Laravel Queues with Redis: Scaling Task Processing
In the world of web applications, efficient task processing is a crucial aspect of delivering a seamless user experience. From sending emails to generating reports, these tasks can sometimes be time-consuming and resource-intensive. Laravel, a popular PHP framework, offers a robust solution to this challenge through its queuing system. When coupled with Redis, a powerful in-memory data store, Laravel’s queues become even more potent, enabling developers to scale task processing effortlessly.
Table of Contents
1. Understanding Laravel Queues
Before diving into the specifics of Redis integration, let’s briefly explore what Laravel Queues are and why they’re beneficial.
1.1. What are Queues?
In a web application, there are tasks that need to be executed in the background, without delaying the user’s interaction. These tasks could include sending notifications, processing images, or handling payments. Queues provide a mechanism for managing and deferring the execution of these tasks.
1.2. Benefits of Using Queues
- Improved Responsiveness: By moving time-consuming tasks to the background, your application remains responsive and provides a seamless user experience.
- Scalability: Queues allow you to distribute tasks across multiple workers, enabling horizontal scaling and better resource utilization.
- Fault Tolerance: In cases of failures, the tasks in the queue can be retried automatically, ensuring that important processes are not lost.
- Priority Handling: Some tasks might be more critical than others. Queues can prioritize tasks based on their importance.
2. Integrating Redis with Laravel Queues
Laravel’s default queue driver is a synchronous system, where jobs are executed immediately as they are dispatched. While this is suitable for small tasks, it’s not optimal for larger tasks or tasks that require a lot of time.
Redis, on the other hand, is an advanced in-memory data structure store known for its speed and versatility. It serves as an excellent backend for Laravel’s queue system, making task processing faster and more scalable.
2.1. Setting Up Redis
Before you can start using Redis for queuing, you need to set up a Redis server and configure Laravel to use it. Here’s how:
- Install Redis: If you haven’t already, install Redis on your server or use a managed Redis service.
- Configure Laravel: In the .env file of your Laravel application, set the QUEUE_CONNECTION variable to redis.
- Provide Redis Configuration: In the .env file, specify the Redis server’s host, port, and other necessary credentials.
2.2. Creating and Dispatching Jobs
Once Redis is set up, you can create jobs that represent the tasks you want to perform. Jobs are PHP classes that encapsulate the task logic. Here’s a simple example of creating and dispatching a job:
php php artisan make:job ProcessImage
This command creates a new job class named ProcessImage in the app/Jobs directory. You can then define the task logic within the handle method of the job class:
php class ProcessImage implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; public function handle() { // Task logic for processing the image } }
To dispatch the job, you can use the dispatch method:
php ProcessImage::dispatch();
2.3. Running Queue Workers
Now that your jobs are ready, you need workers to process them. Workers are processes that pull jobs from the queue and execute them. Run the following command to start a queue worker:
php php artisan queue:work
By default, the worker will use the redis connection as specified in your .env file.
3. Scaling with Redis and Laravel Queues
The true power of using Redis with Laravel Queues lies in the scalability it brings to your application’s task processing. Here’s how this combination helps you scale effectively:
3.1. Load Distribution
As your application grows, the number of tasks that need to be processed can increase significantly. By using Redis-backed queues, you can easily distribute these tasks across multiple worker processes. This load distribution prevents a single worker from becoming overwhelmed, ensuring tasks are handled efficiently.
3.2. Horizontal Scaling
Redis allows you to set up multiple queues and workers, each dedicated to specific types of tasks. For instance, you could have separate queues for email sending, image processing, and more. This way, you can scale each aspect of your application independently based on its demands.
3.3. High Throughput
Redis’s in-memory nature contributes to its lightning-fast performance. When combined with Laravel’s queues, it results in high throughput for task processing. This is especially beneficial when dealing with large volumes of tasks or tasks that involve complex computations.
3.4. Fault Tolerance and Reliability
Redis offers built-in mechanisms for handling failures and ensuring data integrity. If a worker process fails during task execution, the job can be retried without data loss. This resilience is crucial for maintaining reliable task processing, especially in critical scenarios.
4. Best Practices
While Laravel Queues with Redis offer impressive scalability, it’s essential to follow best practices to make the most of this combination:
4.1. Optimize Job Execution Time
Although Redis and Laravel Queues can handle substantial workloads, it’s still advisable to optimize your job execution time. Break down complex tasks into smaller sub-tasks and use techniques like parallel processing to speed up processing.
4.2. Monitor and Manage Queues
Regularly monitor your queues and workers to ensure they are performing as expected. Laravel provides tools like the Horizon dashboard to visualize and manage your queues effectively.
4.3. Choose the Right Queue Configuration
Laravel Queues allow you to configure the number of worker processes, the maximum number of attempts for a job, and more. Choose these configurations wisely based on your application’s requirements and available resources.
Conclusion
Laravel Queues with Redis offer a powerful solution for scaling task processing in your web applications. By leveraging the speed and versatility of Redis, coupled with Laravel’s intuitive queuing system, you can ensure efficient background task execution, improved application responsiveness, and seamless scalability as your application grows. Whether you’re sending emails or processing complex data, integrating Redis with Laravel Queues is a step towards building a robust and high-performance application architecture.
Table of Contents
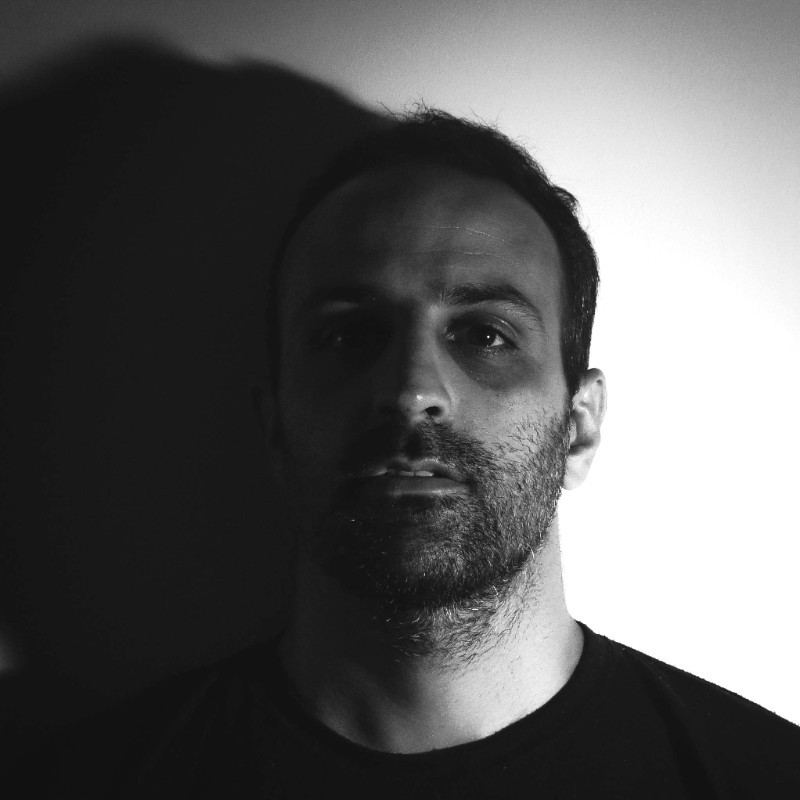
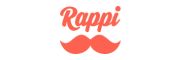