Creating RESTful APIs with Go and JSON Web Tokens (JWT)
In today’s digital landscape, creating robust and secure APIs is crucial for developing modern web applications. Go, a powerful programming language known for its simplicity and performance, coupled with JSON Web Tokens (JWT), a popular standard for authentication and authorization, provides an excellent foundation for building RESTful APIs. In this tutorial, we will explore the step-by-step process of creating RESTful APIs with Go and JWT, along with best practices for secure and efficient API development.
1. Understanding RESTful APIs and JSON Web Tokens (JWT):
1.1 What are RESTful APIs?
REST (Representational State Transfer) is an architectural style used to design networked applications, particularly web services. RESTful APIs enable communication between client and server over the HTTP protocol, utilizing the standard CRUD (Create, Read, Update, Delete) operations. These APIs are stateless and allow clients to access and manipulate resources through well-defined endpoints.
1.2 Introduction to JSON Web Tokens (JWT):
JSON Web Tokens (JWT) are a compact, URL-safe means of representing claims between two parties. They consist of three parts: a header, a payload, and a signature. The header specifies the type of token and the signing algorithm used, while the payload contains the claims or assertions. The signature is generated using a secret key and can be used to verify the integrity of the token.
2. Setting up the Development Environment:
2.1 Installing Go:
To get started, we need to install Go on our system. Visit the official Go website (golang.org) and download the appropriate installer for your operating system. Follow the installation instructions, and make sure to set the necessary environment variables.
2.2 Initializing a Go Module:
Go introduced a module system to manage dependencies effectively. Open a terminal and navigate to your project directory. Run the following command to initialize a new Go module:
bash go mod init example.com/myproject
Replace “example.com/myproject” with the actual name of your project.
2.3 Installing Required Packages:
Go provides excellent support for building web applications, and we will be using some external packages to enhance our API. Install the necessary packages using the following command:
bash go get -u github.com/gorilla/mux go get -u github.com/dgrijalva/jwt-go
These packages are popular in the Go ecosystem and offer convenient utilities for building RESTful APIs and handling JWT authentication.
3. Designing the API:
Before writing any code, it is essential to have a clear understanding of the API structure. Design your API endpoints based on the resources you want to expose. Define the HTTP methods (GET, POST, PUT, DELETE) that correspond to different operations on these resources.
3.1 Defining API Endpoints:
For example, let’s consider a simple blogging platform where users can create, read, update, and delete blog posts. We can define the following API endpoints:
- GET /posts: Retrieve a list of all blog posts
- POST /posts: Create a new blog post
- GET /posts/{id}: Retrieve a specific blog post
- PUT /posts/{id}: Update a specific blog post
- DELETE /posts/{id}: Delete a specific blog post
3.2 Establishing Resource Models:
Once the endpoints are defined, create resource models to represent the structure of the data being sent and received. For our blogging platform, a blog post model could have properties like “title,” “content,” and “author.”
3.3 Mapping HTTP Methods to API Operations:
Next, map the HTTP methods to functions that handle these operations. For example, the “GET /posts” endpoint can be mapped to a function that retrieves all blog posts from the database and returns them as a JSON response.
4. Implementing Authentication with JWT:
Secure APIs often require authentication to restrict access to authorized users. JWT provides a simple yet powerful mechanism for implementing authentication. Let’s explore how to generate and verify JWTs in Go.
4.1 Generating JWTs:
To generate a JWT, we need to create a token with the necessary claims and sign it with a secret key. Here’s an example of generating a JWT with a “username” claim:
go import ( "github.com/dgrijalva/jwt-go" "time" ) func generateJWT(username string) (string, error) { token := jwt.NewWithClaims(jwt.SigningMethodHS256, jwt.MapClaims{ "username": username, "exp": time.Now().Add(time.Hour * 24).Unix(), }) // Sign the token with a secret key return token.SignedString([]byte("your-secret-key")) }
In this code snippet, we use the jwt-go package to create a new token with the “username” claim and an expiration time of 24 hours. Finally, we sign the token using a secret key and return the generated JWT as a string.
4.2 Verifying and Parsing JWTs:
To verify and parse a JWT, we need to extract the token from the request, verify its integrity, and retrieve the claims. Here’s an example:
go func verifyJWT(tokenString string) (*jwt.Token, error) { token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) { // Check the signing method if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok { return nil, fmt.Errorf("unexpected signing method: %v", token.Header["alg"]) } // Provide the secret key to verify the token return []byte("your-secret-key"), nil }) if err != nil { return nil, err } return token, nil }
In this code snippet, we parse the token using the jwt.Parse method, providing a callback function to verify the signing method and return the secret key. If the token is successfully parsed and verified, we obtain the token object, which contains the claims.
4.3 Securing API Endpoints with JWT Authentication:
To secure specific API endpoints, we can add a middleware that validates the JWT before allowing access to the requested resource. Here’s an example of a middleware function:
go func authMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Extract the JWT from the request header or query parameter tokenString := extractToken(r) // Verify and parse the JWT token, err := verifyJWT(tokenString) if err != nil || !token.Valid { http.Error(w, "Unauthorized", http.StatusUnauthorized) return } // Pass the username from the token to the next handler ctx := context.WithValue(r.Context(), "username", token.Claims.(jwt.MapClaims)["username"]) next.ServeHTTP(w, r.WithContext(ctx)) }) }
In this middleware function, we extract the JWT from the request, verify its validity, and store the username from the token in the request context. If the token is invalid, we return an “Unauthorized” error.
5. Handling Request and Response:
To handle incoming requests and send appropriate responses, we need to parse the request payload and build JSON responses. Let’s see how it can be done.
5.1 Parsing Incoming JSON Requests:
When receiving JSON data in the request body, we can use the json.Unmarshal function to parse it into Go structs. Here’s an example:
go type BlogPost struct { Title string `json:"title"` Content string `json:"content"` Author string `json:"author"` } func createPost(w http.ResponseWriter, r *http.Request) { var post BlogPost err := json.NewDecoder(r.Body).Decode(&post) if err != nil { http.Error(w, "Bad Request", http.StatusBadRequest) return } // Process the blog post data // ... }
In this example, we define a BlogPost struct with corresponding JSON tags. We decode the request body into this struct using json.NewDecoder and handle any decoding errors accordingly.
5.2 Building JSON Responses:
To send JSON responses, we can utilize the json.Marshal function to serialize Go structs into JSON format. Here’s an example:
go func getPosts(w http.ResponseWriter, r *http.Request) { // Fetch blog posts from the database posts := fetchPosts() // Convert posts to JSON jsonData, err := json.Marshal(posts) if err != nil { http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } // Set appropriate headers and write JSON response w.Header().Set("Content-Type", "application/json") w.Write(jsonData) }
In this code snippet, we fetch the blog posts from the database and serialize them into JSON using json.Marshal. Finally, we set the appropriate headers and write the JSON response.
6. Testing and Debugging the API:
To ensure the correctness and reliability of our API, thorough testing and effective debugging are necessary. Let’s explore some essential techniques for testing and debugging Go APIs.
6.1 Unit Testing API Endpoints:
Go provides a robust testing framework in its standard library. We can write unit tests for each API endpoint to verify the expected behavior. Here’s an example:
go func TestGetPosts(t *testing.T) { // Create a mock HTTP request and response req := httptest.NewRequest("GET", "/posts", nil) w := httptest.NewRecorder() // Call the API handler function getPosts(w, req) // Check the response status code if w.Code != http.StatusOK { t.Errorf("expected status code %d, got %d", http.StatusOK, w.Code) } // Additional assertions on the response // ... }
In this test function, we create a mock HTTP request and response using the httptest package. We then call the API handler function and perform assertions on the response.
6.2 Debugging Techniques:
When encountering issues during API development, effective debugging techniques can greatly assist in identifying and resolving problems. Utilize the following techniques:
- Logging: Use the standard log package to print informative messages during runtime, aiding in understanding the flow of the program.
- Error Handling: Implement proper error handling to capture and log errors, providing insights into unexpected behavior.
- Debugging Tools: Leverage debugging tools like Delve or integrated development environments (IDEs) with built-in debugging capabilities to step through code and inspect variables.
7. Best Practices for Secure and Efficient API Development:
To build secure and efficient APIs, it’s crucial to follow best practices. Consider the following recommendations:
7.1 Input Validation and Sanitization:
Always validate and sanitize user input to prevent security vulnerabilities like SQL injection and cross-site scripting (XSS) attacks. Utilize frameworks or packages specifically designed for input validation, or implement custom validation logic.
7.2 Rate Limiting and Throttling:
Implement rate limiting and throttling mechanisms to prevent abuse and ensure fair resource allocation. Set limits on the number of requests per minute or hour, and handle excessive requests gracefully.
7.3 Error Handling and Logging:
Implement robust error handling mechanisms to catch and handle errors effectively. Log errors with appropriate details, including error codes, timestamps, and relevant contextual information, to aid in debugging and monitoring.
7.4 Performance Optimization:
Optimize API performance by employing techniques such as caching, database indexing, request/response compression, and efficient data retrieval and processing. Profile and benchmark your code to identify performance bottlenecks and optimize critical sections.
Conclusion:
Building RESTful APIs with Go and JSON Web Tokens (JWT) provides a secure and efficient foundation for modern web application development. By understanding the fundamentals of RESTful APIs, implementing JWT-based authentication, and following best practices, developers can create robust and reliable APIs. With the power and simplicity of Go, combined with the flexibility and security of JWT, you can build scalable and performant APIs for your next web application project. Happy coding!
Table of Contents
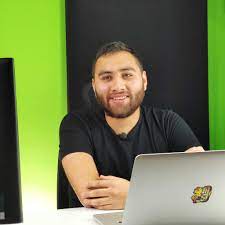
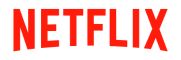