Unleashing the Potential of React with NEXT.js: An In-Depth Exploration
When Facebook introduced React in 2013, it forever altered the landscape of front-end development by offering a powerful, component-based approach to building user interfaces. As a result, many companies are looking to hire NEXT.js developers, who have the skills to harness this potent technology. Fast forward to today, and React’s ecosystem has grown to encompass a vast array of libraries and tools, each addressing different facets of modern web application development. Among the most remarkable of these tools is NEXT.js, which is why the demand to hire NEXT.js developers has significantly increased.
What is NEXT.js?
NEXT.js, developed by Vercel, is a React-based framework for building server-side rendering and static site generation web applications. It effectively marries the strengths of React, such as its component-oriented structure and unidirectional data flow, with features necessary for SEO-friendly, performant, and scalable web applications. This unique blend of capabilities is why many businesses are looking to hire NEXT.js developers.
This framework combines client-side and server-side rendering, pre-rendering, and route-based code splitting, providing developers a robust toolkit for building virtually any kind of web application. As more and more companies recognize the power of this framework, the demand to hire NEXT.js developers has surged.
Now, let’s deep dive into how React powers NEXT.js and explore examples of the unique capabilities of this exciting framework, providing insights into the skills that businesses should look for when they hire NEXT.js developers.
React Powered Pages
The fundamental unit in NEXT.js is the page. In the ‘pages‘ directory, every .js file becomes a route that gets automatically processed and rendered. Each page is essentially a React Component.
Example 1: Basic page creation
```jsx // pages/index.js import React from 'react'; const HomePage = () => ( <div> <h1>Welcome to My NEXT.js Site!</h1> <p>This is powered by React!</p> </div> ); export default HomePage; ```
This file creates a route at the root URL (“/”) of your application. When users visit your site, they’ll be greeted with a “Welcome to My NEXT.js Site!” message. This file effectively works as a React component.
React Component Lifecycle in NEXT.js
In React, the component lifecycle is a well-established concept. The lifecycle consists of various phases like mounting, updating, and unmounting. With NEXT.js, the lifecycle remains virtually the same but with an additional feature: the `getInitialProps` function.
`getInitialProps` enables server-side rendering in a page and allows it to fetch the necessary data to render. It runs on the server-side, but it can also run on the client-side when navigating to different pages using the `Link` component from NEXT.js.
Example 2: Using getInitialProps
```jsx // pages/post.js import React from 'react'; const PostPage = ({ post }) => ( <div> <h1>{post.title}</h1> <p>{post.content}</p> </div> ); PostPage.getInitialProps = async ({ query }) => { const response = await fetch(`https://api.myapi.com/posts/${query.id}`); const post = await response.json(); return { post }; }; export default PostPage; ```
In this example, `getInitialProps` fetches the data for a specific blog post from an API, based on the ID passed in the query string. The fetched data is then used to pre-render the `PostPage` on the server.
Hydration in NEXT.js
In a typical React application, we speak of rendering, but in NEXT.js, another term comes into play – hydration. When NEXT.js serves a page, it renders the page on the server-side (SSR) and sends this HTML to the browser. The browser then creates an instance of the React components in the page and attempts to attach it to the existing server-rendered HTML. This process is known as ‘hydration’.
Example 3: Understanding Hydration
```jsx // pages/about.js import React from 'react'; const AboutPage = () => ( <div> <h1>About Us</h1> <p>We are a software development company.</p> </div> ); export default AboutPage; ```
In this example, when the ‘About Us’ page is requested, NEXT.js will render the page on the server and send this HTML to the client. React on the client-side then takes this HTML, creates the React components, and ‘hydrates’ the HTML with these components, making the page fully interactive.
NEXT.js and React Hooks
React Hooks, introduced in React 16.8, let you use state and other React features without writing a class. NEXT.js fully supports the usage of React Hooks, allowing for functional components and hooks to handle lifecycle events and state.
Example 4: Using React Hooks in NEXT.js
```jsx // pages/counter.js import React, { useState } from 'react'; const CounterPage = () => { const [count, setCount] = useState(0); return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> ); }; export default CounterPage; ```
In this example, the `useState` hook is used to manage the state for the counter in a functional component. This showcases how the power of React Hooks extends to NEXT.js.
Routing in NEXT.js
While React has libraries like `react-router-dom` for client-side routing, NEXT.js provides file-system based routing out of the box. The routing works by correlating a file in the ‘pages’ directory to a route based on its name and location.
Example 5: File-system based routing
Suppose you have a file named `contact.js` in your ‘pages’ directory.
```jsx // pages/contact.js import React from 'react'; const ContactPage = () => ( <div> <h1>Contact Us</h1> <p>Email us at contact@mywebsite.com</p> </div> ); export default ContactPage; ```
This will automatically generate a ‘/contact’ route in your NEXT.js app, displaying the content of the `ContactPage` component when visited.
Conclusion
The fusion of React and NEXT.js offers a dynamic and delightful development experience, solving challenges inherent in creating large-scale, production-grade web applications. The real power of NEXT.js stems from its React foundation – embracing its component-based approach, life-cycle methods, hydration, hooks, and file-system based routing. This makes NEXT.js a formidable framework for building server-rendered React apps, static websites, and even mobile applications.
In the contemporary web development arena, React’s continuous growth and the subsequent evolution of NEXT.js offer significant capabilities, marking the future of web development. It’s these factors that are driving the growing demand to hire NEXT.js developers, as they are well-equipped to build fast, user-friendly, and SEO-optimized web applications with superior efficiency.
Table of Contents
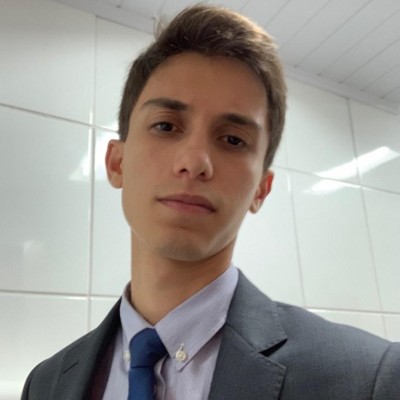
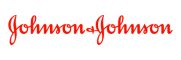