Building a Travel Booking App with Flutter: Payments and Reservations
Flutter is an excellent framework for developing cross-platform apps with a single codebase. When creating a travel booking app, two critical components are payments and reservations. This blog explores how to leverage Flutter for these essential features, offering practical examples and tips for successful implementation.
Understanding Travel Booking Apps
Travel booking apps typically involve features such as searching for flights, hotels, and car rentals, making reservations, and processing payments. A well-designed app should offer a seamless user experience and efficient management of booking and payment processes.
Using Flutter for Payment Integration
Flutter provides various packages and libraries to integrate payment gateways into your app. Popular choices include `flutter_stripe`, `paypal_flutter`, and `flutter_paypal`. These packages help facilitate secure payment transactions within your app.
Example: Integrating Stripe Payments
To integrate Stripe with your Flutter app, follow these steps:
1. Add Dependencies:
Add `flutter_stripe` to your `pubspec.yaml` file:
```yaml dependencies: flutter: sdk: flutter flutter_stripe: ^5.0.0 ```
2. Set Up Stripe:
Configure Stripe in your Flutter app by initializing it with your Stripe publishable key.
```dart import 'package:flutter_stripe/flutter_stripe.dart'; void main() { Stripe.publishableKey = 'your-publishable-key'; runApp(MyApp()); } ```
3. Create Payment Sheet:
Implement a payment sheet for handling user payments.
```dart import 'package:flutter_stripe/flutter_stripe.dart'; void _pay() async { try { // Create payment intent on your server final paymentIntent = await createPaymentIntent(); // Present the payment sheet await Stripe.instance.presentPaymentSheet( paymentSheetParameters: PaymentSheetParameters( paymentIntentClientSecret: paymentIntent.clientSecret, // Additional parameters ), ); print('Payment successful'); } catch (e) { print('Payment failed: $e'); } } ```
4. Handle Payment Confirmation:
Confirm the payment status and handle any post-payment actions.
```dart Future<PaymentIntent> createPaymentIntent() async { // Call your server to create a payment intent } ```
Managing Reservations
Reservations involve booking flights, hotels, or car rentals. You need to manage user data, availability, and booking confirmations. Flutter’s local storage and network libraries can assist with these tasks.
Example: Handling Reservations with Local Storage
1. Add Dependencies:
Add `sqflite` for local storage to your `pubspec.yaml` file:
```yaml dependencies: sqflite: ^2.0.0+4 path: ^1.8.0 ```
2. Set Up Database:
Initialize the local database to store reservation data.
```dart import 'package:sqflite/sqflite.dart'; import 'package:path/path.dart'; Future<Database> initializeDatabase() async { return openDatabase( join(await getDatabasesPath(), 'reservations.db'), onCreate: (db, version) { return db.execute( "CREATE TABLE reservations(id INTEGER PRIMARY KEY, name TEXT, date TEXT)", ); }, version: 1, ); } ```
3. Add Reservation Data:
Implement functions to insert and retrieve reservation data.
```dart Future<void> insertReservation(Reservation reservation) async { final Database db = await initializeDatabase(); await db.insert( 'reservations', reservation.toMap(), conflictAlgorithm: ConflictAlgorithm.replace, ); } Future<List<Reservation>> reservations() async { final Database db = await initializeDatabase(); final List<Map<String, dynamic>> maps = await db.query('reservations'); return List.generate(maps.length, (i) { return Reservation( id: maps[i]['id'], name: maps[i]['name'], date: maps[i]['date'], ); }); } ```
4. Create Reservation Model:
Define the model to represent reservation data.
```dart class Reservation { final int id; final String name; final String date; Reservation({required this.id, required this.name, required this.date}); Map<String, dynamic> toMap() { return { 'id': id, 'name': name, 'date': date, }; } } ```
Visualizing Booking Data
To enhance the user experience, consider visualizing booking data using charts or graphs. Flutter supports various packages like `fl_chart` or `charts_flutter` for this purpose.
Example: Displaying Booking Trends with `fl_chart`
1. Add Dependency:
Add `fl_chart` to your `pubspec.yaml` file:
```yaml dependencies: fl_chart: ^0.40.0 ```
2. Create Chart:
Use `fl_chart` to visualize booking data.
```dart import 'package:fl_chart/fl_chart.dart'; class BookingChart extends StatelessWidget { @override Widget build(BuildContext context) { return LineChart( LineChartData( lineBarsData: [ LineChartBarData( spots: [ FlSpot(0, 3), FlSpot(1, 7), FlSpot(2, 5), ], isCurved: true, colors: [Colors.blue], dotData: FlDotData(show: false), belowBarData: BarAreaData(show: false), ), ], titlesData: FlTitlesData(show: true), ), ); } } ```
Integrating External APIs for Booking
For real-time availability and booking, integrate with external APIs. Flutter’s `http` package is useful for making API requests.
Example: Fetching Data from a Booking API
```dart import 'package:http/http.dart' as http; Future<void> fetchBookingData() async { final response = await http.get(Uri.parse('https://api.booking.com/available-hotels')); if (response.statusCode == 200) { // Parse the response body print('Data fetched successfully'); } else { print('Failed to load data'); } } ```
Conclusion
Building a travel booking app with Flutter involves integrating payment systems and managing reservations efficiently. By leveraging Flutter’s robust libraries and tools, you can create a seamless user experience and handle booking data effectively. With the examples and tips provided, you can start developing a feature-rich travel booking app that meets modern user expectations.
Further Reading:
Table of Contents
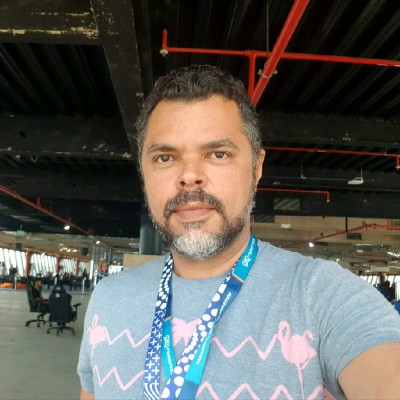
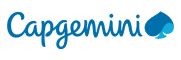