Interview Guide to Hire ASP.NET MVC Developers
ASP.NET MVC, known for its powerful framework and flexibility, remains a popular choice for building dynamic web applications. Hiring proficient ASP.NET MVC developers is crucial for creating robust and scalable software solutions. This guide serves as your roadmap through the hiring process, equipping you with the necessary strategies and interview questions to identify the ideal ASP.NET MVC developer for your team.
1. How to Hire ASP.NET MVC Developers
Embarking on the journey to hire MVC developers? Follow these steps for a successful process:
- Job Requirements: Clearly define specific job prerequisites, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ ASP.NET MVC proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of ASP.NET MVC Developers to Look For
When evaluating ASP.NET MVC developers, ensure they possess these core skills: Learn more over at our MVC developer job description guide here.
- ASP.NET MVC Proficiency: A deep understanding of the ASP.NET MVC framework, including its architecture and components.
- C# Knowledge: Strong knowledge of C#, the primary language used in ASP.NET MVC development.
- Front-End Skills: Proficiency in HTML, CSS, JavaScript, and popular frameworks like Angular or React.
- Database Management: Experience with SQL Server, including database design, optimization, and querying.
- Web Services: Familiarity with building and consuming RESTful APIs and web services.
- Problem-Solving Abilities: Aptitude for identifying challenges and devising efficient solutions.
- Software Development: Proficiency in software development methodologies, version control, and collaborative tools.
- Testing and Debugging: Ability to write unit tests and debug code to ensure software reliability.
3. Overview of the ASP.NET MVC Developer Hiring Process
Here’s an overview of the ASP.NET MVC developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create captivating job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting ASP.NET MVC Developer Interview Questions
Develop a comprehensive set of interview questions covering ASP.NET MVC intricacies, problem-solving aptitude, and relevant technologies. Find out more on the hiring process on our MVC developer hiring guide here.
4. Sample ASP.NET MVC Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ ASP.NET MVC skills:
Q1. Explain the MVC architecture in ASP.NET MVC. How does it work?
A: MVC stands for Model-View-Controller. The architecture separates the application into three main components: Model (data), View (user interface), and Controller (business logic). This separation facilitates modular development and ease of maintenance.
Q2. What is the Razor View Engine in ASP.NET MVC?
A: Razor is a markup syntax for embedding server-based code into web pages. It provides a streamlined way to create dynamic web content using C# or VB.NET.
Q3. How do you handle exceptions in ASP.NET MVC?
A: Exceptions in ASP.NET MVC can be handled using try-catch blocks, custom error pages, and global filters like HandleErrorAttribute
. Proper exception handling ensures a robust and user-friendly application.
Q4. Implement a basic CRUD operation in ASP.NET MVC.
A: Create a controller with methods for Create, Read, Update, and Delete operations. Use Entity Framework for database interactions. Here’s an example for creating an entry:
public class ProductController : Controller { private readonly ApplicationDbContext _context; public ProductController() { _context = new ApplicationDbContext(); } [HttpPost] public ActionResult Create(Product product) { if (ModelState.IsValid) { _context.Products.Add(product); _context.SaveChanges(); return RedirectToAction("Index"); } return View(product); } }
Q5. Explain the purpose of dependency injection in ASP.NET MVC.
A: Dependency injection (DI) is a design pattern used to achieve Inversion of Control (IoC) between classes and their dependencies. DI allows for better modularity, testability, and maintainability of code by decoupling dependencies from the classes that use them.
Q6. How do you implement authentication and authorization in ASP.NET MVC?
A: Authentication can be implemented using ASP.NET Identity, OAuth, or other frameworks. Authorization is typically managed through attributes like [Authorize]
on controllers or actions to restrict access based on user roles.
Q7. Explain the MVC architecture in ASP.NET MVC. How does it work?
A: MVC stands for Model-View-Controller. It separates an application into three main components:
- Model: Represents the application’s data and business logic.
- View: Represents the UI components that display the data.
- Controller: Handles user input and interactions, updating the Model and View accordingly. The separation of concerns facilitates modular development and ease of maintenance.
Q8. How do you create a simple controller in ASP.NET MVC?
A: A simple controller can be created by inheriting from the Controller
class and defining actions as methods.
public class HomeController : Controller { public ActionResult Index() { return View(); } public ActionResult About() { ViewBag.Message = "Your application description page."; return View(); } }
Q9. What is the Razor View Engine in ASP.NET MVC? Provide an example.
A: Razor is a markup syntax for embedding server-based code into web pages. It allows for clean and readable syntax by using @
to switch between HTML and C#.
@{ ViewBag.Title = "Home Page"; } <h2>@ViewBag.Title</h2> <p>Welcome to ASP.NET MVC with Razor!</p>
Q10. How do you handle form submissions in ASP.NET MVC?
A: Form submissions are handled by using HTML forms and controller actions to process the submitted data.
// View @model YourNamespace.Models.ContactFormModel @using (Html.BeginForm("SubmitForm", "Home", FormMethod.Post)) { @Html.LabelFor(m => m.Name) @Html.TextBoxFor(m => m.Name) @Html.LabelFor(m => m.Email) @Html.TextBoxFor(m => m.Email) <input type="submit" value="Submit" /> } // Controller [HttpPost] public ActionResult SubmitForm(ContactFormModel model) { if (ModelState.IsValid) { // Process form data return RedirectToAction("Success"); } return View(model); }
Q11. Explain the concept of routing in ASP.NET MVC. How do you define a custom route?
A: Routing in ASP.NET MVC is the process of mapping URLs to controller actions. Custom routes can be defined in the RouteConfig
class.
public class RouteConfig { public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); routes.MapRoute( name: "CustomRoute", url: "Products/{action}/{id}", defaults: new { controller = "Products", action = "Index", id = UrlParameter.Optional } ); routes.MapRoute( name: "Default", url: "{controller}/{action}/{id}", defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional } ); } }
Q12. How do you implement dependency injection in ASP.NET MVC? Provide an example.
A: Dependency Injection (DI) is implemented using an IoC (Inversion of Control) container like Unity, Ninject, or Autofac. Here’s an example using Unity:
// Install Unity via NuGet public class UnityConfig { public static void RegisterComponents() { var container = new UnityContainer(); // Register types container.RegisterType<IProductService, ProductService>(); DependencyResolver.SetResolver(new UnityDependencyResolver(container)); } } // In Global.asax protected void Application_Start() { UnityConfig.RegisterComponents(); AreaRegistration.RegisterAllAreas(); RouteConfig.RegisterRoutes(RouteTable.Routes); } // Usage in Controller public class ProductsController : Controller { private readonly IProductService _productService; public ProductsController(IProductService productService) { _productService = productService; } public ActionResult Index() { var products = _productService.GetAllProducts(); return View(products); } }
Q13. How do you perform model validation in ASP.NET MVC?
A: Model validation is performed using data annotations and the ModelState
property.
// Model public class UserModel { [Required] [StringLength(100)] public string Name { get; set; } [Required] [EmailAddress] public string Email { get; set; } } // Controller [HttpPost] public ActionResult Register(UserModel model) { if (ModelState.IsValid) { // Process the model return RedirectToAction("Success"); } return View(model); } // View @model YourNamespace.Models.UserModel @using (Html.BeginForm()) { @Html.LabelFor(m => m.Name) @Html.TextBoxFor(m => m.Name) @Html.ValidationMessageFor(m => m.Name) @Html.LabelFor(m => m.Email) @Html.TextBoxFor(m => m.Email) @Html.ValidationMessageFor(m => m.Email) <input type="submit" value="Register" /> } @section Scripts { @Scripts.Render("~/bundles/jqueryval") }
Q14. How do you implement authorization in ASP.NET MVC?
A: Authorization is implemented using the [Authorize]
attribute on controllers or actions.
// Applying to a controller [Authorize] public class AdminController : Controller { public ActionResult Index() { return View(); } } // Applying to specific actions public class HomeController : Controller { [Authorize(Roles = "Admin")] public ActionResult AdminOnly() { return View(); } [Authorize(Users = "specificuser@example.com")] public ActionResult SpecificUserOnly() { return View(); } }
Q15. How do you use Entity Framework in ASP.NET MVC for data access?
A: Entity Framework is used for data access by creating a DbContext class and interacting with the database using LINQ queries.
// Model public class Product { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } } // DbContext public class ApplicationDbContext : DbContext { public DbSet<Product> Products { get; set; } } // Controller public class ProductsController : Controller { private readonly ApplicationDbContext _context; public ProductsController() { _context = new ApplicationDbContext(); } public ActionResult Index() { var products = _context.Products.ToList(); return View(products); } public ActionResult Details(int id) { var product = _context.Products.Find(id); if (product == null) { return HttpNotFound(); } return View(product); } }
Q16. How do you implement AJAX calls in ASP.NET MVC? Provide an example.
A: AJAX calls are implemented using JavaScript/jQuery to send asynchronous requests to controller actions.
// JavaScript/jQuery code in View $(document).ready(function () { $("#btnGetData").click(function () { $.ajax({ url: '/Home/GetData', type: 'GET', success: function (data) { $("#dataContainer").html(data); }, error: function () { alert('Error retrieving data'); } }); }); });
5. Hiring ASP.NET MVC Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected ASP.NET MVC developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional ASP.NET MVC developers, ensuring your team possesses the skills required to build remarkable software solutions.
6. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess ASP.NET MVC developers comprehensively. Whether you’re developing dynamic web applications or complex enterprise solutions, securing the right ASP.NET MVC developers for your team is pivotal to the success of your projects.
Table of Contents
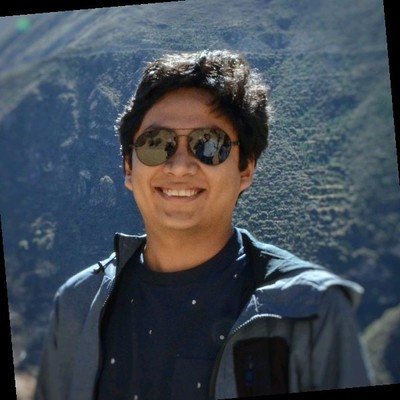
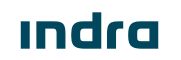