How do you handle user input in Flutter?
Handling user input in Flutter involves using various widgets and event handlers to capture and respond to user interactions. One fundamental widget for this purpose is the `TextField` widget, which allows users to input text. To handle the input, you can use the `onChanged` callback, which triggers whenever the text in the `TextField` changes. Here’s an example:
```dart TextField( onChanged: (text) { // Handle the user input here print("User input: $text"); }, decoration: InputDecoration( labelText: 'Enter text', ), ) ```
In this example, the `onChanged` callback captures the user’s input, and you can perform actions or validations based on the entered text.
For other types of input, such as button presses, you can use the `GestureDetector` widget or specialized widgets like `InkWell` or `GestureDetector`. For instance:
```dart GestureDetector( onTap: () { // Handle button press or tap here print("Button pressed!"); }, child: Container( padding: EdgeInsets.all(10), color: Colors.blue, child: Text('Press me'), ), ) ```
This `GestureDetector` responds to taps, and the `onTap` callback is where you handle the user input.
Key points for handling user input in Flutter include selecting the appropriate widget for the type of input, using callbacks like `onChanged` or `onTap` to capture user actions, and updating the UI or triggering relevant logic based on the user’s input. Always consider user experience and feedback to create responsive and intuitive interfaces.
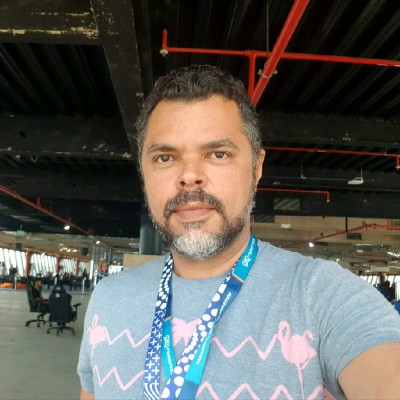
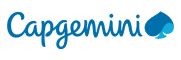