Understanding Grand Central Dispatch in iOS: Concurrency Made Easy
In the ever-evolving world of iOS app development, creating responsive and efficient applications is crucial. As user expectations rise, so does the demand for seamless multitasking and smooth performance. This is where Grand Central Dispatch (GCD) comes to the rescue. GCD is Apple’s solution for managing concurrency and parallelism in iOS applications. Whether you’re a seasoned developer or just starting, understanding GCD can significantly enhance your app’s performance and responsiveness. In this blog post, we’ll delve into the depths of GCD, exploring its concepts, benefits, and providing you with practical code samples.
Table of Contents
1. Introduction to Concurrency:
Concurrency refers to the ability of an application to execute multiple tasks independently, potentially at the same time. In iOS app development, concurrency plays a crucial role in maintaining smooth user experiences, particularly as devices become more powerful and capable of handling multiple operations simultaneously. However, managing concurrent tasks manually can be complex and error-prone.
2. Introducing Grand Central Dispatch (GCD):
Grand Central Dispatch, often abbreviated as GCD, is a powerful and efficient framework provided by Apple for managing concurrent tasks in iOS applications. GCD abstracts away much of the complexity of managing threads and queues, making it easier for developers to write efficient and responsive code.
2.1. Core Concepts of GCD:
At the heart of GCD are queues. Queues are responsible for managing the execution of tasks in a concurrent and organized manner. GCD introduces two main types of queues: serial queues and concurrent queues.
2.3.1. Serial Queues:
Serial queues execute tasks in a sequential order, one at a time. This ensures that only one task is being executed at any given moment, providing a straightforward way to manage access to resources that can’t be used concurrently.
swift let serialQueue = DispatchQueue(label: "com.example.serialQueue") serialQueue.sync { // Perform task 1 } serialQueue.sync { // Perform task 2 (waits for task 1 to complete) }
2.3.2. Concurrent Queues:
Concurrent queues, on the other hand, can execute multiple tasks concurrently. This is useful for tasks that are independent of each other and can be safely executed simultaneously.
swift let concurrentQueue = DispatchQueue(label: "com.example.concurrentQueue", attributes: .concurrent) concurrentQueue.async { // Perform task A } concurrentQueue.async { // Perform task B (can run concurrently with task A) }
2.4. Advantages of Using GCD:
- Simplicity: GCD abstracts away low-level thread management, making it easier to write safe concurrent code.
- Performance: GCD automatically optimizes the number of threads used, improving overall application performance.
- Resource Management: GCD helps prevent issues like thread leaks and deadlocks by managing resources efficiently.
- Battery Efficiency: GCD’s intelligent scheduling reduces unnecessary CPU usage, leading to better battery life.
- Responsive UI: GCD’s ability to offload time-consuming tasks from the main thread ensures that the UI remains responsive.
3. Dispatching Work with GCD:
GCD provides two main ways to dispatch tasks: synchronously and asynchronously.
3.1. Dispatching Tasks Synchronously:
Synchronous dispatching blocks the current thread until the dispatched task completes.
swift let serialQueue = DispatchQueue(label: "com.example.serialQueue") serialQueue.sync { // Perform a time-consuming task } // Code here waits for the task to finish
3.2. Dispatching Tasks Asynchronously:
Asynchronous dispatching allows the current thread to continue its execution while the dispatched task runs in the background.
swift let concurrentQueue = DispatchQueue(label: "com.example.concurrentQueue", attributes: .concurrent) concurrentQueue.async { // Perform a task asynchronously } // Code here continues executing immediately
4. Managing Priorities with Queues:
GCD provides Quality of Service (QoS) classes that allow you to specify the priority of tasks in a queue.
4.1. Understanding QoS Classes:
- .userInteractive: High-priority tasks that require immediate user interaction.
- .userInitiated: Tasks initiated by the user that should complete quickly.
- .default: The default priority level for tasks.
- .utility: Long-running tasks that don’t require immediate completion.
- .background: Tasks that can run in the background without affecting the user experience.
4.2. Choosing the Right QoS Class for Tasks:
swift let highPriorityQueue = DispatchQueue(label: "com.example.highPriority", qos: .userInteractive) highPriorityQueue.async { // Perform high-priority task } let backgroundQueue = DispatchQueue(label: "com.example.background", qos: .background) backgroundQueue.async { // Perform background task }
5. Grouping Tasks:
GCD allows you to group multiple tasks together using dispatch groups.
5.1. Using Dispatch Groups:
swift let group = DispatchGroup() let queue = DispatchQueue.global() queue.async(group: group) { // Perform task 1 } queue.async(group: group) { // Perform task 2 } group.notify(queue: .main) { // Code here executes when all tasks are completed }
5.2. Handling Group Notifications:
The group.notify method is used to specify a closure that executes when all tasks in the group have finished.
6. Barrier Tasks for Data Safety:
In scenarios where data needs to be accessed safely, GCD provides a mechanism called barrier tasks.
6.1. Synchronizing Data Access:
swift let concurrentQueue = DispatchQueue(label: "com.example.concurrentQueue", attributes: .concurrent) var sharedData = [Int]() concurrentQueue.async(flags: .barrier) { // Write to sharedData safely } concurrentQueue.async { let dataCopy = concurrentQueue.sync { // Read from sharedData safely return sharedData } }
6.2. Implementing Barrier Tasks:
Barrier tasks ensure that the dispatched task is the only one running in the queue, allowing safe read/write operations.
7. Global and Main Queues:
GCD provides two special queues: global queues and the main queue.
7.1. Global Queues for Common Tasks:
Global queues are concurrent queues that are created and managed by the system. They are designed for common tasks of various priorities.
swift let globalQueue = DispatchQueue.global(qos: .userInitiated) globalQueue.async { // Perform a task on a global queue }
7.2. The Main Queue for UI Updates:
The main queue is a serial queue specifically designed for UI updates. All UI-related tasks should be dispatched to the main queue to ensure a responsive user interface.
swift DispatchQueue.main.async { // Update UI elements here }
8. GCD in Real-world Scenarios:
Let’s explore a few real-world scenarios where GCD shines:
- Fetching Data from a Remote Server: Use GCD to make asynchronous network requests without blocking the main thread.
- Parallel Image Processing: Apply filters or transformations to images concurrently to enhance user experience.
- Background Data Loading: Load data in the background while keeping the UI responsive.
9. Debugging and Pitfalls:
While GCD greatly simplifies concurrent programming, there are still some common mistakes and challenges to be aware of.
9.1. Debugging GCD-related Issues:
Use Xcode’s debugging tools to identify issues related to deadlocks or thread contention.
Ensure that tasks dispatched to the main queue don’t cause UI freezes.
9.2. Common Mistakes to Avoid:
Deadlocks: Make sure not to block the main thread while waiting for tasks that can’t complete.
Overusing Dispatch Groups: Be cautious with dispatch groups, as overusing them might lead to unnecessary complexity.
Thread Explosion: Avoid creating too many threads, as it can degrade performance.
Conclusion
In conclusion, Grand Central Dispatch (GCD) is a powerful tool for managing concurrency in iOS applications. With its simple yet effective API, GCD makes it easier to create responsive, efficient, and user-friendly apps. By understanding the core concepts, utilizing different queue types, and mastering dispatch techniques, you can harness the full potential of GCD to deliver top-notch user experiences. Whether you’re handling complex data operations or ensuring a smooth UI, GCD has you covered. So, dive into the world of GCD and take your iOS development skills to the next level!
Table of Contents
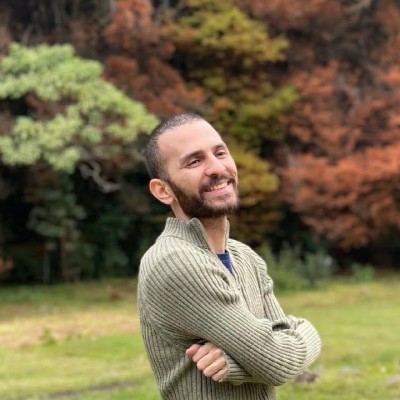
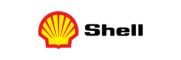