PHP’s array_key_last() Function: Finding the Last Key
PHP provides a wide range of built-in functions to work with arrays, making it a versatile language for handling various data structures. One such function is array_key_last(), introduced in PHP 7.3, which simplifies the process of retrieving the last key from an array. In this blog, we’ll explore the array_key_last() function, its usage, and practical applications in PHP development.
What is the array_key_last() Function?
The array_key_last() function returns the last key of an array without modifying the original array. This function is particularly useful when you need to access the last element of an array, especially when working with associative arrays or arrays with mixed keys.
Syntax
```php array_key_last(array $array): mixed ```
- $array: The input array from which to retrieve the last key.
- Returns: The last key of the array, or NULL if the array is empty.
Using array_key_last() in PHP
Let’s look at some examples to understand how the array_key_last() function works in different scenarios.
Example 1: Numeric Array
```php <?php $fruits = ['apple', 'banana', 'cherry']; $lastKey = array_key_last($fruits); echo $lastKey; // Output: 2 ?> ```
In this example, the function returns 2, which is the last key of the numeric array $fruits.
Example 2: Associative Array
```php <?php $user = [ 'name' => 'Alice', 'email' => 'alice@example.com', 'age' => 30 ]; $lastKey = array_key_last($user); echo $lastKey; // Output: 'age' ?> ```
For an associative array like $user, array_key_last() returns ‘age’, the key of the last element.
Example 3: Empty Array
```php <?php $emptyArray = []; $lastKey = array_key_last($emptyArray); var_dump($lastKey); // Output: NULL ?> ```
If the input array is empty, the function returns NULL.
Practical Applications
The array_key_last() function can be particularly useful in various practical scenarios:
Tracking the Latest Data
When dealing with data that arrives sequentially, such as logs or time-series data, you may need to access the most recent entry quickly. Using array_key_last(), you can efficiently find the last key and retrieve the corresponding value.
Managing Session Data
In web applications, session data often involves tracking the latest user actions. By storing session data in an associative array, you can use array_key_last() to access the most recent action performed by the user.
Implementing Stacks
For implementing stack-like data structures, where the last inserted element needs to be accessed or removed, array_key_last() can help identify the key of the top element without iterating through the entire array.
Limitations and Considerations
While array_key_last() is a useful function, there are some considerations to keep in mind:
- PHP Version: This function is only available in PHP 7.3 and later. Ensure your server environment supports this version or higher.
- Performance: Although array_key_last() is efficient, if your application frequently needs to access the last element of an array, consider using other data structures, like a deque, for better performance in certain cases.
Conclusion
The array_key_last() function is a handy addition to PHP’s array manipulation toolkit. It offers a straightforward way to retrieve the last key from an array, simplifying tasks that involve accessing the most recent data. Whether you’re working with numeric, associative, or mixed arrays, this function can help streamline your code and improve readability.
Further Reading
Table of Contents
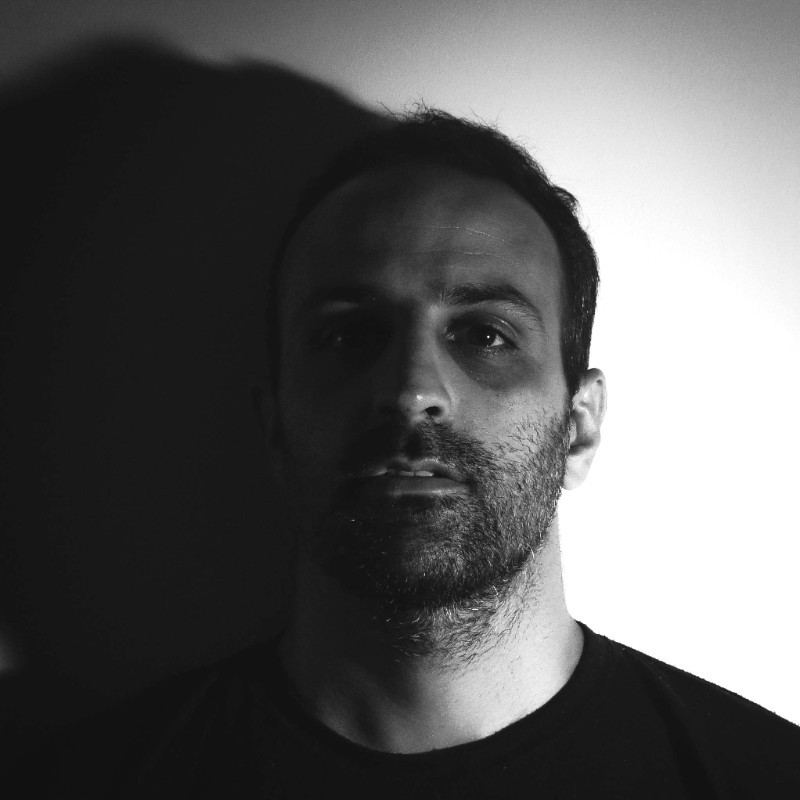
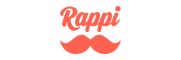