A Comprehensive Guide to PHP’s array_key_first() Function
In PHP, arrays are a fundamental data structure used to store multiple values in a single variable. Manipulating arrays efficiently is crucial for optimal performance and cleaner code. One such function that enhances array manipulation is array_key_first(). This function, introduced in PHP 7.3, provides a straightforward way to retrieve the first key of an array. This blog will delve into the array_key_first() function, exploring its usage, advantages, and practical examples.
What is array_key_first()?
The array_key_first() function returns the first key of an array. This is particularly useful when dealing with associative arrays where the order of keys can be significant. By obtaining the first key, you can easily access the corresponding value or perform operations based on the initial key.
Syntax
```php array_key_first(array $array): int|string|null ```
- $array: The input array from which the first key will be retrieved.
- Return Value: The function returns the first key of the array if it exists; otherwise, it returns null.
How to Use array_key_first()
Here are some practical examples to illustrate how array_key_first() can be used effectively.
Example 1: Basic Usage
Let’s start with a simple example where we retrieve the first key from an associative array.
```php <?php $array = [ 'first' => 'apple', 'second' => 'banana', 'third' => 'cherry' ]; $firstKey = array_key_first($array); echo "The first key is: " . $firstKey; // Output: The first key is: first ?> ```
In this example, array_key_first() returns ‘first’, which is the first key of the array.
Example 2: Handling Empty Arrays
When working with potentially empty arrays, it’s essential to handle the case where array_key_first() might return null.
```php <?php $array = []; $firstKey = array_key_first($array); if ($firstKey === null) { echo "The array is empty."; } else { echo "The first key is: " . $firstKey; } ?> ```
This code checks if the array is empty and handles the scenario appropriately.
Example 3: Associative vs. Indexed Arrays
array_key_first() can be used with both associative and indexed arrays. However, the behavior differs slightly based on the array type.
php Copy code ```php <?php $assocArray = [ 'a' => 'alpha', 'b' => 'beta' ]; $indexArray = ['apple', 'banana']; echo "First key of associative array: " . array_key_first($assocArray) . "\n"; // Output: a echo "First key of indexed array: " . array_key_first($indexArray) . "\n"; // Output: 0 ?> ```
In associative arrays, array_key_first() returns the first defined key, while for indexed arrays, it returns 0, the first numeric index.
Advantages of Using array_key_first()
- Simplicity: The function provides a simple and direct way to access the first key without needing additional logic or iteration.
- Readability: Code becomes more readable and maintainable by clearly expressing the intent to retrieve the first key.
- Performance: Since the function operates in constant time, it performs efficiently even with large arrays.
Conclusion
The array_key_first() function is a valuable tool for PHP developers working with arrays. Its simplicity and efficiency make it a great choice for tasks involving array manipulation and key retrieval. By understanding and utilizing this function, you can enhance your PHP code’s readability and performance.
Further Reading
- PHP Manual: array_key_first() – Official PHP documentation for array_key_first().
- PHP Arrays: Understanding Indexed and Associative Arrays – A comprehensive guide to PHP arrays.
- Effective PHP Array Manipulation Techniques – Tips and techniques for working with arrays in PHP.
Table of Contents
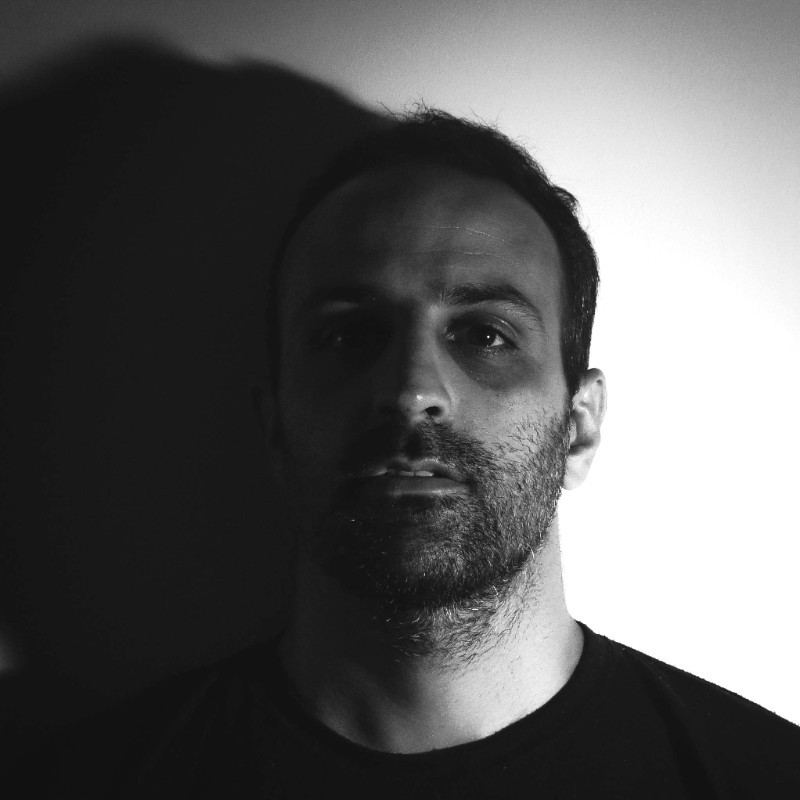
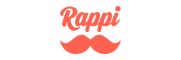