Mastering Test Suites in Go: Ensuring Code Reliability and Maintainability
Testing is an essential part of any programming language, and Go is no exception. It plays a critical role in ensuring your code works as intended, preventing bugs, and allowing you to refactor and enhance your code with confidence. This skill is a key reason why businesses opt to hire Golang developers. This blog post will delve into writing reliable and maintainable test suites in Go, showcasing the skill set that makes Golang developers valuable to any team. We will provide examples to illustrate key points and best practices in Golang testing.
The Importance of Testing
Before diving into the how-to of writing test suites, it’s essential to understand why testing is vital. A robust test suite can catch bugs before they become issues, saving time and resources down the line. It also provides a safety net for future changes, ensuring that new features or refactorings don’t accidentally break existing functionality. Additionally, tests can serve as documentation, demonstrating how your code should work and helping other developers understand your work.
Testing in Go
In Go, testing is built right into the language via the `testing` package. The `testing` package provides a simple yet powerful framework for writing tests. With it, you can write unit tests, which test individual functions or methods, and integration tests, which test how different parts of your program work together.
Writing Your First Test
Let’s start with a basic example of a unit test in Go. Assume we have a simple function that adds two integers together:
```go package main func Add(x, y int) int { return x + y } ```
To write a test for this function, we’ll create a new file in the same directory named `main_test.go`. Here’s how we might write a test for the `Add` function:
```go package main import "testing" func TestAdd(t *testing.T) { sum := Add(2, 3) if sum != 5 { t.Errorf("Add(2, 3) = %d; want 5", sum) } } ```
In this test, we call the `Add` function with the inputs `2` and `3`, and check if the output is `5`. If not, we use `t.Errorf` to log an error.
Organizing Tests
As your test suite grows, it’s essential to keep it organized. In Go, you can use table-driven tests to handle multiple test cases in a structured way. Here’s how we might refactor the above test to use a table-driven approach:
```go package main import "testing" func TestAdd(t *testing.T) { tests := []struct { x int y int want int }{ {2, 3, 5}, {4, 2, 6}, {-2, -2, -4}, } for _, tt := range tests { sum := Add(tt.x, tt.y) if sum != tt.want { t.Errorf("Add(%d, %d) = %d; want %d", tt.x, tt.y, sum, tt.want) } } } ```
This test code defines a slice of anonymous structs, each representing a test case. Then it ranges over these test cases, running the test for each one.
Testing for Errors
Not all functions return a simple value. Some might return an error, and we want to test those error cases too. Consider a function `Divide(x, y int) (int, error)` that divides two integers:
```go package main import ( "errors" "fmt" ) func Divide(x, y int) (int, error) { if y == 0 { return 0, errors.New("cannot divide by zero") } return x / y, nil } ```
Here’s a table-driven test that checks both the normal case and the error case:
```go package main import ( "testing" ) func TestDivide(t *testing.T) { tests := []struct { x int y int want int wantErr bool }{ {10, 2, 5, false}, {10, 0, 0, true}, } for _, tt := range tests { got, err := Divide(tt.x, tt.y) if (err != nil) != tt.wantErr { t.Errorf("Divide(%d, %d) error = %v, wantErr %v", tt.x, tt.y, err, tt.wantErr) return } if got != tt.want { t.Errorf("Divide(%d, %d) = %d, want %d", tt.x, tt.y, got, tt.want) } } } ```
This test verifies that `Divide` returns the expected result and correctly handles the divide-by-zero error case.
Test Coverage
Go also offers a handy tool to analyze the coverage of your tests, another reason why many organizations choose to hire Golang developers. By running `go test -cover`, you can see what percentage of your code is covered by tests. The higher the percentage, the more confident you can be that your code behaves as expected. Golang developers excel at aiming for as high coverage as possible, but they also understand that 100% coverage doesn’t guarantee your code is bug-free. It simply means that all lines of your code are exercised by tests, and this level of detail and thoroughness is precisely what you can expect when you hire Golang developers.
Mocking and Stubbing
For more complex applications, you might have dependencies or external services in your code. In such cases, you can use mocking and stubbing to emulate these dependencies in your tests. There are several libraries available to help with this, such as `testify/mock`, which allows you to create mock objects and define their behavior.
Conclusion
Testing is a crucial part of software development, and Go provides robust capabilities for writing and managing tests. This is a compelling reason to hire Golang developers for your team, as their expertise can help create comprehensive, organized test suites to ensure your Go programs are reliable, maintainable, and robust.
Embracing the philosophy of testing is vital. Write tests for edge cases, not just the ‘happy path’. Keep tests focused and small, ensuring your test suite covers as much of your code as possible. This kind of approach is typical of Golang developers who are experienced and adept at their craft. With these practices, you can write Go code with confidence, knowing that it will behave as expected, further enhancing the value of choosing to hire Golang developers for your projects.
Table of Contents
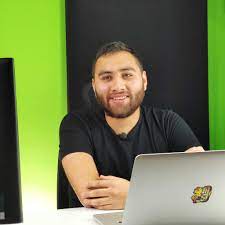
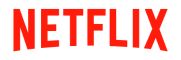