PHP Q & A
What is an array?
In PHP, an array is a versatile and fundamental data structure that allows you to store and manage collections of data in a single variable. Arrays are widely used in PHP and are essential for tasks like storing lists of items, managing data, and iterating over elements efficiently.
Here are some key characteristics and information about PHP arrays:
- Definition: An array in PHP is a composite data type that can hold multiple values. These values can be of different data types, such as integers, strings, objects, or even other arrays.
- Indexed Arrays: The most common type of PHP array is the indexed array. In an indexed array, elements are stored with numeric keys, starting from 0 by default. For example:
```php $fruits = array("apple", "banana", "cherry"); ```
- Associative Arrays: In associative arrays, elements are stored with named keys. These keys can be strings or integers, and they allow you to access array elements using descriptive labels. For example:
```php $person = array("first_name" => "John", "last_name" => "Doe", "age" => 30); ```
- Multidimensional Arrays: PHP also supports multidimensional arrays, which are arrays containing other arrays as elements. They are useful for representing complex data structures, such as tables or matrices.
```php $matrix = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); ```
- Array Functions: PHP provides a wide range of built-in array functions for sorting, searching, filtering, and manipulating arrays. These functions make it easier to work with arrays and perform common operations efficiently.
- Dynamic Sizing: Arrays in PHP are dynamically sized, meaning you can add or remove elements as needed without specifying a fixed size beforehand.
- Looping through Arrays: You can use loops like `for`, `foreach`, or array-specific functions to iterate through array elements and perform actions on them.
- Accessing Array Elements: To access array elements, you use square brackets `[]` and specify the index or key. For example:
```php echo $fruits[0]; // Output: apple echo $person["first_name"]; // Output: John ```
PHP arrays are versatile data structures used for storing and organizing collections of data efficiently. They are an essential part of PHP programming and are employed in various scenarios, from simple lists to complex data structures, making them a fundamental concept in PHP development.
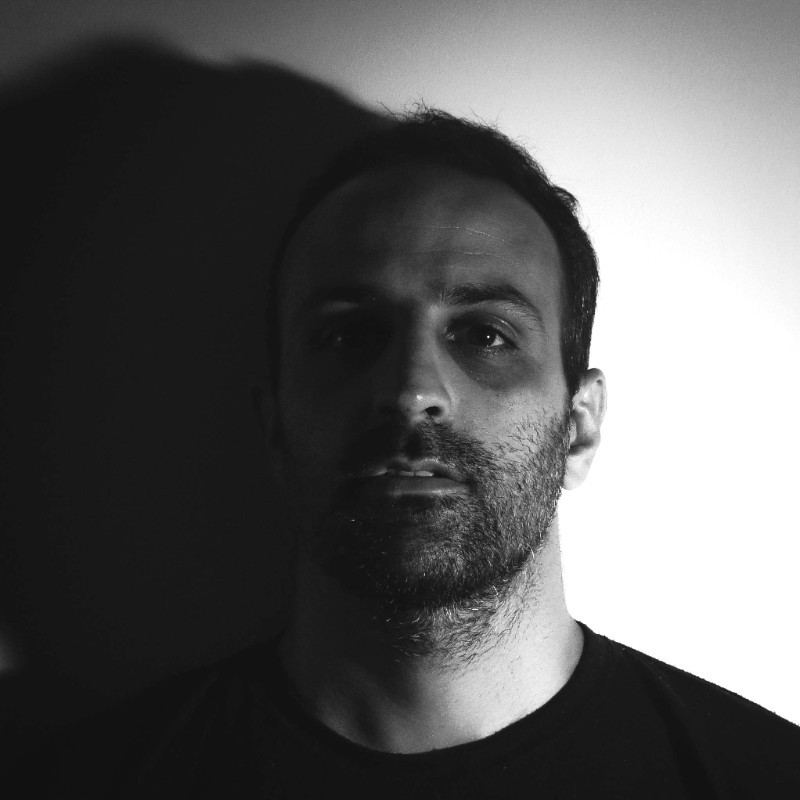
Previously at
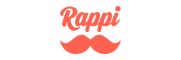
Full Stack Engineer with extensive experience in PHP development. Over 11 years of experience working with PHP, creating innovative solutions for various web applications and platforms.