Secure Coding Practices in Java: Protecting Your Application
In today’s digital landscape, ensuring the security of your software applications is of paramount importance. Cyberattacks and data breaches are on the rise, and developers must take proactive measures to protect their applications and the sensitive data they handle. One crucial aspect of this protection is implementing secure coding practices. In this article, we will delve into the world of secure coding practices in Java and explore techniques to safeguard your applications against potential vulnerabilities.
Table of Contents
1. Why Secure Coding Matters
Secure coding is the process of writing software in a way that mitigates security risks and vulnerabilities. It involves considering potential threats and risks throughout the development lifecycle and implementing practices that prevent or minimize these risks. By following secure coding practices, you can reduce the likelihood of security breaches, unauthorized access, and data leaks.
Java is one of the most popular programming languages for building robust and versatile applications. However, like any other language, Java applications are susceptible to security vulnerabilities if not developed with security in mind. By incorporating secure coding practices into your Java development process, you can bolster your application’s defenses against a wide range of potential attacks.
2. Input Validation and Sanitization
Input validation is a fundamental security practice that involves checking and validating user inputs to ensure they adhere to expected formats and ranges. Failing to validate inputs properly can lead to various attacks, such as SQL injection and cross-site scripting (XSS). Consider the following code snippet:
java public void processInput(String input) { if (input != null && !input.isEmpty()) { // Process the input } else { // Handle invalid input } }
In this example, the processInput method checks whether the input is null or empty before proceeding. This simple validation step can prevent potential issues caused by malicious inputs.
3. Avoid Hardcoded Secrets
Hardcoding sensitive information, such as passwords and API keys, directly into your code is a security risk. These secrets can be easily accessed by attackers if they gain access to your application’s codebase. Instead, use environment variables or secure storage mechanisms to manage and access sensitive data. Here’s an example:
java // Avoid this String apiKey = "my_secret_api_key"; // Prefer this String apiKey = System.getenv("API_KEY");
By using environment variables, you keep sensitive information separate from your codebase and make it harder for attackers to access these secrets.
4. Sanitize Data for Output
When displaying user-generated content, such as data from a database or user inputs, sanitize the data to prevent cross-site scripting (XSS) attacks. XSS attacks occur when malicious scripts are injected into web pages and executed by unsuspecting users. To mitigate this risk, use output encoding to ensure that user-generated content is treated as data, not executable code. Here’s an example using Java’s StringEscapeUtils:
java String userInput = "<script>alert('XSS attack');</script>"; String sanitizedOutput = StringEscapeUtils.escapeHtml(userInput); System.out.println(sanitizedOutput); // Output: <script>alert('XSS attack');</script>
5. Implement Proper Authentication and Authorization
Authentication ensures that users are who they claim to be, while authorization defines what actions users are allowed to perform within an application. Utilize strong authentication mechanisms, such as multi-factor authentication (MFA), and implement role-based access control (RBAC) to restrict user actions based on their roles. Consider the following example:
java // Authentication public boolean authenticateUser(String username, String password) { // Validate credentials against a secure database // Implement MFA return true; } // Authorization public boolean hasPermission(User user, String requestedPermission) { // Check user's role and permissions return user.getRoles().stream() .anyMatch(role -> role.getPermissions().contains(requestedPermission)); }
By implementing proper authentication and authorization, you ensure that only authorized users can access and modify sensitive functionalities.
6. Prevent SQL Injection
SQL injection is a common attack where attackers manipulate SQL queries by injecting malicious code. To prevent SQL injection, use prepared statements or parameterized queries when interacting with databases. These techniques ensure that user inputs are treated as data, not executable SQL code. Here’s an example:
java String userInput = "maliciousInput' OR '1'='1"; String query = "SELECT * FROM users WHERE username = ? AND password = ?"; PreparedStatement preparedStatement = connection.prepareStatement(query); preparedStatement.setString(1, userInput); preparedStatement.setString(2, password); ResultSet resultSet = preparedStatement.executeQuery();
Using prepared statements, the user input is automatically treated as a parameter, reducing the risk of SQL injection.
7. Regular Updates and Patch Management
Maintaining the security of your Java applications requires regular updates and patch management. Stay informed about security vulnerabilities in the libraries and frameworks you use, and promptly apply updates and patches. Outdated libraries can expose your application to known vulnerabilities that attackers can exploit.
8. Secure Session Management
Effective session management is essential to prevent unauthorized access to user accounts. Use secure techniques for creating, managing, and terminating user sessions. Implement session timeouts, use secure cookies, and regenerate session IDs after authentication to reduce the risk of session hijacking.
Conclusion
Developing secure Java applications demands a proactive approach to identifying and mitigating potential security vulnerabilities. By integrating secure coding practices into your development process, you can significantly reduce the risk of cyberattacks, data breaches, and unauthorized access. This article covered just a handful of essential practices, but there are many more aspects to explore in the realm of secure coding in Java. Remember that security is an ongoing effort, and staying up-to-date with the latest security trends and vulnerabilities is crucial to keeping your applications and users safe.
Table of Contents
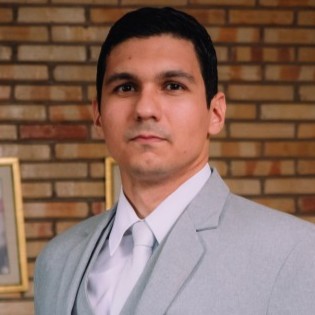
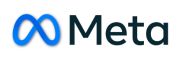