Leveraging Machine Learning in Node.js with TensorFlow.js
Machine learning (ML) has become an essential component in modern software development, enabling applications to learn from data and make predictions or decisions. TensorFlow.js, a JavaScript library developed by Google, brings the power of machine learning to the JavaScript ecosystem, including Node.js. This article explores how TensorFlow.js can be used with Node.js to build intelligent applications and provides practical examples to get you started.
Understanding TensorFlow.js
TensorFlow.js is an open-source library that allows developers to build and deploy machine learning models directly in the browser or on Node.js. With TensorFlow.js, you can train models from scratch, use pre-trained models, and perform various machine learning tasks, all within your JavaScript environment.
Using TensorFlow.js in Node.js
Node.js, known for its non-blocking I/O and scalability, complements TensorFlow.js by enabling server-side machine learning tasks. Below, we explore how to leverage TensorFlow.js with Node.js through practical examples.
1. Setting Up TensorFlow.js in Node.js
To start using TensorFlow.js with Node.js, you first need to install the `@tensorflow/tfjs` package. This can be done using npm.
```bash npm install @tensorflow/tfjs ```
Example: Basic TensorFlow.js Usage
Here’s a simple example of how to use TensorFlow.js to perform basic tensor operations.
```javascript const tf = require('@tensorflow/tfjs'); // Create a tensor const tensor = tf.tensor([1, 2, 3, 4], [2, 2]); // Perform an operation const result = tensor.add(tf.scalar(10)); result.print(); // Outputs: [[11, 12], [13, 14]] ```
2. Training a Machine Learning Model
TensorFlow.js allows you to train custom models directly in Node.js. Here’s an example of training a simple neural network model to perform linear regression.
Example: Training a Linear Regression Model
```javascript const tf = require('@tensorflow/tfjs'); // Create a model const model = tf.sequential(); model.add(tf.layers.dense({units: 1, inputShape: [1]})); // Compile the model model.compile({optimizer: 'sgd', loss: 'meanSquaredError'}); // Prepare training data const xs = tf.tensor1d([1, 2, 3, 4]); const ys = tf.tensor1d([1, 2, 3, 4]); // Train the model model.fit(xs, ys, {epochs: 10}).then(() => { // Make predictions model.predict(tf.tensor2d([5], [1, 1])).print(); // Outputs: [5] }); ```
3. Using Pre-Trained Models
TensorFlow.js provides access to various pre-trained models that can be used for tasks like image classification, object detection, and natural language processing.
Example: Using a Pre-Trained Model for Image Classification
```javascript const tf = require('@tensorflow/tfjs'); require('@tensorflow/tfjs-node'); // for Node.js support const mobilenet = require('@tensorflow-models/mobilenet'); const fs = require('fs'); const { Image } = require('canvas'); // Load a pre-trained model mobilenet.load().then(async (model) => { // Load an image const imageBuffer = fs.readFileSync('image.jpg'); const img = new Image(); img.src = imageBuffer; // Classify the image const predictions = await model.classify(img); console.log(predictions); }); ```
4. Integrating TensorFlow.js with Other Node.js Modules
TensorFlow.js can be integrated with other Node.js modules to enhance functionality. For instance, combining TensorFlow.js with `express` to create an API that performs image classification.
Example: Building an Image Classification API
```javascript const express = require('express'); const tf = require('@tensorflow/tfjs'); require('@tensorflow/tfjs-node'); const mobilenet = require('@tensorflow-models/mobilenet'); const { Image } = require('canvas'); const fs = require('fs'); const app = express(); const port = 3000; let model; async function loadModel() { model = await mobilenet.load(); } loadModel(); app.post('/classify', express.json(), async (req, res) => { const imageBuffer = Buffer.from(req.body.image, 'base64'); const img = new Image(); img.src = imageBuffer; const predictions = await model.classify(img); res.json(predictions); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); }); ```
Conclusion
TensorFlow.js brings powerful machine learning capabilities to Node.js, allowing developers to build intelligent applications efficiently. From basic tensor operations to training custom models and integrating pre-trained models, TensorFlow.js offers a versatile toolkit for enhancing your applications with machine learning. By leveraging these capabilities, you can create more dynamic and responsive solutions that leverage the full potential of AI.
Further Reading:
Table of Contents
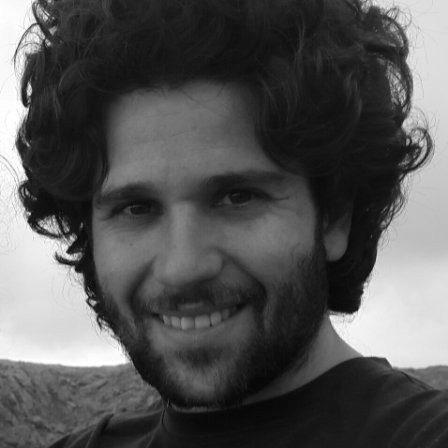
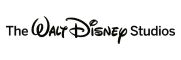