Choosing the Right State Management Approach for Your Flutter Application
In any application development project, state management is a critical component that determines how the app responds to user interactions. When it comes to building apps using Flutter, a popular cross-platform app development framework, state management is key. Despite its importance, state management in Flutter is often a topic that confuses developers, including those looking to hire Flutter developers, primarily due to the numerous options available.
This blog post will delve into the different state management approaches in Flutter, aiming to clarify this topic for both experienced developers and those considering to hire Flutter developers. By explaining the principles, pros, and cons of each method, we hope to provide a comprehensive guide to help you navigate this critical aspect of Flutter app development.
Understanding State Management
In application development, “state” refers to the information that can change over time and affect the behavior and output of an application. Managing the state involves handling user interactions, managing data, and updating the UI accordingly. Effective state management enables smooth user experiences, more predictable code, and easier debugging.
State Management Approaches in Flutter
Flutter offers several approaches to state management. Each one has its strengths, weaknesses, and specific use cases where they excel. Some of the commonly used methods include:
- Provider
- Riverpod
- Redux
- Bloc
- MobX
Let’s explore each one in detail:
1. Provider
Provider is a wrapper around the InheritedWidget, making it easier to manage and update state across widgets. The package, endorsed by the Flutter team, serves as a good starting point for state management.
1.1 Principles
Provider is all about dependency injection. It can provide values directly (Provider), expose a single value that might change over time (ChangeNotifierProvider), or expose a complex family of objects (StreamProvider, FutureProvider).
1.2 Pros
– Easy to understand, especially for beginners.
– Reduces boilerplate over using InheritedWidgets directly.
– Does not require additional classes or methods for small projects.
1.3 Cons
– It may not scale well for larger projects with complex states.
– The use of ChangeNotifier can lead to unnecessary widget rebuilds if not used carefully.
1.4 Example
The following code snippet demonstrates the basic usage of Provider for state management:
```dart void main() { runApp( ChangeNotifierProvider( create: (context) => Counter(), child: MyApp(), ), ); } class Counter with ChangeNotifier { int value = 0; void increment() { value += 1; notifyListeners(); } } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Provider Example')), body: Center( child: Text( 'Value: ${context.watch<Counter>().value}', ), ), floatingActionButton: FloatingActionButton( onPressed: () => context.read<Counter>().increment(), child: Icon(Icons.add), ), ), ); } } ```
In this example, we create a simple counter app where we encapsulate our state (the counter value) in a `ChangeNotifier` and use `context.watch` and `context.read` to read the value and trigger state changes.
2. Riverpod
Riverpod is a relatively newer package that was developed by the same author as Provider. It aims to address some limitations of Provider while retaining its simplicity and power.
2.1 Principles
Like Provider, Riverpod is all about dependency injection. However, Riverpod providers are immutable and globally accessible, which provides several benefits over Provider.
2.2 Pros
– It’s safer and more flexible than Provider.
– It allows for easier testing and mocking.
– It doesn’t require context to read a provider.
2.3 Cons
– Being relatively new, it might have fewer community resources.
– It may be overwhelming for beginners due to its different design principles.
2.4 Example
Here’s how the previous counter example would look like with Riverpod:
```dart final counterProvider = StateProvider<int>((ref) => 0); void main() { runApp(ProviderScope(child: MyApp())); } class MyApp extends ConsumerWidget { @override Widget build(BuildContext context, ScopedReader watch) { final counter = watch(counterProvider).state; return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Riverpod Example')), body: Center( child: Text('Value: $counter'), ), floatingActionButton: FloatingActionButton( onPressed: () => context.read(counterProvider).state++, child: Icon(Icons.add), ), ), ); } } ```
In this example, we define our state as a `StateProvider` at the top level of our app, and we read and modify this state in our widgets using `watch` and `read`.
3. Redux
Redux is a predictable state container designed to help you write JavaScript apps that behave consistently across client, server, and native environments.
3.1 Principles
Redux implements the Flux architecture and emphasizes a unidirectional data flow, immutability, and predictability.
3.2 Pros
– Enables easy debugging and time-travel debugging.
– Good for larger projects where predictability and consistency are required.
– Well-established with extensive community resources.
3.3 Cons
– Steeper learning curve.
– Requires more boilerplate code.
3.4 Example
Here is a simplified version of the counter app implemented with Redux in Flutter:
```dart class AppState { final int counter; AppState(this.counter); } enum Actions { Increment } AppState reducer(AppState prevState, dynamic action) { if (action == Actions.Increment) { return AppState(prevState.counter + 1); } return prevState; } void main() { final store = Store<AppState>(reducer, initialState: AppState(0)); runApp(MyApp(store)); } class MyApp extends StatelessWidget { final Store<AppState> store; MyApp(this.store); @override Widget build(BuildContext context) { return StoreProvider<AppState>( store: store, child: MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Redux Example')), body: Center( child: StoreConnector<AppState, String>( converter: (store) => store.state.counter.toString(), builder: (context, counter) => Text('Value: $counter'), ), ), floatingActionButton: FloatingActionButton( onPressed: () => store.dispatch(Actions.Increment), child: Icon(Icons.add), ), ), ), ); } } ```
In the Redux example, we have an `AppState` to hold our state, a `reducer` to define how state changes in response to actions, and a `Store` to hold our app state and dispatch actions.
4. Bloc
Bloc (Business Logic Component) is a well-liked and widely used state management library in the Flutter ecosystem.
4.1 Principles
Bloc is built on top of RxDart and streams, emphasizing the separation of presentation from business logic.
4.2 Pros
– Enforces a clean architecture and separation of concerns.
– Has clear and well-defined conventions.
– Extensive community resources and support.
4.3 Cons
– Steeper learning curve due to its reliance on streams.
– Can be overkill for small, simple apps.
4.4 Example
Here is a Bloc version of the counter app:
```dart enum CounterEvent { increment } class CounterBloc { int _counter = 0; final _counterStateController = StreamController<int>(); StreamSink<int> get _inCounter => _counterStateController.sink; Stream<int> get counter => _counterStateController.stream; final _counterEventController = StreamController<CounterEvent>(); Sink<CounterEvent> get counterEventSink => _counterEventController.sink; CounterBloc() { _counterEventController.stream.listen(_mapEventToState); } void _mapEventToState(CounterEvent event) { if (event == CounterEvent.increment) _counter++; _inCounter.add(_counter); } void dispose() { _counterStateController.close(); _counterEventController.close(); } } void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { final bloc = CounterBloc(); @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Bloc Example')), body: Center( child: StreamBuilder<int>( stream: bloc.counter, initialData: 0, builder: (BuildContext context, AsyncSnapshot<int> snapshot) { return Text('Value: ${snapshot.data}'); }, ), ), floatingActionButton: FloatingActionButton( onPressed: () => bloc.counterEventSink.add(CounterEvent.increment), child: Icon(Icons.add), ), ), ); } } ```
In this Bloc example, the `CounterBloc` separates the business logic (incrementing the counter) from the presentation layer (the widgets). This allows for clean and maintainable code.
5. MobX
MobX is a state management solution that promotes a reactive programming coding style.
5.1 Principles
MobX revolves around observables, actions, and computed values, enabling you to manage and automatically track state changes in an efficient way.
5.2 Pros
– Offers a high level of abstraction and simplicity.
– Automatically manages dependencies between state variables.
– Allows for mutable state, which can be more intuitive for some developers.
5.3 Cons
– Steeper learning curve due to reactive programming concepts.
– Requires code generation, which can complicate the setup.
5.4 Example
Here’s how you might implement the counter app using MobX:
```dart class Counter = CounterBase with _$Counter; abstract class CounterBase with Store { @observable int value = 0; @action void increment() { value++; } } void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { final counter = Counter(); @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('MobX Example')), body: Center( child: Observer( builder: (_) => Text('Value: ${counter.value}'), ), ), floatingActionButton: FloatingActionButton( onPressed: counter.increment, child: Icon(Icons.add), ), ), ); } } ```
In this MobX example, we have an observable `value` and an action `increment` in our `Counter` store. We use an `Observer` widget to automatically rebuild our widgets when the state changes.
Choosing the Right Approach
Choosing the right state management approach for your Flutter application depends on several factors, including project size, team experience, and specific project requirements.
- Project Size: For small projects or prototyping, Provider or Riverpod are often sufficient. For larger, more complex projects, a more structured approach like Redux, Bloc, or MobX might be more suitable.
- Team Experience: The team’s familiarity with various programming paradigms (e.g., reactive programming, immutability) can also influence the choice. While learning new concepts can be beneficial, it might slow down the development process.
- Project Requirements: Some projects may have unique requirements that favor one approach over others. For example, if you require time-travel debugging, Redux would be a good choice. If you prefer mutable state and automatic dependency tracking, MobX might be the best fit.
Remember, the ultimate goal is to write maintainable and bug-free code. The “best” state management solution is the one that helps your team achieve this goal effectively and efficiently.
Conclusion
State management is a crucial part of any Flutter application, a concept well-understood by professional Flutter developers. Each state management solution has its advantages, disadvantages, and unique use cases. Understanding these can assist you in selecting the right approach for your next Flutter project, or even guide your decision when looking to hire Flutter developers. We hope this post has offered valuable insights to navigate these choices. Happy coding!
Table of Contents
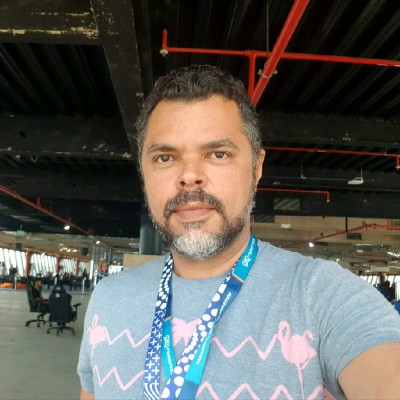
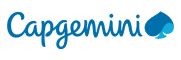