Flutter Widgets 101: Exploring Core Concepts for Efficient Development
Flutter, a comprehensive app SDK developed by Google, has taken the cross-platform mobile development world by storm. This has led many companies to hire Flutter developers to tap into its robust capabilities. Flutter enables developers to build natively compiled apps for web, mobile, and desktop from a single codebase. At the heart of Flutter’s magic lie its widgets – the fundamental building blocks of any Flutter app. Whether you’re looking to hire Flutter developers or aiming to master it yourself, understanding these crucial elements is essential. This article will guide you on a deep dive into mastering these widgets.
1. What Are Flutter Widgets?
Widgets in Flutter are similar to components in other frameworks like React or Vue.js. They describe what their view should look like given their current configuration and state. Flutter has a rich collection of widgets for creating complex UIs. They are organized into a hierarchical structure, forming a widget tree with parent-child relationships.
2. StatelessWidget and StatefulWidget
The most common types of widgets you’ll encounter in Flutter are `StatelessWidget` and `StatefulWidget`.
A `StatelessWidget` describes part of the user interface which can depend on configuration information in the widget itself and in the context in which the widget is inflated. However, once built, it cannot be changed. A common example is the `Text` widget:
```dart class MyStatelessWidget extends StatelessWidget { @override Widget build(BuildContext context) { return Text('Hello, Flutter!'); } } ```
On the other hand, a `StatefulWidget` can change over time, such as a widget that can move in response to user interactions. It can change – internally or externally – while still being presented in the UI. An example is the `Checkbox` widget:
```dart class MyCheckbox extends StatefulWidget { MyCheckbox({Key key}) : super(key: key); @override _MyCheckboxState createState() => _MyCheckboxState(); } class _MyCheckboxState extends State<MyCheckbox> { bool isChecked = false; @override Widget build(BuildContext context) { return Checkbox( value: isChecked, onChanged: (bool value) { setState(() { isChecked = value; }); }, ); } } ```
3. Commonly Used Flutter Widgets
3.1 Container
The `Container` widget is likely the most used one in Flutter. It’s a convenience widget that combines common painting, positioning, and sizing widgets. It can take only one child and aligns it within itself, optionally expanding to fill the available space:
```dart Container( color: Colors.blue, child: Text('Hello, Flutter!'), ) ```
3.2 Row and Column
`Row` and `Column` are flexible layout widgets that allow you to align child widgets along a horizontal or vertical axis, respectively. They are perfect when you need to position multiple widgets. For example:
```dart Row( mainAxisAlignment: MainAxisAlignment.spaceAround, children: <Widget>[ Icon(Icons.home), Icon(Icons.business), Icon(Icons.school), ], ) ```
3.3 Stack
The `Stack` widget allows for stacking multiple widgets over one another, forming a pile where each child widget fills the available space. It’s handy when creating overlays or layered designs:
```dart Stack( alignment: const Alignment(0.6, 0.6), children: [ CircleAvatar( backgroundImage: AssetImage('images/pic.jpg'), radius: 100.0, ), Container( decoration: BoxDecoration( color: Colors.black45, ), child: Text( 'Flutter', style: TextStyle( fontSize: 20.0, fontWeight: FontWeight.bold, color: Colors.white, ), ), ), ], ) ```
3.4 MaterialApp and Scaffold
`MaterialApp` is a widget that provides many widgets that are required for material design applications. It also makes use of the visual density in various contexts.
`Scaffold` provides a framework which implements the basic material design visual layout structure. It gives a set of APIs for showing drawers, snack bars, and bottom sheets:
```dart MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Hello Flutter'), ), body: Center( child: Text('This is the body of the Scaffold'), ), floatingActionButton: FloatingActionButton( child: Icon(Icons.add), onPressed: () { // Add your onPressed code here! }, ), ), ) ```
4. Leveraging Flutter’s Widget Catalog
Flutter’s extensive widget catalog helps you develop beautiful, customizable, and efficient UIs. The possibilities are endless, with options for text widgets, scrolling widgets, styling widgets, input widgets, and many more. A good rule of thumb is to break your UI into smaller pieces and use the best-suited widgets for each piece.
Flutter’s widget-oriented approach allows for a high level of code reuse, decreasing development time and potential for bugs. By truly understanding and mastering widgets, you will be well-equipped to tackle the most complex Flutter applications.
Take the time to familiarize yourself with the extensive [Flutter widget catalog] (https://flutter.dev/widgets/). It will be your go-to guide for finding the perfect widget for any situation.
Conclusion
Mastering Flutter widgets is an essential step towards becoming an efficient and productive Flutter developer. This expertise is precisely why many organizations choose to hire Flutter developers. Remember, Flutter is all about widgets – they are the framework’s building blocks. Learning to use them effectively allows developers, whether you hire Flutter developers or are one yourself, to create captivating UIs with ease and efficiency. So keep exploring, keep experimenting, and let your creativity flow with Flutter’s flexible widget system.
Table of Contents
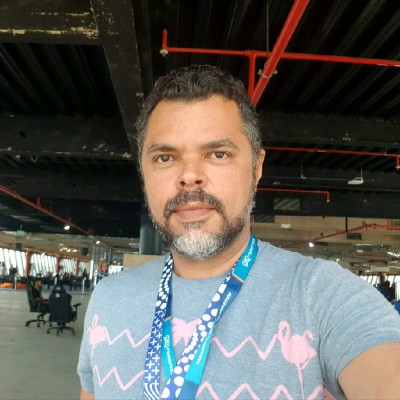
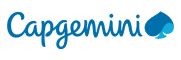