Exploring Core Bluetooth in iOS: Building Connected Apps
In the world of mobile applications, the ability to establish connections and communicate with external devices has opened up a whole new realm of possibilities. Whether it’s a fitness tracker, a smart thermostat, or wireless headphones, the magic that makes these devices work often involves Bluetooth technology. In the realm of iOS development, Core Bluetooth is the framework that empowers developers to create apps that can seamlessly interact with and control Bluetooth-enabled peripherals. In this blog post, we’ll embark on a journey to explore Core Bluetooth in iOS and learn how to build connected apps that harness the power of this technology.
Table of Contents
1. Understanding Bluetooth Basics
Before we dive into the intricacies of Core Bluetooth, let’s grasp the basics of Bluetooth technology. Bluetooth is a wireless communication protocol that allows devices to exchange data over short distances. It operates in the 2.4 GHz frequency range and is widely used for connecting devices such as smartphones, laptops, and IoT gadgets. Bluetooth devices typically assume one of two roles in a connection: central or peripheral.
- Central: This is the device that initiates the connection and scans for nearby peripherals. It’s the active participant in the communication process.
- Peripheral: This is the device that broadcasts its presence and waits for a central device to establish a connection. Once connected, the peripheral can send data to the central.
2. Introducing Core Bluetooth
In iOS, Core Bluetooth is the framework that enables developers to interact with Bluetooth devices. It provides an abstraction layer that hides much of the underlying complexity, making it easier to work with Bluetooth peripherals. Let’s take a look at the key components and concepts within Core Bluetooth.
2.1. CBCentralManager
The CBCentralManager class is at the heart of the central role. It manages the discovery and connection to peripheral devices. Here’s how you can set up a CBCentralManager instance:
swift import CoreBluetooth class MyCentralViewController: UIViewController, CBCentralManagerDelegate { var centralManager: CBCentralManager! override func viewDidLoad() { super.viewDidLoad() centralManager = CBCentralManager(delegate: self, queue: nil) } // Implement delegate methods here... }
2.2. CBPeripheral
On the peripheral side, we have the CBPeripheral class, which represents a Bluetooth peripheral device. It exposes services and characteristics that define the data being exchanged.
swift func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String: Any], rssi RSSI: NSNumber) { if peripheral.name == "MyDeviceName" { // Connect to the peripheral centralManager.connect(peripheral, options: nil) } }
2.3. Services and Characteristics
Services represent a logical collection of data and behavior on the peripheral. Characteristics, on the other hand, are the actual data points that can be read from or written to. For instance, a heart rate monitor might have a “Heart Rate Measurement” characteristic within a “Heart Rate Service.”
swift func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) { guard let services = peripheral.services else { return } for service in services { if service.uuid == CBUUID(string: "HeartRateServiceUUID") { peripheral.discoverCharacteristics(nil, for: service) } } }
3. Establishing Connections
The process of establishing a connection between a central and a peripheral involves scanning for nearby peripherals, discovering services and characteristics, and then interacting with the desired data. Here’s a simplified breakdown:
- Scan for peripherals using the central manager.
- Discover services on the peripheral after it’s discovered.
- Explore characteristics within each service.
- Read from or write to specific characteristics as needed.
4. Building a Connected App
Let’s put theory into practice by building a simple iOS app that connects to a hypothetical Bluetooth-enabled light bulb. Our app will discover the light bulb, toggle its state, and receive updates about its state changes.
4.1. Setting Up the Project
Create a new Xcode project and choose the “Single View App” template. In the project settings, make sure to enable the “Background Modes” capability and check the “Uses Bluetooth LE accessories” option.
4.2. Scanning for Peripherals
First, import the Core Bluetooth framework and create a CBCentralManager instance in your view controller. Don’t forget to make your view controller conform to the CBCentralManagerDelegate protocol.
swift import CoreBluetooth class LightBulbViewController: UIViewController, CBCentralManagerDelegate { var centralManager: CBCentralManager! override func viewDidLoad() { super.viewDidLoad() centralManager = CBCentralManager(delegate: self, queue: nil) } // Implement delegate methods... }
In the centralManagerDidUpdateState delegate method, you should check if the central manager’s state is .poweredOn before initiating scanning.
swift func centralManagerDidUpdateState(_ central: CBCentralManager) { if central.state == .poweredOn { centralManager.scanForPeripherals(withServices: nil, options: nil) } else { // Handle other states... } }
4.3. Discovering Services and Characteristics
Once a peripheral is discovered, the didDiscover peripheral delegate method is called. In this method, you can check if the discovered peripheral is the light bulb you’re looking for and then proceed to discover its services and characteristics.
swift func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String: Any], rssi RSSI: NSNumber) { if peripheral.name == "LightBulb" { centralManager.connect(peripheral, options: nil) } }
Upon successful connection, the peripheral(_:didDiscoverServices:) method will be called. In this method, you can discover the services and characteristics of the peripheral.
swift func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) { guard let services = peripheral.services else { return } for service in services { peripheral.discoverCharacteristics(nil, for: service) } }
4.4. Interacting with Characteristics
Once the characteristics are discovered, you can interact with them. For our hypothetical light bulb, let’s assume it has a characteristic for toggling its state.
swift func peripheral(_ peripheral: CBPeripheral, didDiscoverCharacteristicsFor service: CBService, error: Error?) { guard let characteristics = service.characteristics else { return } for characteristic in characteristics { if characteristic.uuid == CBUUID(string: "ToggleCharacteristicUUID") { // Toggle the light bulb's state let data = Data([0x01]) // Assuming 0x01 toggles the light on peripheral.writeValue(data, for: characteristic, type: .withResponse) } } }
4.5. Receiving Updates
If the light bulb’s state changes, the peripheral will notify the central through the characteristic you’ve set up. To receive these updates, implement the peripheral(_:didUpdateValueFor:error:) delegate method.
swift func peripheral(_ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error?) { if characteristic.uuid == CBUUID(string: "ToggleCharacteristicUUID") { if let data = characteristic.value, data == Data([0x01]) { // Light bulb is now on } else { // Light bulb is now off } } }
Conclusion
Core Bluetooth opens the door to building captivating iOS apps that seamlessly interact with Bluetooth peripherals. From fitness trackers to smart home devices, the ability to control and monitor external devices through your app can greatly enhance user experiences. By understanding the basics of Bluetooth communication, diving into the Core Bluetooth framework, and applying these concepts in your projects, you’ll be well on your way to crafting innovative and connected applications that leverage the power of wireless communication. So, go ahead and embark on your journey to explore the fascinating world of Core Bluetooth in iOS development!
Table of Contents
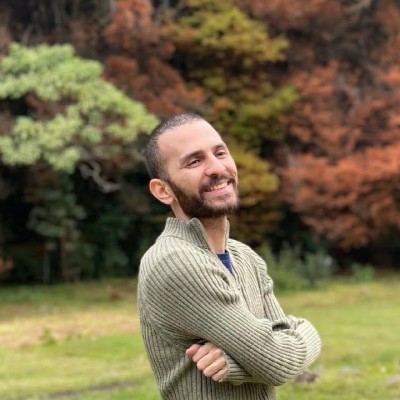
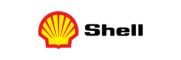