points and examples to achieve an effective splash screen:
- Create a Splash Screen Widget:
– Begin by designing a dedicated Splash Screen widget. This widget typically includes the app’s logo, branding, or any captivating animation.
```dart class SplashScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Image.asset('assets/logo.png'), // Example image asset ), ); } } ```
- Set the Splash Screen Duration:
– Determine the duration you want the splash screen to be visible. This duration can vary based on the complexity of your app initialization.
```dart void main() { runApp(MyApp()); } ```
- Navigate to the Main Screen:
– After the splash screen duration, navigate to the main screen or the home screen of your application. Use `Navigator` for seamless transitions.
```dart Future<void> main() async { WidgetsFlutterBinding.ensureInitialized(); await Future.delayed(Duration(seconds: 2)); // Example duration runApp(MyApp()); } ```
- Optimize for Various Screen Sizes:
– Ensure your splash screen looks appealing on various screen sizes and orientations by using responsive design principles.
- Add Animation:
– To enhance user experience, consider adding entrance animations to elements on the splash screen. Flutter provides animation widgets like `Hero` or you can use packages like `flare_flutter` for more complex animations.
- Preload Resources:
– If your app requires resources like images or data, preload them during the splash screen to expedite the main screen’s loading time.
- Customize Splash Screen Appearance:
– Tailor the splash screen’s appearance to align with your app’s branding and design guidelines. This includes choosing the right colors, fonts, and layout.
By following these steps, you’ll create a polished and efficient splash screen for your Flutter app, providing users with a visually pleasing introduction to your application.
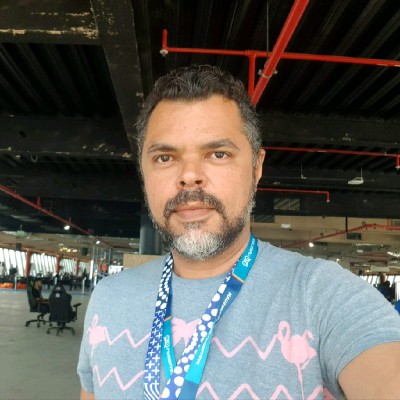
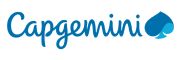