Flutter Q & A
How do you perform unit testing in a Flutter application?
Performing unit testing in a Flutter application is crucial for ensuring the reliability and maintainability of your codebase. Flutter provides a robust testing framework that integrates seamlessly with the Dart programming language. Here’s a comprehensive guide on how to perform unit testing in Flutter:
- Test Framework: Flutter uses the built-in `test` package for writing and executing tests. This package provides a straightforward way to structure and run tests within your project.
- Test Files: Tests in Flutter are typically organized into files named with the `_test.dart` suffix. These files contain functions annotated with `test` that define individual test cases.
- Testing Widgets: The `flutter_test` package allows you to write tests for widgets. You can use the `testWidgets` function to create a widget tester and interact with widgets in a test environment.
- Assertions: Dart provides a rich set of assertion functions (e.g., `expect`, `assert`) to check that your code behaves as expected. Use these assertions within your test functions to validate outcomes.
Example:
```dart import 'package:flutter_test/flutter_test.dart'; // Example class to be tested class Calculator { int add(int a, int b) => a + b; } void main() { group('Calculator Tests', () { test('Adding two numbers', () { // Arrange final calculator = Calculator(); // Act final result = calculator.add(2, 3); // Assert expect(result, 5); }); // Add more test cases as needed }); } ```
In this example, a simple `Calculator` class is tested for the `add` method using the `test` function and the `expect` assertion.
Running Tests:
- Use the terminal command `flutter test` to execute all tests in your project.
- Integration with CI/CD tools like Jenkins or GitHub Actions allows automated testing on each commit.
By incorporating unit testing into your Flutter development workflow, you can catch bugs early, improve code quality, and streamline the maintenance process. Regularly running tests ensures that your application remains stable and reliable throughout its lifecycle.
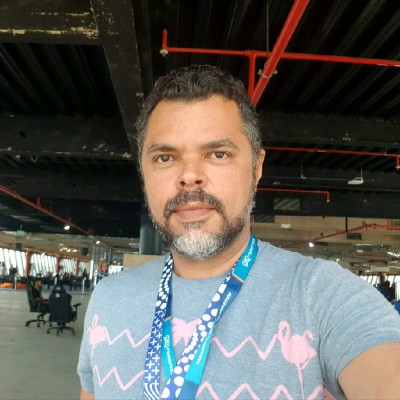
Previously at
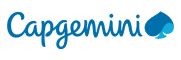
Full Stack Systems Analyst with a strong focus on Flutter development. Over 5 years of expertise in Flutter, creating mobile applications with a user-centric approach.