Go for Web Scraping: Extracting Data from Websites with Ease
Web scraping has become an essential technique for gathering data from the vast expanse of the internet. It allows developers, researchers, and businesses to extract valuable information from websites quickly and efficiently. While many programming languages support web scraping, Go, also known as Golang, offers a unique set of features and benefits that make it an excellent choice for this task.
In this blog, we will explore why Go is an ideal language for web scraping and dive into various aspects of web scraping using Go. We’ll cover essential concepts, popular libraries, and best practices, along with code samples to demonstrate the power and simplicity of Go in extracting data from websites.
1. Why Choose Go for Web Scraping?
Before we delve into the how-to’s of web scraping with Go, let’s take a moment to understand why Go is a great choice for this purpose.
- Efficiency: Go is renowned for its impressive performance and efficiency. It compiles down to native code, making it lightning-fast, which is crucial when scraping large volumes of data from multiple websites.
- Concurrency: Go’s built-in concurrency features, such as Goroutines and channels, make it exceptionally suitable for handling multiple web requests simultaneously. This means you can scrape data from multiple pages or sites concurrently, drastically reducing scraping time.
- Strong Standard Library: Go’s standard library includes excellent packages for handling HTTP requests, parsing HTML, and managing network connections, making it a breeze to work with web scraping.
- Static Binary: Go compiles into a single static binary, making it easy to distribute and deploy your web scraping applications without external dependencies.
- Community and Third-Party Libraries: The Go community actively contributes to a wide range of third-party libraries that further streamline the web scraping process. You’ll find a plethora of tools and packages to parse HTML, interact with APIs, and navigate websites.
Now that we understand the advantages of using Go for web scraping, let’s dive into the practical aspects of extracting data from websites.
2. Setting Up the Environment
To get started with web scraping in Go, you need to set up your development environment. Follow these steps to get Go installed and ready:
- Install Go: Visit the official Go website (https://golang.org/) and download the installer for your operating system. Install Go by following the installation instructions provided for your OS.
- Verify Installation: After installation, open your terminal or command prompt and run the following command to verify that Go is installed correctly:
bash go version
If everything is set up correctly, you should see the installed Go version printed in the terminal.
- Choose a Text Editor or IDE: Go is highly versatile and can be used with a variety of text editors and Integrated Development Environments (IDEs). Some popular choices include Visual Studio Code, GoLand, Sublime Text, and Vim. Choose the one that suits your preferences and start coding!
With your environment set up, it’s time to move on to the core concepts of web scraping in Go.
3. Understanding the Core Concepts of Web Scraping
Before diving into the code, it’s essential to grasp the core concepts of web scraping. These concepts will form the foundation of your scraping efforts and help you navigate the intricacies of different websites.
3.1. HTTP Requests:
To scrape data from a website, your Go program needs to send HTTP requests to the web server hosting the site. The server responds with the HTML content of the page, which your program can then parse and extract the desired information.
3.2. HTML Parsing:
HTML is the markup language used to structure the content of websites. To extract data, you’ll need to parse the HTML and navigate through its elements, such as tags, attributes, and text nodes. Fortunately, Go offers several libraries to help with HTML parsing, such as “golang.org/x/net/html” and “github.com/PuerkitoBio/goquery.”
3.3. Navigating Websites:
Websites often consist of multiple pages and interconnected links. To scrape data from multiple pages or follow links to reach specific content, you need to handle website navigation effectively. This involves identifying and following links programmatically.
3.4. Handling JavaScript Rendering:
Some websites load data dynamically using JavaScript. When using Go for web scraping, you have two options: either parse the static HTML content or use a headless browser with JavaScript support to scrape dynamically rendered pages.
With these core concepts in mind, let’s proceed to the implementation of web scraping in Go using various libraries and techniques.
4. Scraping Websites with Go’s “net/http” Package
Go’s standard library provides the “net/http” package, which allows us to send HTTP requests and handle responses. This package forms the basis of simple web scraping tasks that do not require JavaScript rendering. Let’s see an example of how to use it to scrape data from a website.
go package main import ( "fmt" "io/ioutil" "net/http" ) func main() { url := "https://example.com" response, err := http.Get(url) if err != nil { fmt.Printf("Error fetching URL: %s", err) return } defer response.Body.Close() body, err := ioutil.ReadAll(response.Body) if err != nil { fmt.Printf("Error reading response body: %s", err) return } fmt.Println(string(body)) }
In this code, we send a GET request to “https://example.com” and read the response body. Note that this approach is suitable for static pages without JavaScript rendering. For more complex scenarios, we’ll explore other options.
5. Scraping Websites with “github.com/PuerkitoBio/goquery”
For parsing HTML and navigating websites, the “github.com/PuerkitoBio/goquery” package is a powerful tool. It provides a jQuery-like syntax to traverse the HTML DOM tree with ease. Let’s see an example of using “goquery” to extract specific data from a website.
go package main import ( "fmt" "log" "github.com/PuerkitoBio/goquery" ) func main() { url := "https://example.com" doc, err := goquery.NewDocument(url) if err != nil { log.Fatal("Error fetching URL:", err) } doc.Find(".product").Each(func(i int, s *goquery.Selection) { productName := s.Find(".product-name").Text() price := s.Find(".price").Text() fmt.Printf("Product %d: %s - Price: %s\n", i+1, productName, price) }) }
In this example, we use “goquery” to fetch the HTML content of a webpage, and then we extract product names and prices from elements with the “product” class.
6. Scraping JavaScript-Rendered Websites with “chromedp”
For websites that rely on JavaScript rendering, the “github.com/chromedp/chromedp” package is a powerful solution. It allows you to use a headless browser (Chrome or Edge) to render the page and then extract the data.
Before using “chromedp,” you need to install the Chrome or Edge browser and ensure it’s in your system’s PATH.
go package main import ( "context" "fmt" "github.com/chromedp/chromedp" ) func main() { url := "https://example.com" ctx, cancel := chromedp.NewContext(context.Background()) defer cancel() var pageTitle string err := chromedp.Run(ctx, chromedp.Navigate(url), chromedp.Title(&pageTitle), ) if err != nil { fmt.Println("Error navigating to URL:", err) return } fmt.Println("Page Title:", pageTitle) }
In this example, we use “chromedp” to navigate to “https://example.com” and extract the page’s title. This approach enables us to interact with JavaScript-rendered content.
7. Best Practices for Web Scraping in Go
As you engage in web scraping, it’s essential to follow some best practices to ensure you are respectful of the websites you are scraping and to minimize the risk of being blocked or causing disruption.
- Respect Robots.txt: Always review the website’s “robots.txt” file before scraping. This file provides guidelines on what content can be scraped and what should be avoided.
- Use Delays: Avoid aggressive scraping by implementing delays between requests. Respect the server’s response time to reduce the load on their end.
- Crawl Politely: Don’t overwhelm the website with too many requests at once. Space them out, and limit the number of concurrent requests to avoid straining the server.
- User-Agent Spoofing: Some websites may block default user-agents. Use a common user-agent or customize your user-agent to appear more like a regular browser.
- Monitor Website Changes: Websites may update their structure or policies, leading to broken scrapers. Regularly check if your scraping code is still functioning correctly.
Conclusion
Go is a powerful and efficient language for web scraping, offering strong standard libraries and a supportive community. Armed with the knowledge of core web scraping concepts, you can now explore different websites, extract valuable data, and put it to use for your projects or business needs. Remember to adhere to best practices to be a responsible web scraper and make the most out of Go’s capabilities for extracting data from websites with ease. Happy scraping!
Table of Contents
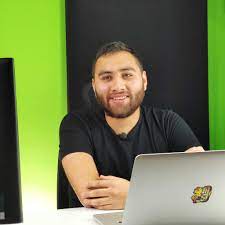
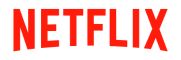