A Developer’s Guide to Stunning UI Design with Flutter
When it comes to creating stunning, interactive, and high-performing mobile applications, Flutter has quickly become one of the go-to frameworks. Many businesses are looking to hire Flutter developers due to the framework’s promising benefits. Released by Google in 2017, it’s earned praise for its robust widget library, seamless cross-platform capabilities, and strong performance.
The user interface (UI) is a vital part of any mobile app. With Flutter, designing the UI becomes an enjoyable task rather than a chore. Whether you’re a Flutter developer or a business planning to hire Flutter developers, understanding the framework’s potential for UI design is crucial. This blog post will guide you through some essential design tips and tricks to create beautiful UIs with Flutter.
1. Leverage the Power of Flutter’s Widgets
One of Flutter’s defining features is its rich, extensible widget system. Widgets are the building blocks of your app’s UI. They cover a broad spectrum – from simple layout structures like Padding or Center to complex interface elements like MaterialApp or Scaffold.
For instance, consider a scenario where you need a beautiful, custom alert dialog. Instead of manually writing the entire code for it, you can use Flutter’s AlertDialog widget:
```dart showDialog( context: context, builder: (BuildContext context) { return AlertDialog( title: Text('Alert Dialog'), content: Text('This is a custom alert dialog in Flutter.'), actions: <Widget>[ TextButton( child: Text('Close'), onPressed: () { Navigator.of(context).pop(); }, ), ], ); }, ); ```
This is just a basic example, but it shows how using widgets can significantly simplify your code and improve UI design efficiency.
2. Use Custom Themes for Consistent UI
Themes in Flutter allow you to maintain consistency across your app. It means you can define your color schemes, typographies, and button styles in one place and reuse them throughout the app.
The ThemeData class in Flutter makes it easy to manage your app’s look and feel. Here’s an example of creating a custom theme:
```dart final ThemeData myTheme = ThemeData( primarySwatch: Colors.blue, brightness: Brightness.light, accentColor: Colors.red, fontFamily: 'Montserrat', textTheme: TextTheme( headline1: TextStyle(fontSize: 72.0, fontWeight: FontWeight.bold), headline6: TextStyle(fontSize: 36.0, fontStyle: FontStyle.italic), bodyText2: TextStyle(fontSize: 14.0, fontFamily: 'Hind'), ), ); ```
You can now apply this theme to your app using the MaterialApp widget:
```dart MaterialApp( title: 'My Flutter App', theme: myTheme, home: MyHomePage(), ); ```
This makes it easier to change your application’s aesthetic while ensuring consistency.
3. Harness the Potential of Custom Animations
Animations make your app lively and can significantly enhance user experience. Flutter provides a powerful animation library that can handle everything from simple to complex animations.
Let’s consider a basic example where we animate the opacity of a Flutter logo over a three-second duration:
```dart TweenAnimationBuilder( tween: Tween<double>(begin: 0, end: 1), duration: const Duration(seconds: 3), builder: (BuildContext context, double opacity, Widget? child) { return Opacity( opacity: opacity, child: FlutterLogo(size: 100), ); }, ); ```
This code uses the TweenAnimationBuilder widget to animate the logo’s opacity from fully transparent (0) to fully visible (1) over a period of three seconds.
4. Craft a Responsive Design
Building a responsive UI is essential to ensure your app looks good on various screen sizes. Flutter’s layout and flexible widget system make it easy to create a responsive design.
One key principle of responsive design is using relative rather than absolute dimensions. Here’s an example of using MediaQuery to get the screen width and adjust the layout accordingly:
```dart double screenWidth = MediaQuery.of(context).size.width; return Container( padding: EdgeInsets.all(screenWidth * 0.05), child: Text('Responsive UI in Flutter'), ); ```
In this example, we use 5% of the screen width as the padding for a container. The layout will now adapt to different screen sizes, maintaining relative proportions.
5. Experiment with Flutter’s CustomPainter
Flutter’s CustomPainter allows you to draw custom shapes and paths. This is an extremely powerful tool to create unique UI elements that can set your app apart.
Here’s an example where we draw a custom circle using CustomPaint and CustomPainter:
```dart CustomPaint( painter: CirclePainter(), child: Container(), ); class CirclePainter extends CustomPainter { @override void paint(Canvas canvas, Size size) { final paint = Paint() ..color = Colors.blue ..strokeWidth = 10 ..style = PaintingStyle.stroke; canvas.drawCircle(Offset(size.width/2, size.height/2), 100, paint); } @override bool shouldRepaint(covariant CustomPainter oldDelegate) { return false; } } ```
In the code above, we’ve created a custom painter that draws a blue circle.
Conclusion
Creating a beautiful UI is a process that combines creativity with the right tools and methodologies. With Flutter, you get a broad array of powerful tools to design stunning, interactive, and user-friendly interfaces. This is why many companies are looking to hire Flutter developers who can fully utilize the framework’s capabilities.
By leveraging the power of Flutter’s widgets, creating custom themes, using animations, designing responsively, and making use of the CustomPainter, you can significantly enhance the look and feel of your Flutter apps. This skillset is highly sought-after when businesses aim to hire Flutter developers.
Remember, a great UI is not just about looking good. It’s about creating an excellent user experience that keeps your users engaged and makes interaction with your app enjoyable. Flutter provides the perfect blend of tools and flexibility to help you achieve just that. So, whether you’re a Flutter developer or a business planning to hire Flutter developers, go ahead and experiment with these tips and tricks to create your beautiful Flutter UI!
Table of Contents
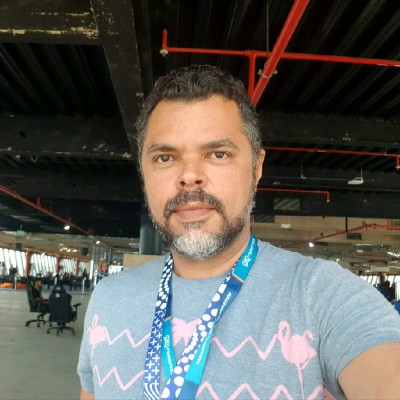
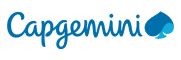