JavaScript Pure Functions: A Paradigm for Predictable Code
When writing code in JavaScript, one of the most significant concerns developers face is maintaining predictability and reducing side effects. Unpredictable code can lead to bugs, making it harder to debug and maintain. To address these challenges, functional programming introduces the concept of “pure functions.” In this blog, we’ll explore what pure functions are, how they work, and how they can revolutionize your JavaScript codebase for the better.
Table of Contents
1. Introduction to Pure Functions
What are Pure Functions?
Pure functions are a fundamental concept in functional programming. A pure function is a function that consistently produces the same output given the same input, without causing any side effects. This means pure functions solely depend on their arguments and don’t rely on external state or data. Consequently, they don’t modify the state of variables or objects outside of their scope.
Characteristics of Pure Functions
Pure functions exhibit several crucial characteristics:
- Deterministic: A pure function will always produce the same output for a given set of inputs. This predictability makes debugging and reasoning about code much easier.
- No Side Effects: Pure functions do not alter variables, objects, or any state outside of their local scope. This eliminates unwanted interactions between different parts of the code.
- Referential Transparency: Since pure functions solely depend on their inputs, they can be replaced with their return value without affecting the program’s behavior. This property is known as referential transparency.
2. Benefits of Pure Functions
Predictability and Determinism
Pure functions bring predictability to your codebase. Given the same inputs, they will always produce the same results. This predictability simplifies debugging and ensures that your program behaves consistently.
Improved Readability
By eliminating side effects and external dependencies, pure functions become self-contained units of logic. This enhances code readability, as you can focus solely on the function’s implementation without worrying about global state or interactions with other parts of the code.
Easier Testing
The testability of pure functions is unparalleled. Since they depend only on their inputs, you can easily test them in isolation. Writing unit tests for pure functions is straightforward, and their deterministic nature makes it easier to identify and fix issues.
3. How Pure Functions Work
No Side Effects
Side effects refer to changes made to state outside of a function’s local scope. These changes can include modifying variables, changing object properties, or updating data in databases. Pure functions eliminate side effects by restricting their interactions to the arguments passed to them.
Consider the following example:
javascript // Impure function let total = 0; function addToTotal(amount) { total += amount; }
In this example, the addToTotal function is impure because it modifies the total variable outside its scope. Now, let’s rewrite this function as a pure function:
javascript // Pure function function addToTotalPure(total, amount) { return total + amount; }
By returning a new value instead of modifying an external variable, the function becomes pure.
Immutability
Another crucial aspect of pure functions is immutability, which means not modifying the function’s arguments within the function. Instead of changing the arguments, pure functions should create and return new objects or values.
javascript // Impure function function updatePersonName(person, newName) { person.name = newName; return person; }
In this example, updatePersonName modifies the person object, making it impure. A pure alternative would look like this:
javascript // Pure function function updatePersonNamePure(person, newName) { return { ...person, name: newName }; }
By creating a new object with the updated name, the original person object remains unchanged.
4. Identifying Impure Functions
Common Impure Functions
Various functions can be impure due to side effects, including:
- Functions that modify global variables or object properties.
- Functions that perform I/O operations, such as reading from or writing to files or databases.
- Functions that generate random numbers without a seed value.
- Functions that use external services or APIs.
Spotting Side Effects
To identify impure functions, look for operations that interact with the environment outside the function’s scope. These operations include:
- Modifying variables outside the function.
- Changing properties of objects passed as arguments.
- Making network requests or interacting with databases.
Understanding impure functions will help you embrace pure functions and functional programming paradigms effectively.
5. Writing Pure Functions
Avoiding External State
To write pure functions, it’s crucial to avoid external state. Avoid using global variables or variables from outer scopes within your functions. Instead, pass all necessary data as function arguments.
javascript // Impure function using external state let counter = 0; function incrementCounter() { counter++; }
This impure function relies on the external counter variable. A pure version would look like this:
javascript // Pure function with no external state function incrementPure(counter) { return counter + 1; }
By explicitly passing the counter as an argument and returning a new value, we maintain purity.
Immutability in Practice
Immutability plays a significant role in writing pure functions. Avoiding mutations ensures that the function doesn’t change the original data, preventing unwanted side effects.
Consider the following example of an impure function that modifies an array:
javascript // Impure function modifying an array function addNumber(numbers, newNumber) { numbers.push(newNumber); return numbers; }
An immutable and pure alternative would be:
javascript // Pure function using immutability function addNumberPure(numbers, newNumber) { return [...numbers, newNumber]; }
The pure version creates a new array with the newNumber, preserving the original numbers array.
Returning Values vs. Modifying Arguments
Pure functions should not modify the arguments they receive. Instead, they should return new values. This keeps the function’s behavior predictable and prevents unexpected side effects.
Consider the following impure function that modifies an object:
javascript // Impure function modifying an object argument function addUser(user, name) { user.name = name; return user; }
The pure version would return a new object with the updated name property:
javascript // Pure function returning a new object function addUserPure(user, name) { return { ...user, name }; }
By adhering to this approach, you can ensure your functions remain pure and predictable.
6. Functional Composition
Combining Pure Functions
Functional composition is a powerful concept in functional programming. It involves combining pure functions to create more complex functions or transformations.
For example, let’s consider two pure functions:
javascript function doubleNumber(number) { return number * 2; } function addFive(number) { return number + 5; }
We can compose these functions to create a new function that doubles a number and then adds five to it:
javascript const doubleAndAddFive = (number) => addFive(doubleNumber(number));
This composition creates a new function without affecting the original functions, demonstrating the benefits of pure functions.
The Compose Function
The compose function simplifies functional composition in JavaScript. It takes multiple functions as arguments and returns a new function that applies them in reverse order.
Let’s implement a simple compose function:
javascript function compose(...fns) { return (x) => fns.reduceRight((acc, fn) => fn(acc), x); }
Now, we can rewrite the previous example using compose:
javascript const doubleAndAddFive = compose(addFive, doubleNumber);
The doubleAndAddFive function now performs the same computation but is more readable and easier to maintain.
7. Pure Functions in Real-World Scenarios
Handling Asynchronous Operations
While pure functions are deterministic and predictable, real-world applications often involve asynchronous operations like API calls and file reading. To deal with such cases, functional programmers often use techniques like functional promises and monads.
Caching Results
Pure functions are suitable for caching results. Since pure functions always produce the same output for the same inputs, you can cache the results to avoid recomputation and improve performance.
8. Pitfalls and Trade-offs
Performance Considerations
Pure functions can be computationally expensive when dealing with large datasets or performing complex computations. In such cases, balancing purity with performance becomes essential.
When Not to Use Pure Functions
Not all parts of an application need to be written as pure functions. For performance-critical or I/O-heavy tasks, impure functions may be more suitable. It’s crucial to strike a balance between purity and practicality.
9. Embracing Pure Functions: A Paradigm Shift
Migrating Existing Code
Migrating an entire codebase to pure functions might be impractical. Instead, start by identifying critical sections of the codebase where predictability and testability are essential and refactor those areas to use pure functions.
Team Collaboration and Best Practices
Introducing pure functions requires collaboration and agreement within a development team. Establishing best practices and educating team members on functional programming concepts are essential for a successful transition.
Conclusion
JavaScript pure functions offer a paradigm shift towards predictable and maintainable code. By adhering to functional programming principles and leveraging the benefits of pure functions, developers can create more reliable and scalable applications. Embracing functional programming and pure functions empowers developers to write code that is easier to reason about, test, and maintain, ultimately leading to higher-quality software.
Table of Contents
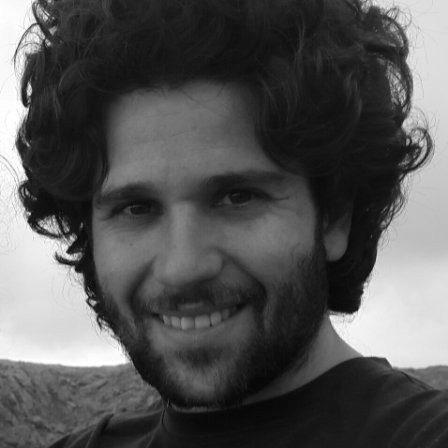
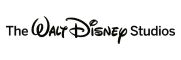