Unlocking the Power of PHP’s array_filter() Function
In PHP, handling and manipulating arrays is a common task in many web development scenarios. One powerful tool for managing arrays is the array_filter() function. This function allows you to filter elements of an array based on a callback function, making it easier to work with complex data sets and streamline your code.
What is array_filter()?
The array_filter() function filters elements of an array using a callback function. The callback function determines which elements to keep based on a condition you define. This function returns a new array containing only the elements that pass the callback test.
Syntax:
```php array_filter(array $array, callable $callback = null, int $flag = 0): array ```
- $array: The input array to be filtered.
- $callback: A callback function to use for filtering. If no callback is provided, all entries of the input array with a non-false value will be included in the result.
- $flag: A flag to modify the behavior of the callback function.
Basic Usage
Here’s a simple example to demonstrate how array_filter() works:
```php $numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; // Define a callback function to filter out even numbers function is_odd($number) { return $number % 2 !== 0; } // Use array_filter with the callback function $odd_numbers = array_filter($numbers, 'is_odd'); print_r($odd_numbers); ```
Output:
```php Array ( [0] => 1 [2] => 3 [4] => 5 [6] => 7 [8] => 9 ) ```
In this example, array_filter() filters out even numbers, leaving only odd numbers in the resulting array.
Using Anonymous Functions
You can also use anonymous functions (closures) with array_filter(), which is often more concise:
```php $numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; // Use an anonymous function to filter out odd numbers $even_numbers = array_filter($numbers, function($number) { return $number % 2 === 0; }); print_r($even_numbers); ```
Output:
```php Array ( [1] => 2 [3] => 4 [5] => 6 [7] => 8 [9] => 10 ) ```
Advanced Usage: Using Flags
The $flag parameter can modify the behavior of array_filter(). For example, using ARRAY_FILTER_USE_KEY allows you to filter based on array keys:
```php $assoc_array = [ 'a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, ]; // Filter array by keys $filtered_array = array_filter($assoc_array, function($key) { return $key === 'a' || $key === 'c'; }, ARRAY_FILTER_USE_KEY); print_r($filtered_array); ```
Output:
Array ( [a] => 1 [c] => 3 ) ```
Practical Examples
Filtering Non-Empty Values:
```php $data = ["apple", "", "banana", null, "cherry"]; // Remove empty values $filtered_data = array_filter($data); print_r($filtered_data); ```
Output:
```php Array ( [0] => apple [2] => banana [4] => cherry ) ```
Filtering Users Based on Age:<br /><br />
```php $users = [ ['name' => 'Alice', 'age' => 25], ['name' => 'Bob', 'age' => 30], ['name' => 'Charlie', 'age' => 20], ]; // Get users older than 25 $filtered_users = array_filter($users, function($user) { return $user['age'] > 25; }); print_r($filtered_users); ```
Output:
```php Array ( [1] => Array ( [name] => Bob [age] => 30 ) ) ```
Conclusion
The array_filter() function in PHP is a versatile tool for filtering array elements based on specific conditions. Whether you’re working with simple arrays or more complex associative arrays, array_filter() can simplify your code and make data processing more efficient.
Further Reading
Table of Contents
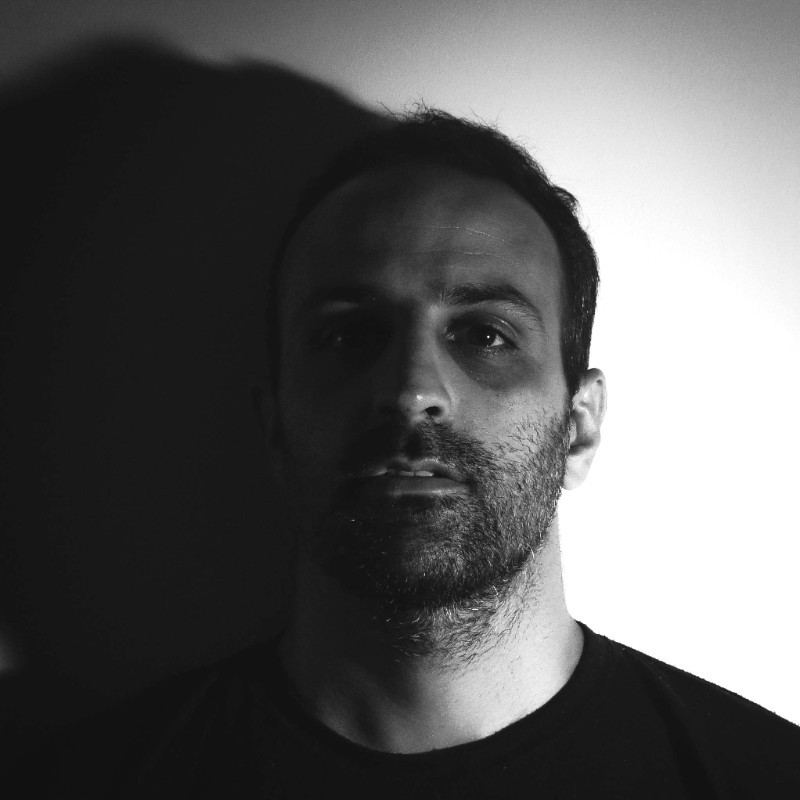
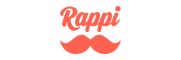