Securing Go Applications: Common Security Vulnerabilities and Mitigations
In the world of software development, security is of paramount importance. As more applications are built using the Go programming language (also known as Golang), it becomes crucial to understand the common security vulnerabilities that can affect Go applications and how to mitigate them effectively. This blog post aims to shed light on some prevalent security issues in Go applications and provide actionable insights into securing your codebase.
1. Introduction to Go Application Security
1.1. Understanding the Importance of Security
Security breaches can lead to data leaks, unauthorized access, and even financial losses. For Go applications, a robust security posture is essential to protect sensitive user information and maintain the trust of your users. Ignoring security concerns can result in severe consequences for both your users and your organization.
1.2. Go’s Growing Popularity and Security Challenges
Go’s simplicity, performance, and strong concurrency support have contributed to its increasing popularity for building various applications, including web services and microservices. However, as Go applications become more prevalent, they also become more attractive targets for attackers. This makes it imperative for developers to be aware of potential security vulnerabilities and adopt best practices to secure their applications.
2. Common Security Vulnerabilities in Go Applications
2.1. Cross-Site Scripting (XSS)
XSS is a type of attack where an attacker injects malicious scripts into a web application, which are then executed in the context of an unsuspecting user’s browser. This can lead to theft of sensitive information or unauthorized actions on behalf of the user. To prevent XSS attacks in Go applications, developers should ensure proper input validation and output encoding.
go package main import ( "fmt" "html/template" "net/http" ) func main() { http.HandleFunc("/hello", func(w http.ResponseWriter, r *http.Request) { input := r.URL.Query().Get("name") tmpl := template.Must(template.New("").Parse("Hello, {{.}}!")) tmpl.Execute(w, input) }) http.ListenAndServe(":8080", nil) }
In the example above, using the template package for output rendering automatically escapes the input variable, preventing potential XSS attacks.
2.2. SQL Injection
SQL injection occurs when an attacker manipulates input to execute malicious SQL queries on a database. This can lead to unauthorized data access or even database manipulation. To prevent SQL injection in Go applications, developers should use prepared statements or an Object-Relational Mapping (ORM) library.
go package main import ( "database/sql" "fmt" "log" "net/http" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(localhost:3306)/database") if err != nil { log.Fatal(err) } defer db.Close() http.HandleFunc("/user", func(w http.ResponseWriter, r *http.Request) { username := r.URL.Query().Get("username") query := fmt.Sprintf("SELECT * FROM users WHERE username = '%s'", username) rows, err := db.Query(query) // ... }) http.ListenAndServe(":8080", nil) }
Using placeholders in the query and parameter binding, as provided by prepared statements, helps prevent SQL injection attacks.
2.3. Cross-Site Request Forgery (CSRF)
CSRF attacks involve tricking users into performing unwanted actions on a different site where they are authenticated. To mitigate CSRF attacks, developers should implement anti-CSRF tokens that are generated per user session and validated before processing sensitive requests.
go package main import ( "fmt" "net/http" "time" "github.com/gorilla/csrf" "github.com/gorilla/mux" "github.com/gorilla/sessions" ) func main() { store := sessions.NewCookieStore([]byte("super-secret-key")) r := mux.NewRouter() r.HandleFunc("/transfer", func(w http.ResponseWriter, r *http.Request) { session, _ := store.Get(r, "session-name") if session.IsNew { http.Error(w, "Unauthorized", http.StatusUnauthorized) return } token := csrf.Token(r) // Use token for validation // ... }) http.ListenAndServe(":8080", csrf.Protect([]byte("32-byte-long-auth-key"))(r)) }
The example demonstrates how to use the Gorilla CSRF package to protect against CSRF attacks by generating and validating tokens.
2.4. Insecure Dependencies
Go applications often rely on third-party packages and libraries. However, if these dependencies have security vulnerabilities, they can introduce risks into your application. To mitigate this, developers should regularly update their dependencies to the latest secure versions and use tools like go list and go mod tidy to identify and resolve vulnerabilities.
bash go list -m -u all go mod tidy
2.5. Improper Error Handling
Improper error handling can lead to unexpected application behavior and potentially expose sensitive information. It’s essential to handle errors gracefully and avoid exposing internal details to users or attackers.
go package main import ( "database/sql" "fmt" "log" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(localhost:3306)/database") if err != nil { log.Fatal(err) } defer db.Close() // Incorrect error handling _, err = db.Exec("INSERT INTO users (username) VALUES ('new_user')") if err != nil { fmt.Println("An error occurred:", err) } }
In the example above, the error message err is being printed directly, which might leak sensitive information. Instead, consider logging only necessary information without revealing implementation details.
3. Mitigating Security Vulnerabilities
3.1. Input Validation and Sanitization
A key step in preventing various security vulnerabilities is thorough input validation and sanitization. Sanitizing user inputs, such as removing or escaping potentially malicious characters, helps protect against attacks like XSS.
3.2. Prepared Statements and ORM
Using prepared statements or ORM libraries is critical for preventing SQL injection. These methods ensure that user inputs are treated as data, not executable code.
3.3. Anti-CSRF Tokens
Implementing anti-CSRF tokens prevents unauthorized requests from being executed on behalf of users. These tokens are unique to each session and request, adding an extra layer of security.
3.4. Dependency Auditing
Regularly audit and update your application’s dependencies to avoid using outdated packages with known vulnerabilities. Tools like go list and third-party security scanners can help identify and address these issues.
3.5. Comprehensive Error Handling
Handle errors gracefully and provide users with only necessary information. Avoid exposing implementation details that could be exploited by attackers.
4. Best Practices for Go Application Security
4.1. Keep Dependencies Updated
Stay vigilant about your application’s dependencies and ensure they are regularly updated to the latest secure versions. Set up automated processes to regularly check for updates and vulnerabilities.
4.2. Utilize Security Tools
Leverage security tools and scanners like gosec and GoAudit to analyze your code for potential vulnerabilities and security weaknesses.
4.3. Implement Role-Based Access Control
Implement role-based access control (RBAC) to ensure that users have appropriate permissions based on their roles. This reduces the risk of unauthorized access.
4.4. Regular Security Audits
Conduct regular security audits of your codebase and infrastructure. Identify potential vulnerabilities, assess risks, and implement necessary fixes.
Conclusion
Securing Go applications is an ongoing process that demands a proactive approach from developers. By understanding and addressing common security vulnerabilities like XSS, SQL injection, CSRF, and improper error handling, you can significantly enhance the security of your Go applications. Utilizing best practices, staying informed about security updates, and conducting regular security audits will contribute to a safer and more robust application ecosystem. Remember, investing in security today can save you from potential disasters in the future.
Table of Contents
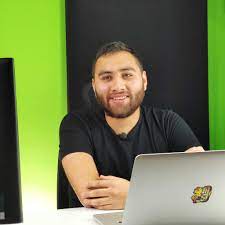
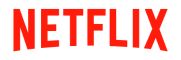