Kotlin and Reactive Programming: Exploring RxJava and Kotlin Flow
Reactive Programming has gained significant popularity in recent years due to its ability to handle asynchronous and event-driven programming paradigms effectively. In this blog post, we will explore how to harness the power of Reactive Programming in Kotlin using two popular libraries: RxJava and Kotlin Flow. We will dive into the key concepts of Reactive Programming and provide code samples to help you get started.
1. What is Reactive Programming?
Reactive Programming is a programming paradigm that allows developers to build applications that react to changes in data streams and handle asynchronous events efficiently. It emphasizes declarative and event-driven programming models, making it easier to manage complex asynchronous operations.
2. Introduction to RxJava
RxJava is a popular Reactive Programming library for the Java Virtual Machine (JVM) that provides powerful abstractions for working with asynchronous data streams. It follows the ReactiveX specification and offers a rich set of operators and utilities.
2.1 Installation and Setup
To use RxJava in a Kotlin project, you need to add the RxJava dependency to your project’s build configuration. Here’s an example using Gradle:
kotlin dependencies { implementation 'io.reactivex.rxjava3:rxjava:3.1.2' }
2.2 Observables and Observers
At the core of RxJava are two main components: Observables and Observers. Observables emit items or sequences of items over time, and Observers consume these items and react to them. Here’s a simple example:
kotlin val observable: Observable<String> = Observable.just("Hello", "World") observable.subscribe { value -> println(value) }
In this example, the Observable.just() method emits two strings, “Hello” and “World”. The subscribe method sets up an Observer that receives and prints each emitted value.
2.3 Operators and Transformations
RxJava provides a vast array of operators and transformations that allow you to manipulate and transform data streams efficiently. For instance, the map operator transforms each item emitted by an Observable by applying a function to it. Here’s an example:
kotlin val observable: Observable<Int> = Observable.just(1, 2, 3) observable.map { it * 2 } .subscribe { value -> println(value) }
This code multiplies each emitted integer by 2 and prints the transformed values.
2.4 Error Handling
RxJava provides various error handling mechanisms to handle exceptional cases gracefully. The onError method allows you to handle errors emitted by an Observable. Here’s an example:
kotlin val observable: Observable<Int> = Observable.just(1, 2, 3) observable.map { 10 / it } .subscribe( { value -> println(value) }, { error -> println("Error: $error") } )
In this example, the division by zero will cause an exception. The second lambda passed to subscribe handles the error and prints an error message.
2.5 Threading and Schedulers
RxJava provides schedulers to control the threading behavior of Observable streams. You can use schedulers to specify which thread should execute the Observable’s logic, subscribe on a background thread, or observe on the main thread. Here’s an example:
kotlin Observable.just(1, 2, 3) .subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) .subscribe { value -> println(value) }
This code schedules the Observable’s emission on an IO thread and the Observer’s consumption on the main thread.
3. Introduction to Kotlin Flow
Kotlin Flow is a reactive streams library introduced by JetBrains, the creators of Kotlin. It is designed to provide a more idiomatic and efficient way to work with asynchronous streams in Kotlin.
3.1 Installation and Setup
To use Kotlin Flow in your Kotlin project, you need to add the appropriate dependency. Here’s an example using Gradle:
kotlin dependencies { implementation "org.jetbrains.kotlinx:kotlinx-coroutines-core:1.5.1" implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:1.5.1" implementation "org.jetbrains.kotlinx:kotlinx-coroutines-reactive:1.5.1" }
3.2 Flow Builders and Operators
Kotlin Flow offers a variety of operators and builders to create, transform, and combine streams of data. You can use operators like map, filter, and reduce to manipulate the emitted values. Here’s an example:
kotlin val flow: Flow<Int> = flowOf(1, 2, 3) flow.map { it * 2 } .collect { value -> println(value) }
This code creates a simple flow of integers, multiplies each value by 2, and collects the transformed values.
3.3 Error Handling
Kotlin Flow provides built-in error handling mechanisms through exception handling operators. The catch operator allows you to handle exceptions emitted by a Flow. Here’s an example:
kotlin val flow: Flow<Int> = flowOf(1, 2, 0, 3) flow.map { 10 / it } .catch { error -> println("Error: $error") } .collect { value -> println(value) }
In this example, the division by zero will cause an exception. The catch operator handles the exception and prints an error message.
3.4 Threading and Contexts
Kotlin Flow seamlessly integrates with Kotlin Coroutines, allowing you to control the execution context of flows. You can use CoroutineContext to specify which thread should execute the flow’s logic and apply various context operators. Here’s an example:
kotlin flowOf(1, 2, 3) .flowOn(Dispatchers.IO) .collect { value -> println(value) }
This code schedules the flow’s execution on an IO dispatcher.
4. Comparing RxJava and Kotlin Flow
4.1 Syntax and Usage
RxJava and Kotlin Flow have similar concepts and offer similar functionality. However, Kotlin Flow leverages the language features of Kotlin, making the syntax more concise and idiomatic.
4.2 Interoperability
RxJava has been around for a longer time and has a larger ecosystem. It provides better interoperability with existing Java libraries. On the other hand, Kotlin Flow integrates seamlessly with Kotlin Coroutines, making it a natural choice for Kotlin projects.
4.3 Performance Considerations
Both RxJava and Kotlin Flow are designed to be efficient and provide excellent performance. However, Kotlin Flow’s integration with Kotlin Coroutines can offer better performance in Kotlin-based projects.
Conclusion
Reactive Programming is a powerful paradigm for handling asynchronous and event-driven programming in Kotlin. In this blog post, we explored two popular libraries, RxJava and Kotlin Flow, and learned how to leverage their capabilities. We covered the key concepts, installation, usage, and error handling, along with a comparison of the two libraries. By mastering Reactive Programming with RxJava and Kotlin Flow, you can build more robust and responsive Kotlin applications. So, go ahead and dive into the world of Reactive Programming in Kotlin!
Table of Contents
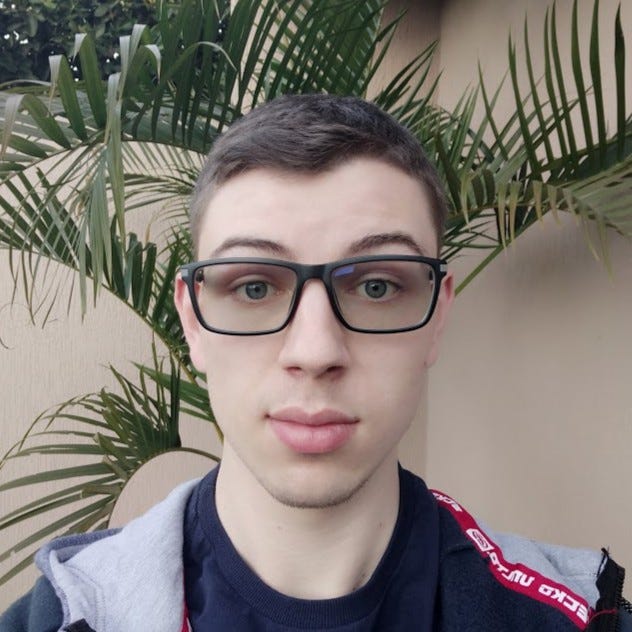
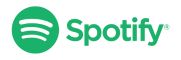