Transitioning to Kotlin DSL: A Guide to Cleaner, More Intuitive Build Scripts
The power of Gradle as a build system for Android and JVM-based projects is well known. But when it comes to writing and maintaining build scripts, the traditional Groovy-based scripts can be a bit cumbersome, especially for those who primarily code in Kotlin. Enter Kotlin DSL for Gradle—a mechanism to use Kotlin to express your build scripts, making them more concise, type-safe, and easily maintainable.
In this post, we’ll dive into the reasons to choose Kotlin DSL over Groovy for your Gradle scripts, followed by hands-on examples demonstrating its simplicity and power.
1. Why Choose Kotlin DSL for Gradle?
- Type Safety: Being a statically-typed language, Kotlin offers compile-time checks. This means less runtime errors and more feedback during the build script authoring.
- Improved IDE Support: With Kotlin DSL, you get better auto-completion, navigation, refactoring, and more, leveraging the strength of IntelliJ IDEA.
- Kotlin Expertise: If you’re already a Kotlin developer, using Kotlin DSL provides consistency across your project, from app code to build scripts.
2. Getting Started with Kotlin DSL
Start by renaming your `build.gradle` to `build.gradle.kts`. Gradle will recognize the `kts` extension as a signal to use the Kotlin DSL parser.
2.1 Basic Examples
- Applying Plugins
Groovy:
```groovy apply plugin: 'com.android.application' apply plugin: 'kotlin-android' ```
Kotlin DSL:
```kotlin plugins { id("com.android.application") kotlin("android") } ```
- Defining Dependencies:
Groovy:
```groovy dependencies { implementation 'org.jetbrains.kotlin:kotlin-stdlib:1.5.0' testImplementation 'junit:junit:4.13.2' } ```
Kotlin DSL:
```kotlin dependencies { implementation("org.jetbrains.kotlin:kotlin-stdlib:1.5.0") testImplementation("junit:junit:4.13.2") } ```
2.2 Advanced Examples
- Configuring Android Builds:
Groovy:
```groovy android { compileSdkVersion 30 defaultConfig { applicationId "com.example.myapp" minSdkVersion 21 targetSdkVersion 30 } } ```
Kotlin DSL:
```kotlin android { compileSdkVersion(30) defaultConfig { applicationId = "com.example.myapp" minSdkVersion(21) targetSdkVersion(30) } } ```
- Custom Tasks:
Groovy:
```groovy task myTask(type: Copy) { from 'src/main/resources' into 'build/resources' } ```
Kotlin DSL:
```kotlin tasks.register<Copy>("myTask") { from("src/main/resources") into("build/resources") } ```
3. Using Extension Functions
One of the powerful features of Kotlin is extension functions. This can be super handy to make your build scripts cleaner.
Suppose you find yourself repeating the same configuration for multiple tasks. Instead of duplicating the configuration, you can define an extension function:
```kotlin fun TaskContainer.customJavaCompile() { withType<JavaCompile>().configureEach { sourceCompatibility = JavaVersion.VERSION_11 targetCompatibility = JavaVersion.VERSION_11 } } ```
And then use it in your build script:
```kotlin tasks.customJavaCompile() ```
Conclusion
Kotlin DSL for Gradle not only simplifies your build scripts but also offers a more powerful and expressive way to define them. By leveraging Kotlin’s type safety and IDE support, developers can get immediate feedback, leading to fewer errors and faster iterations. Whether you are starting a new project or maintaining an old one, consider making the switch to Kotlin DSL for a cleaner, more consistent, and intuitive build process.
Table of Contents
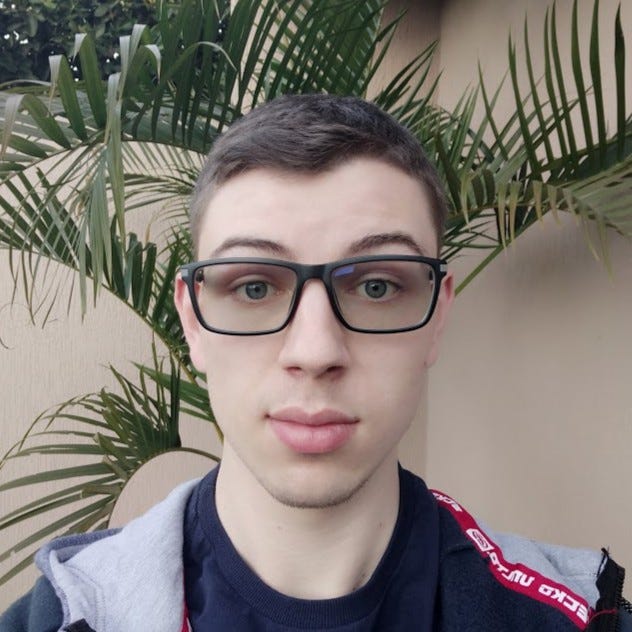
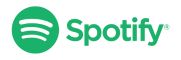