What is the Flutter widget tree?
The Flutter widget tree is a hierarchical structure that represents the user interface (UI) components of a Flutter application. In Flutter, everything is a widget, whether it’s a button, a layout, or even the entire application itself. The widget tree organizes these components in a parent-child relationship, defining how they are composed and displayed on the screen.
At the root of every Flutter application is the `main()` function, which returns the top-level widget. This widget, often a MaterialApp or a CupertinoApp, serves as the starting point for the widget tree. Within this main widget, various child widgets are nested to create the complete UI.
For instance, consider a simple Flutter app with a MaterialApp containing a Scaffold widget. The Scaffold, in turn, might have an AppBar, a body with a Column widget, and several children like Text and RaisedButton. Each of these widgets is a node in the widget tree, forming a hierarchy that determines their relationship and layout.
```dart void main() { runApp( MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter Widget Tree'), ), body: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Welcome to Flutter!'), RaisedButton( onPressed: () { // Button press logic }, child: Text('Click me'), ), ], ), ), ), ); } ```
Understanding the widget tree is crucial for efficient Flutter development. The tree’s structure influences how UI updates, event handling, and state management are handled. Developers can leverage this knowledge to optimize performance, manage state effectively, and create complex yet responsive user interfaces in their Flutter applications.
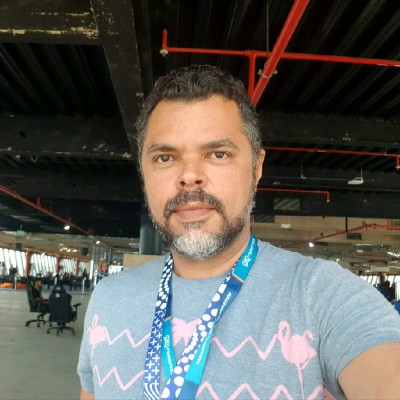
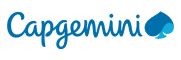