Building Kotlin Android Libraries: Share Your Code with the Community
Creating Android apps often involves reusing code across projects or sharing functionalities with other developers. Building libraries is an excellent way to encapsulate and distribute reusable code, empowering others to leverage your work and contribute to the Android development community. In this blog, we’ll explore the process of building Kotlin Android libraries, along with best practices and tips to foster effective collaboration.
Android development thrives on collaboration and sharing knowledge within the community. When you build a library in Kotlin, you open the doors to contribution and encourage others to build upon your work. In this guide, we’ll walk you through the process of building a Kotlin Android library, covering essential aspects such as defining the library’s goals, writing clean Kotlin code, testing, documentation, versioning, and more.
1. Setting Up the Project
Before diving into writing code, setting up the project correctly is crucial. You have a few options to get started:
Android Studio Template: Android Studio provides a library template that streamlines the process. Go to File > New > New Module and select Android Library.
Manual Setup: If you prefer more control, create a new Android module and set its plugin to com.android.library.
2. Defining the Library
2.1. Library Goals and Features
Clearly define the goals and scope of your library. Identify the specific functionalities it will provide and the problems it aims to solve. Maintaining a clear vision will help you focus on essential features and avoid feature creep.
2.2. Designing a Clean API
A well-designed API is crucial for a successful library. Strive for simplicity, consistency, and ease of use. Hide implementation details and expose only the necessary functions. Use clear and meaningful names for classes and methods.
3. Writing the Kotlin Code
3.1. Kotlin Language Overview
Kotlin is a powerful and expressive language that brings numerous features to Android development. Familiarize yourself with Kotlin’s key features such as null safety, extension functions, data classes, and coroutines.
3.2. Common Kotlin Features for Libraries
- Data Classes: Use data classes to model data structures effectively.
- Extensions: Leverage extension functions to add functionality to existing classes without subclassing.
- Coroutines: Consider using coroutines to write asynchronous and concurrent code more elegantly.
- Companion Objects: Use companion objects to define static methods and constants.
kotlin // Example of a data class and an extension function data class User(val name: String, val age: Int) fun User.isAdult() = age >= 18
4. Unit Testing and Test Coverage
4.1. Importance of Testing in Libraries
Unit testing is essential to ensure the reliability of your library. Write unit tests for each function and edge case to catch bugs early and provide a seamless experience to the users.
4.2. Writing Unit Tests with Kotlin and JUnit
Kotlin works seamlessly with JUnit for unit testing. Ensure you use dependency injection to facilitate mocking and keep tests isolated.
kotlin class MathUtils { fun add(a: Int, b: Int): Int = a + b } class MathUtilsTest { @Test fun testAddition() { val mathUtils = MathUtils() assertEquals(5, mathUtils.add(2, 3)) } }
4.3. Measuring Test Coverage with Jacoco
Use Jacoco to measure your test coverage and identify areas that lack test cases. Aim for high test coverage to ensure robustness.
5. Documentation
Writing Clear and Comprehensive Docs
Proper documentation is crucial for your library’s adoption. Include usage examples, explanations, and any potential gotchas. Keep the documentation up-to-date with the codebase.
5.1. Generating API Documentation with Dokka
Dokka is a documentation engine for Kotlin that generates API documentation from code comments. Integrating Dokka into your build process ensures that your documentation stays in sync with your code.
6. Versioning and Dependency Management
6.1. Semantic Versioning
Follow semantic versioning to ensure compatibility and provide users with a clear understanding of the changes between releases.
6.2. Publishing to Maven Central
Make your library accessible to others by publishing it to Maven Central or other package repositories. This allows developers to easily include your library as a dependency in their projects.
7. Proguard and Code Obfuscation
Protecting Library Code with Proguard
If your library contains sensitive code or assets, consider using Proguard to obfuscate and shrink your code, making it harder to reverse engineer.
8. Sample App for Demonstration
Creating a Sample App for the Library
Include a sample app in your library to demonstrate its capabilities and provide users with a hands-on experience.
9. Collaboration and Contribution
Hosting the Project on GitHub
GitHub is the go-to platform for hosting open-source projects. Share your library’s code and invite others to collaborate.
Code Review and Pull Requests
Encourage contributions from the community and conduct code reviews to maintain code quality. Be responsive to pull requests and foster a welcoming environment for contributors.
Conclusion
Building Kotlin Android libraries is a rewarding way to share your expertise and contribute to the vibrant Android development community. By following best practices, writing clean code, and fostering collaboration, you can create powerful and widely adopted libraries that make a positive impact on Android app development. So go ahead, start building, and let your code shine in the hands of developers worldwide!
Table of Contents
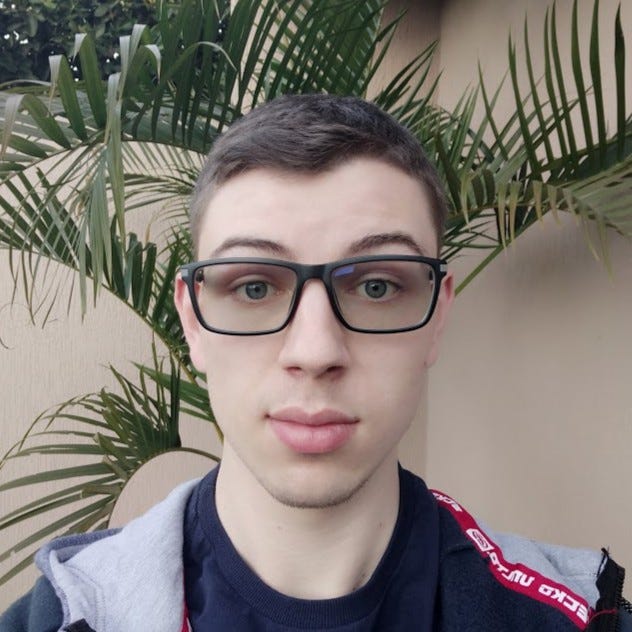
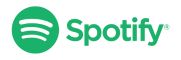