Stateful vs. Stateless Widgets in Flutter: When to Use Each
Flutter, the open-source UI framework from Google, has gained immense popularity for building beautiful and performant mobile applications. A crucial aspect of creating Flutter apps is understanding the concept of widgets. Widgets are the building blocks of Flutter applications, and they come in two main flavors: stateful and stateless. In this blog, we will dive deep into the differences between stateful and stateless widgets and explore when to use each for your Flutter projects.
Table of Contents
1. Understanding Widgets in Flutter
Before we get into the specifics of stateful and stateless widgets, let’s first understand what widgets are in Flutter.
1.1. What Are Widgets?
In Flutter, everything is a widget. Widgets are responsible for creating the user interface (UI) of your app. They can range from simple elements like buttons and text to complex layouts and even entire screens. Widgets can be combined to create more complex widgets, and this composability is one of the strengths of Flutter.
Widgets are categorized into two main types:
- Stateful Widgets: These widgets can change their state during their lifetime. Stateful widgets are mutable, meaning they can be updated, leading to changes in their appearance or behavior.
- Stateless Widgets: These widgets, as the name suggests, are immutable and do not change their state once created. They are purely based on their input parameters and always produce the same output for the same input.
Now, let’s explore each type in more detail.
2. Stateless Widgets
Stateless widgets are the simpler of the two types. They are used for UI components that do not change over time, meaning their properties remain constant after creation. Stateless widgets are perfect for displaying static content or elements that don’t require interaction or updates based on user input.
2.1. Characteristics of Stateless Widgets
- Immutable: Once created, the properties of a stateless widget cannot be changed.
- Rebuild on Change: Stateless widgets are rebuilt only when the parent widget or the data they depend on changes.
- Purely Functional: Stateless widgets are based solely on their input parameters and produce the same output for the same input.
2.2. Use Cases for Stateless Widgets
- Displaying Static Content: Use stateless widgets for elements like labels, icons, or images that do not change based on user interactions.
- Reusable Components: Stateless widgets are great for creating reusable UI components that can be used across your app.
- Performance Benefits: Stateless widgets are more performant because they don’t need to manage state. They are an excellent choice for parts of your UI that won’t change often.
Here’s an example of a simple stateless widget that displays a static piece of text:
dart class MyStaticWidget extends StatelessWidget { final String text; MyStaticWidget(this.text); @override Widget build(BuildContext context) { return Text(text); } }
In this example, MyStaticWidget takes a text parameter and displays it using the Text widget. Since this widget doesn’t change its state or appearance, it’s a suitable use case for a stateless widget.
3. Stateful Widgets
Stateful widgets, on the other hand, can change their internal state during their lifetime. They are used for UI components that need to update based on user interactions, data changes, or any other dynamic factors. Stateful widgets are more versatile and can handle complex UI scenarios.
3.1. Characteristics of Stateful Widgets
- Mutable: Stateful widgets can change their internal state, which can trigger rebuilds of the widget.
- Dynamic Behavior: They are ideal for UI elements that need to respond to user input or data changes.
- Lifespan Management: Stateful widgets have a longer lifespan as compared to stateless widgets, allowing them to persist and manage data.
3.2. Use Cases for Stateful Widgets
- User Input Handling: Use stateful widgets for UI elements that require user interaction, such as buttons, forms, and sliders.
- Dynamic Data Display: When you need to display data that can change over time, like real-time updates or user-specific information, stateful widgets are a better choice.
- Complex UI Components: Stateful widgets are suitable for building complex UI components that involve multiple interactions and animations.
Here’s an example of a stateful widget that represents a simple counter button:
dart class CounterWidget extends StatefulWidget { @override _CounterWidgetState createState() => _CounterWidgetState(); } class _CounterWidgetState extends State<CounterWidget> { int _counter = 0; void _incrementCounter() { setState(() { _counter++; }); } @override Widget build(BuildContext context) { return Column( children: [ Text('Counter Value: $_counter'), ElevatedButton( onPressed: _incrementCounter, child: Text('Increment Counter'), ), ], ); } }
In this example, _CounterWidgetState maintains the state of the counter, and the _incrementCounter function updates the state when the button is pressed. This is a classic use case for a stateful widget as it manages and updates its internal state based on user interaction.
4. When to Choose Stateless Widgets
Now that we have a clear understanding of stateless and stateful widgets, let’s explore when it’s best to use stateless widgets in your Flutter app development.
4.1. Static Content
As mentioned earlier, stateless widgets are perfect for displaying static content that doesn’t change. This includes elements like labels, icons, and images.
dart class MyStaticWidget extends StatelessWidget { final String text; MyStaticWidget(this.text); @override Widget build(BuildContext context) { return Text(text); } }
In this example, MyStaticWidget displays a static piece of text and is an ideal use case for a stateless widget.
4.2. Reusable Components
Stateless widgets are highly reusable. You can create custom UI components as stateless widgets and use them throughout your app. This promotes a clean and maintainable codebase.
dart class MyButton extends StatelessWidget { final String label; final VoidCallback onPressed; MyButton({required this.label, required this.onPressed}); @override Widget build(BuildContext context) { return ElevatedButton( onPressed: onPressed, child: Text(label), ); } }
In this example, MyButton is a reusable button component that can be used across your app whenever you need a button with custom text and behavior.
4.3. Static Screens
For screens or sections of your app that display static information and do not require user interaction or dynamic updates, consider using stateless widgets. This helps keep your code simple and efficient.
dart class AboutUsScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('About Us'), ), body: Center( child: Text('Welcome to our app!'), ), ); } }
The AboutUsScreen in this example is a static screen that doesn’t change, making it a suitable candidate for a stateless widget.
5. When to Choose Stateful Widgets
Stateful widgets are more versatile and come into play when you need to manage dynamic data, user interactions, or complex UI behavior. Let’s explore scenarios where stateful widgets are the better choice.
5.1. User Input Handling
When your UI elements require user interaction, such as handling button clicks, form submissions, or slider adjustments, use stateful widgets. They allow you to manage and respond to user input effectively.
dart class LoginForm extends StatefulWidget { @override _LoginFormState createState() => _LoginFormState(); } class _LoginFormState extends State<LoginForm> { final TextEditingController _emailController = TextEditingController(); final TextEditingController _passwordController = TextEditingController(); void _onSubmit() { // Handle form submission } @override Widget build(BuildContext context) { return Column( children: [ TextField( controller: _emailController, decoration: InputDecoration(labelText: 'Email'), ), TextField( controller: _passwordController, decoration: InputDecoration(labelText: 'Password'), obscureText: true, ), ElevatedButton( onPressed: _onSubmit, child: Text('Login'), ), ], ); } }
In this example, LoginForm is a stateful widget that manages user input fields and handles form submission.
5.2. Dynamic Data Display
When you need to display data that can change over time, such as real-time updates, user-specific information, or dynamic content from an API, stateful widgets are a better fit. They allow you to update the UI when data changes.
dart class DynamicDataWidget extends StatefulWidget { @override _DynamicDataWidgetState createState() => _DynamicDataWidgetState(); } class _DynamicDataWidgetState extends State<DynamicDataWidget> { String _data = 'Initial Data'; void _updateData() { setState(() { _data = 'Updated Data'; }); } @override Widget build(BuildContext context) { return Column( children: [ Text('Data: $_data'), ElevatedButton( onPressed: _updateData, child: Text('Update Data'), ), ], ); } }
In this example, DynamicDataWidget displays data that can be updated by pressing the “Update Data” button. Stateful widgets allow you to manage and reflect such dynamic changes in your UI.
5.3. Complex UI Components
For building complex UI components that involve multiple interactions, animations, or state management, stateful widgets provide the necessary flexibility and control.
dart class InteractiveCarousel extends StatefulWidget { @override _InteractiveCarouselState createState() => _InteractiveCarouselState(); } class _InteractiveCarouselState extends State<InteractiveCarousel> { int _currentIndex = 0; void _nextSlide() { setState(() { _currentIndex = (_currentIndex + 1) % 3; }); } @override Widget build(BuildContext context) { return Column( children: [ CarouselItem(imageUrl: 'image1.jpg'), CarouselItem(imageUrl: 'image2.jpg'), CarouselItem(imageUrl: 'image3.jpg'), ElevatedButton( onPressed: _nextSlide, child: Text('Next Slide'), ), ], ); } }
In this example, InteractiveCarousel manages a carousel of images with a “Next Slide” button. Stateful widgets are essential for handling the carousel’s state and transitioning between images.
6. Best Practices for Using Widgets
Whether you choose stateful or stateless widgets, there are some best practices to keep in mind for effective Flutter app development:
6.1. Keep Widgets Small and Focused
Each widget should have a single responsibility and represent a small piece of your UI. This makes your code more maintainable and encourages reusability.
6.2. Minimize Widget Rebuilds
To optimize performance, minimize the number of times widgets are rebuilt. Use the const constructor for stateless widgets and update stateful widgets only when necessary using the setState method.
6.3. Use Keys Wisely
Keys help Flutter identify widgets uniquely and can be essential in managing stateful widgets. However, use them judiciously, as they can lead to unexpected behavior if not used correctly.
6.4. Embrace Flutter’s Reactive Programming Model
Flutter provides a reactive programming model that allows you to efficiently manage and update your UI in response to changes. Utilize this model to keep your UI in sync with your data.
6.5. Test Your Widgets
Write tests for your widgets to ensure they behave as expected. Flutter provides excellent testing tools and libraries for this purpose.
Conclusion
In Flutter, choosing between stateful and stateless widgets depends on your app’s requirements and the specific UI elements you’re building. Stateless widgets are ideal for static content and reusable components, while stateful widgets shine when dealing with user interactions, dynamic data, and complex UI behavior.
To build efficient and performant Flutter apps, it’s crucial to understand the characteristics and use cases of both types of widgets. By making informed choices and following best practices, you can create engaging and responsive user interfaces that meet your users’ expectations.
As you continue your Flutter journey, experiment with both stateful and stateless widgets to get a feel for when to use each based on your project’s needs. Ultimately, mastering the art of widget selection will lead to more enjoyable and productive Flutter development experiences. Happy coding!
Table of Contents
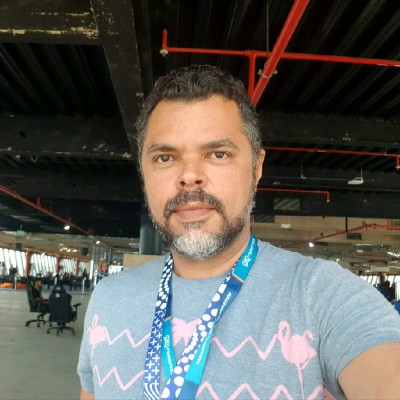
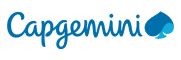