What is a lambda with receiver in Kotlin?
A lambda with receiver, also known as a “receiver lambda,” is a unique construct in Kotlin designed to facilitate concise and expressive extension functions for specific types. With a lambda with receiver, developers can augment the functionality of a particular type by providing a receiver object directly within the lambda expression.
The distinguishing characteristic of a lambda with receiver lies in its ability to grant direct access to the properties and functions of the receiver object within the lambda body. This capability mimics the behavior of member functions within a class, which can access the class’s properties and methods seamlessly.
By leveraging a lambda with receiver, developers can extend the behavior of existing types in Kotlin without the need for wrapper classes or verbose syntax. This approach promotes cleaner and more readable code by encapsulating related functionality within the context of the receiver object.
For example, consider a scenario where you want to add additional functionality to the String class in Kotlin. Instead of creating a separate utility class or cluttering the global namespace with standalone functions, you can define an extension function using a lambda with receiver:
kotlin // Define an extension function on String using a lambda with receiver fun String.customExtension(block: String.() -> Unit): String { block() // Invoke the lambda with receiver on the current string instance return this // Return the modified string } // Usage of the extension function val modifiedString = "Hello".customExtension { println(this) // Access the properties and methods of the receiver object // Additional modifications or operations on the string }
In this example, the customExtension function extends the functionality of the String class by accepting a lambda with receiver as a parameter. Within the lambda body, the this keyword refers to the receiver object, allowing direct access to the properties and methods of the String instance.
Lambda expressions with receivers are particularly useful for building domain-specific languages (DSLs) and creating fluent APIs in Kotlin. They enable developers to define domain-specific syntax and chain method calls in a concise and expressive manner, enhancing the readability and maintainability of the codebase.
A lambda with receiver is a powerful feature in Kotlin that empowers developers to extend the functionality of existing types in a clean and expressive way. By providing direct access to the receiver object’s members within the lambda body, this construct promotes code encapsulation, reusability, and readability in Kotlin projects.
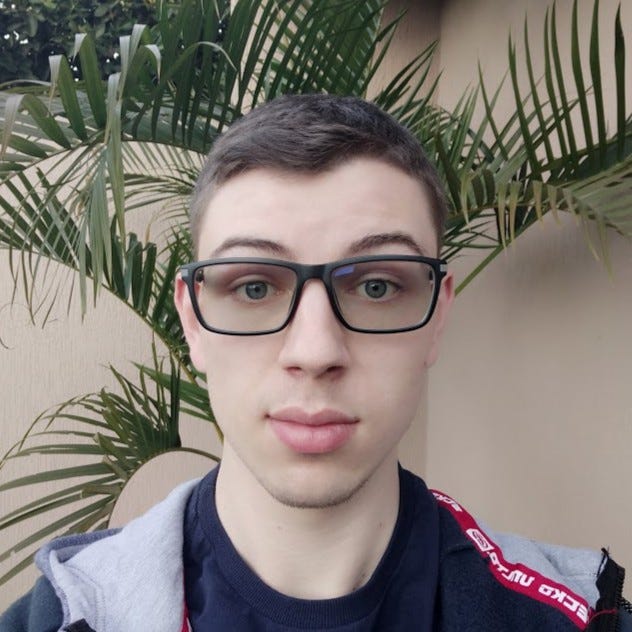
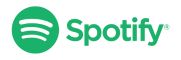