What is the defer statement used for in Go?
In Go, the defer statement is used to schedule a function call to be executed just before the surrounding function returns. It allows you to delay the execution of a function until the surrounding function exits, regardless of how it exits—whether it returns normally, panics, or encounters a runtime error.
The defer statement is commonly used for cleanup actions such as closing files, releasing resources, or performing finalization tasks. By deferring function calls, you ensure that cleanup actions are executed reliably and consistently, even in the presence of multiple return paths or error conditions.
The defer statement operates using a last-in, first-out (LIFO) stack, meaning that deferred function calls are executed in reverse order of their appearance in the code. This ensures that resources are released in the reverse order of their acquisition, helping to prevent resource leaks and maintain program correctness.
Here’s an example demonstrating the use of the defer statement in Go:
go func processFile(filename string) error { file, err := os.Open(filename) if err != nil { return err } defer file.Close() // Close the file when processFile returns // Process the file contents // ... return nil }
In this example, the defer statement is used to defer the call to file.Close() until the processFile function returns. This ensures that the file is closed properly, even if an error occurs during file processing or if the function returns prematurely.
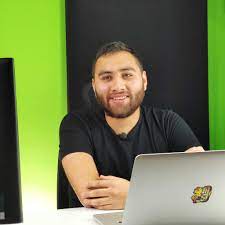
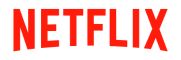