Go Q & A
How do you work with JSON data in Go?
In Go, working with JSON (JavaScript Object Notation) data is straightforward and efficient, thanks to the built-in encoding/json package, which provides functionalities for encoding and decoding JSON data into Go data structures and vice versa.
Here’s how you can work with JSON data in Go using the encoding/json package:
- Encoding JSON: To encode Go data structures into JSON format, you can use the json.Marshal function, which takes a Go value as input and returns its JSON representation as a byte slice. For example:
go package main import ( "encoding/json" "fmt" ) type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` } func main() { person := Person{Name: "John Doe", Age: 30, Email: "john@example.com"} jsonData, err := json.Marshal(person) if err != nil { fmt.Println("Error encoding JSON:", err) return } fmt.Println(string(jsonData)) }
- Decoding JSON: To decode JSON data into Go data structures, you can use the json.Unmarshal function, which takes a byte slice containing JSON data as input and populates a Go value with the decoded data. For example:
go package main import ( "encoding/json" "fmt" ) type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` } func main() { jsonData := []byte(`{"name":"Jane Doe","age":25,"email":"jane@example.com"}`) var person Person err := json.Unmarshal(jsonData, &person) if err != nil { fmt.Println("Error decoding JSON:", err) return } fmt.Println("Name:", person.Name) fmt.Println("Age:", person.Age) fmt.Println("Email:", person.Email) }
- Tagging Struct Fields: Go’s encoding/json package uses struct tags to determine how struct fields should be encoded or decoded from JSON. Struct tags are specified using backticks “ around field names and can include additional metadata such as field names, omitempty, and custom field mappings.
- Working with JSON Streams: Go’s encoding/json package supports streaming JSON data by using json.Encoder and json.Decoder types, which allow you to encode and decode JSON data incrementally from streams such as network connections or files.
By using the encoding/json package and its associated functionalities, developers can work with JSON data efficiently and seamlessly in Go applications, enabling interoperability with other systems and services that use JSON as a data interchange format.
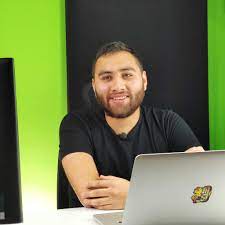
Previously at
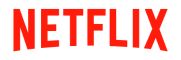
Over 5 years of experience in Golang. Led the design and implementation of a distributed system and platform for building conversational chatbots.