Go for DevOps: Automating Tasks and Infrastructure with Go
DevOps, the combination of software development and IT operations, has revolutionized the way organizations deliver software and manage their infrastructure. By automating tasks and infrastructure, DevOps practices enable faster deployment, enhanced scalability, and improved collaboration between development and operations teams. In this blog, we will explore how Go, a powerful programming language, can be leveraged to automate various aspects of your DevOps workflow, from provisioning infrastructure to continuous integration and deployment.
1. Getting Started with Go for DevOps
1.1 Why Go is a Perfect Fit for DevOps
Go, also known as Golang, is an open-source programming language developed by Google. It is designed for simplicity, efficiency, and ease of use. Go’s concise syntax and strong concurrency primitives make it an ideal choice for automating tasks and infrastructure in a DevOps context. Its compiled nature ensures fast execution, making it suitable for high-performance systems. Additionally, Go has a vast ecosystem of libraries and frameworks, allowing developers to leverage existing tools or build their own to tackle specific automation challenges.
1.2 Installing Go
To get started with Go, you need to install it on your machine. Visit the official Go website (https://golang.org) and download the appropriate installer for your operating system. Follow the installation instructions provided, and you’ll have Go up and running in no time.
1.3 Writing Your First Go Program
Let’s write a simple “Hello, World!” program in Go to ensure everything is set up correctly:
go package main import "fmt" func main() { fmt.Println("Hello, World!") }
Save this code in a file with a .go extension, such as hello.go. Open a terminal, navigate to the directory containing the file, and run the following command to compile and execute the program:
go go run hello.go
You should see the output Hello, World! printed on your screen.
2. Automating Infrastructure with Go
2.1 Provisioning Infrastructure with Infrastructure as Code (IaC)
Infrastructure as Code (IaC) is a practice that involves managing and provisioning infrastructure resources through code. Go can be used to automate the process of creating and configuring cloud resources, making it easier to manage complex infrastructure setups. Tools like Terraform, a popular IaC tool, have Go libraries that allow you to define infrastructure configurations programmatically. This enables you to version control your infrastructure and automate the provisioning process.
2.2 Example: Automating AWS EC2 Instance Creation with Go
Let’s take a look at a simple example of using Go to automate the creation of an Amazon Web Services (AWS) EC2 instance using the AWS SDK for Go:
go package main import ( "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/aws/session" "github.com/aws/aws-sdk-go/service/ec2" "fmt" ) func main() { // Create a new AWS session sess := session.Must(session.NewSessionWithOptions(session.Options{ SharedConfigState: session.SharedConfigEnable, })) // Create an EC2 service client svc := ec2.New(sess) // Specify the instance parameters input := &ec2.RunInstancesInput{ ImageId: aws.String("ami-12345678"), InstanceType: aws.String("t2.micro"), MinCount: aws.Int64(1), MaxCount: aws.Int64(1), } // Launch the EC2 instance result, err := svc.RunInstances(input) if err != nil { fmt.Println("Error launching instance:", err) return } // Print the instance ID fmt.Println("Instance ID:", *result.Instances[0].InstanceId) }
Make sure you have the AWS SDK for Go installed (go get -u github.com/aws/aws-sdk-go). Replace the ImageId and InstanceType with appropriate values. Running this program will create an EC2 instance and print its ID.
3. Continuous Integration and Deployment with Go
3.1 Building and Testing Go Projects
Go provides built-in tools for building and testing projects, which are crucial for continuous integration and deployment (CI/CD) pipelines. The go build command compiles a Go project into an executable binary, while go test runs tests defined in the project’s codebase. These commands can be integrated into your CI/CD pipelines to ensure that code changes are thoroughly tested before deployment.
3.2 Example: Go-Based CI/CD Pipeline with Jenkins
Jenkins is a popular open-source automation server that supports building, testing, and deploying software. Let’s see how Go can be used within a Jenkins pipeline to automate the build and test stages:
groovy pipeline { agent any stages { stage('Build') { steps { sh 'go build -o myapp' } } stage('Test') { steps { sh 'go test ./...' } } // Additional stages for deployment, etc. } }
In this example, the pipeline consists of two stages: build and test. The go build command compiles the Go project into an executable named myapp, while go test runs all tests in the project recursively (./…).
4. Conclusion
Go’s simplicity, efficiency, and concurrency features make it an excellent choice for automating tasks and infrastructure in a DevOps context. From provisioning infrastructure with IaC to building CI/CD pipelines, Go empowers DevOps teams to streamline their workflows and enhance productivity. By leveraging Go’s rich ecosystem and libraries, you can create robust automation solutions tailored to your specific needs. Embrace the power of Go and unlock the full potential of automation in your DevOps journey.
Table of Contents
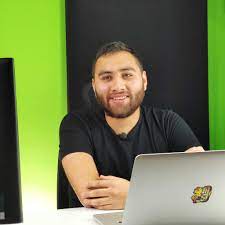
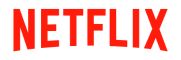