Building a Video Streaming App with Flutter: Integration and UI
In today’s digital age, video streaming apps have become an integral part of our lives. From entertainment to education, these apps serve a myriad of purposes. If you’re looking to create your own video streaming app with a sleek user interface, Flutter is the perfect choice. Flutter, Google’s UI toolkit, allows you to build natively compiled applications for mobile, web, and desktop from a single codebase.
Table of Contents
In this comprehensive guide, we’ll walk you through the process of building a video streaming app with Flutter. We’ll cover the crucial aspects of integrating video playback functionality and crafting a captivating user interface that will keep your users engaged. Let’s dive in!
1. Prerequisites
Before we get started, make sure you have the following prerequisites in place:
- Flutter Installed: Ensure that you have Flutter installed on your development machine. You can follow the official installation guide on the Flutter website.
- Video Content: You’ll need a source of video content to stream in your app. This could be videos hosted on a server, a YouTube API, or any other video source of your choice.
Now that you’re all set, let’s break down the process of building your video streaming app into two key components: integration and user interface design.
2. Integration of Video Playback
2.1. Choosing a Video Player Package
The first step in integrating video playback into your Flutter app is choosing a suitable video player package. There are several packages available, but for this tutorial, we’ll use the video_player package, which is the official video player package provided by Flutter.
To add video_player to your project, open your pubspec.yaml file and add the following dependency:
yaml dependencies: video_player: ^2.3.8
After adding the dependency, run flutter pub get to fetch the package.
2.2. Initializing Video Player
To use the video_player package, you need to initialize it in your Flutter app. Here’s a simple code snippet to get you started:
dart import 'package:flutter/material.dart'; import 'package:video_player/video_player.dart'; void main() => runApp(VideoApp()); class VideoApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Video Streaming App'), ), body: Center( child: VideoPlayerScreen(), ), ), ); } } class VideoPlayerScreen extends StatefulWidget { @override _VideoPlayerScreenState createState() => _VideoPlayerScreenState(); } class _VideoPlayerScreenState extends State<VideoPlayerScreen> { late VideoPlayerController _controller; @override void initState() { super.initState(); _controller = VideoPlayerController.network( 'https://www.example.com/sample_video.mp4') ..initialize().then((_) { // Ensure the first frame is shown and set state. setState(() {}); }); } @override Widget build(BuildContext context) { return AspectRatio( aspectRatio: _controller.value.aspectRatio, child: VideoPlayer(_controller), ); } @override void dispose() { super.dispose(); _controller.dispose(); } }
In this code, we create a simple Flutter app with an AppBar and a video player screen. The VideoPlayerScreen widget initializes the video_player controller with a network video URL. Remember to replace the URL with the actual video source you want to stream.
2.3. Controlling Video Playback
To control video playback, you can add playback controls like play, pause, and seek to your video player. Here’s a basic example of adding video controls:
dart class VideoPlayerScreen extends StatefulWidget { @override _VideoPlayerScreenState createState() => _VideoPlayerScreenState(); } class _VideoPlayerScreenState extends State<VideoPlayerScreen> { late VideoPlayerController _controller; @override void initState() { super.initState(); _controller = VideoPlayerController.network( 'https://www.example.com/sample_video.mp4') ..initialize().then((_) { setState(() {}); }); // Add listeners to the controller for state changes _controller.addListener(() { if (_controller.value.hasError) { // Handle any errors that occur during playback print('Video Player Error: ${_controller.value.errorDescription}'); } }); } @override Widget build(BuildContext context) { return Column( children: <Widget>[ AspectRatio( aspectRatio: _controller.value.aspectRatio, child: VideoPlayer(_controller), ), // Video playback controls Row( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ IconButton( icon: Icon(_controller.value.isPlaying ? Icons.pause : Icons.play_arrow), onPressed: () { setState(() { if (_controller.value.isPlaying) { _controller.pause(); } else { _controller.play(); } }); }, ), IconButton( icon: Icon(Icons.stop), onPressed: () { _controller.pause(); _controller.seekTo(Duration(seconds: 0)); }, ), ], ), ], ); } @override void dispose() { super.dispose(); _controller.dispose(); } }
In this code, we’ve added playback control buttons for play, pause, and stop. These buttons update the video playback state accordingly.
3. Designing the User Interface
With video playback integrated into your Flutter app, it’s time to focus on creating an engaging user interface. A well-designed UI can significantly enhance the user experience of your video streaming app.
3.1. Choosing a Design Theme
Start by selecting a design theme that aligns with the content and purpose of your app. Flutter offers various widgets and tools for creating beautiful user interfaces. You can customize the app’s theme using the Theme widget and predefined themes like ThemeData.light() or ThemeData.dark().
Here’s an example of setting the app’s theme:
dart return MaterialApp( theme: ThemeData.light(), home: Scaffold( appBar: AppBar( title: Text('Video Streaming App'), ), body: Center( child: VideoPlayerScreen(), ), ), );
3.2. Creating a Video List
In most video streaming apps, you’ll want to display a list of available videos. You can use Flutter’s ListView or GridView widgets to create a scrollable list of video thumbnails or titles.
Here’s an example of a simple video list using ListView.builder:
dart ListView.builder( itemCount: videoList.length, itemBuilder: (context, index) { return ListTile( leading: Image.network(videoList[index].thumbnailUrl), title: Text(videoList[index].title), onTap: () { // Handle video selection and navigation to the video player screen Navigator.push( context, MaterialPageRoute( builder: (context) => VideoPlayerScreen( videoUrl: videoList[index].videoUrl, ), ), ); }, ); }, )
In this code, videoList represents a list of video data, and each ListTile in the ListView displays a video thumbnail and title. When a user taps on a video, they are navigated to the video player screen.
3.3. Adding User Interactions
To make your app more interactive, consider adding features like user comments, likes, and sharing options. Flutter provides various widgets for handling user interactions, such as TextField for comments and IconButton for like and share buttons.
Here’s an example of adding a comment section to your video player screen:
dart Column( children: <Widget>[ AspectRatio( aspectRatio: _controller.value.aspectRatio, child: VideoPlayer(_controller), ), // Video playback controls (as shown earlier) // Comment section Padding( padding: const EdgeInsets.all(16.0), child: TextField( decoration: InputDecoration( hintText: 'Add a comment...', suffixIcon: IconButton( icon: Icon(Icons.send), onPressed: () { // Handle sending the comment String comment = _commentController.text; // Add your logic to post the comment to the server // and display it in the comment section. }, ), ), controller: _commentController, ), ), // Display comments here ], )
In this code, we’ve added a TextField for users to input comments and an IconButton for sending comments. You can customize the comment section further to display user comments and implement liking and sharing functionality.
3.4. Incorporating Responsive Design
To ensure your video streaming app looks great on various devices and screen sizes, implement responsive design principles. Flutter makes it easy to create responsive layouts using widgets like MediaQuery and LayoutBuilder.
dart return MaterialApp( theme: ThemeData.light(), home: Scaffold( appBar: AppBar( title: Text('Video Streaming App'), ), body: LayoutBuilder( builder: (context, constraints) { if (constraints.maxWidth > 600) { // Display a two-column layout on larger screens return TwoColumnLayout(); } else { // Display a single-column layout on smaller screens return VideoPlayerScreen(); } }, ), ), );
In this code, we use LayoutBuilder to determine the screen width and choose between a two-column layout for larger screens and a single-column layout for smaller screens.
Conclusion
Congratulations! You’ve learned how to integrate video playback functionality and design an engaging user interface for your video streaming app using Flutter. Building a video streaming app can be a complex endeavor, but with the right tools and knowledge, you can create a seamless and visually appealing experience for your users.
Remember that this tutorial provides a starting point, and you can expand upon it by adding more features, such as user authentication, playlists, and personalized recommendations. Flutter’s flexibility and extensive widget library make it an excellent choice for developing feature-rich video streaming applications.
Now it’s time to put your skills to the test and start building your own video streaming app with Flutter. Happy coding!
Note: Make sure to replace the example URLs, data, and functionality with your own video content and logic as needed for your specific app.
Table of Contents
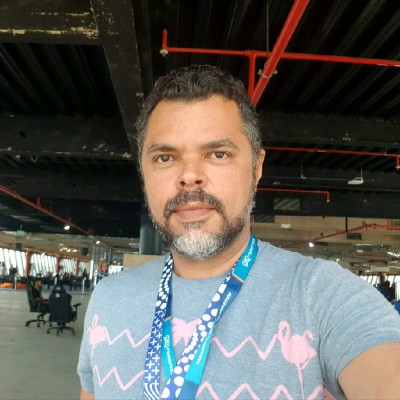
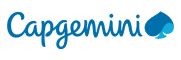