Manipulating Data with Kotlin Collections: An In-depth Guide on Lists, Maps, and Sets
In the realm of programming, data manipulation is an inescapable reality. No matter which language we utilize, handling data comes to the forefront. For companies looking to hire Kotlin developers, understanding these concepts is especially important. Kotlin, a language gaining significant traction in the developer community, has collections as the go-to solution for managing data, featuring an extensive set of features. In this blog post, we’ll delve into three significant types of Kotlin collections: Lists, Maps, and Sets. These are key concepts that any competent Kotlin developer, such as those you might hire, should be well versed in.
1. Lists in Kotlin
A list is an ordered collection, and it is analogous to an array. In Kotlin, you can create a list by using the `listOf()` function. It also provides `mutableListOf()` for creating mutable lists. Let’s explore some examples:
```kotlin val immutableList = listOf(1, 2, 3, 4, 5) val mutableList = mutableListOf(1, 2, 3, 4, 5) ```
The `immutableList` can’t be changed once initialized. It can’t accept any modifications such as adding or removing elements. On the other hand, `mutableList` allows changes.
Here’s how to add and remove elements from a mutable list:
```kotlin mutableList.add(6) mutableList.removeAt(2) ```
You can also manipulate list elements by using various methods provided by Kotlin, such as `first()`, `last()`, `filter()`, `map()`, etc.
```kotlin println(mutableList.first()) // prints 1 println(mutableList.last()) // prints 6 val evenNumbers = mutableList.filter { it % 2 == 0 } println(evenNumbers) // prints [2, 4, 6] val squares = mutableList.map { it * it } println(squares) // prints [1, 4, 16, 36] ```
2. Maps in Kotlin
Maps, also known as dictionaries or associative arrays in other languages, store key-value pairs. A map can’t have duplicate keys, but it can have duplicate values. You can create a map in Kotlin using the `mapOf()` function for an immutable map and `mutableMapOf()` for a mutable map.
```kotlin val immutableMap = mapOf(1 to "one", 2 to "two", 3 to "three") val mutableMap = mutableMapOf(1 to "one", 2 to "two", 3 to "three") ```
Adding and removing elements in a mutable map is straightforward:
```kotlin mutableMap.put(4, "four") mutableMap.remove(2) ```
You can access and manipulate elements of the map using keys and various methods:
```kotlin println(mutableMap[1]) // prints "one" println(mutableMap.keys) // prints [1, 3, 4] println(mutableMap.values) // prints ["one", "three", "four"] ```
Kotlin provides a multitude of useful functions for maps, such as `filter()`, `map()`, `any()`, etc.
```kotlin val filteredMap = mutableMap.filter { (key, value) -> key % 2 == 0 } println(filteredMap) // prints {4="four"} val mappedMap = mutableMap.map { (key, value) -> "$key => $value" } println(mappedMap) // prints ["1 => one", "3 => three", "4 => four"] val isAnyEvenKey = mutableMap.any { (key, _) -> key % 2 == 0 } println(isAnyEvenKey) // prints true ```
3. Sets in Kotlin
A set is a collection of unique elements, meaning it doesn’t contain any duplicate elements. Like lists and maps, we have `setOf()` for creating an immutable set and `mutableSetOf()` for a mutable set.
```kotlin val immutableSet = setOf(1, 2, 3, 3, 4, 5, 5) val mutableSet = mutableSetOf(1, 2, 3, 3, 4, 5, 5) ```
In the above example, even though we tried to add duplicate elements, the set will only contain unique elements. Thus the output will be `[1, 2, 3, 4, 5]` for both.
You can add and remove elements in a mutable set:
```kotlin mutableSet.add(6) mutableSet.remove(2) ```
Sets offer various operations like union, intersection, and difference:
```kotlin val setA = setOf(1, 2, 3) val setB = setOf(2, 3, 4) println(setA union setB) // prints [1, 2, 3, 4] println(setA intersect setB) // prints [2, 3] println(setA subtract setB) // prints [1] ```
Additionally, like Lists and Maps, Kotlin provides functions for sets such as `filter()`, `map()`, `any()`, etc.
```kotlin val evenNumbersSet = mutableSet.filter { it % 2 == 0 } println(evenNumbersSet) // prints [4, 6] val squaresSet = mutableSet.map { it * it } println(squaresSet) // prints [1, 9, 16, 25, 36] val isAnyEvenNumber = mutableSet.any { it % 2 == 0 } println(isAnyEvenNumber) // prints true ```
Conclusion
Kotlin Collections are an integral part of the Kotlin Standard Library. Lists, Maps, and Sets are fundamental collection types that every Kotlin developer, including those you might hire as Kotlin developers, must be familiar with. With the power to create, manipulate, and traverse these collections, Kotlin provides a robust mechanism to handle data.
Understanding these collections not only deepens your toolkit and enhances your Kotlin programming capabilities, but it also helps you assess the skills of Kotlin developers you plan to hire. By harnessing these concepts, you’re a step closer to mastering Kotlin and making more informed decisions in your hiring process.
Table of Contents
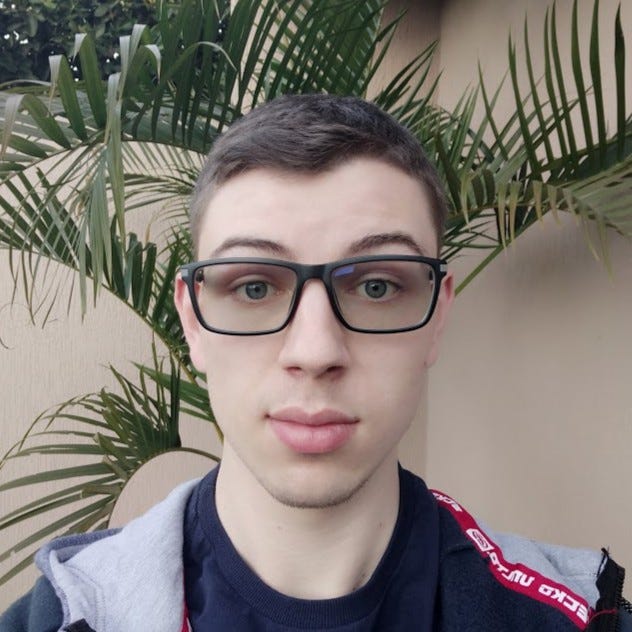
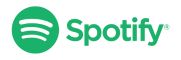