Mastering PHP’s array_keys() Function
PHP’s array manipulation capabilities are extensive, and one of the most versatile functions in this toolkit is array_keys(). Whether you’re working with associative arrays or indexed arrays, array_keys() can help you extract and manipulate the keys of an array efficiently. In this guide, we’ll dive deep into the usage, parameters, and practical examples of this function to help you master it.
What is array_keys()?
The array_keys() function returns all the keys from an array. It can also filter the keys based on specific values, making it a powerful tool for various array operations.
Basic Syntax
```php array_keys(array $array, mixed $search_value = null, bool $strict = false): array ```
- $array: The input array from which to retrieve keys.
- $search_value: (Optional) If specified, only the keys containing these values are returned.
- $strict: (Optional) If set to true, the function uses strict comparison (===) for value matching.
Basic Usage
Let’s start with a basic example of using array_keys() to extract all keys from an associative array.
```php <?php $fruits = [ 'a' => 'apple', 'b' => 'banana', 'c' => 'cherry', ]; $keys = array_keys($fruits); print_r($keys); ?> ```
Output:
``` Array ( [0] => a [1] => b [2] => c ) ```
In this example, array_keys() extracts the keys a, b, and c from the $fruits array.
Filtering Keys by Value
The array_keys() function can also filter keys by a specific value. This is useful when you need to find keys associated with a particular value in the array.
```php <?php $numbers = [ 0 => 100, 1 => 200, 2 => 100, 3 => 300, ]; $keys = array_keys($numbers, 100); print_r($keys); ?>
Output:
``` Array ( [0] => 0 [1] => 2 ) ```
Here, array_keys($numbers, 100) returns the keys 0 and 2 because they correspond to the value 100.
Strict Comparison
By default, array_keys() uses loose comparison. However, you can enable strict comparison to ensure that the value types match as well.
```php <?php $mixed = [ 'int' => 1, 'string' => '1', 'float' => 1.0, ]; $keys = array_keys($mixed, '1', true); print_r($keys); ?> ```
Output:
``` Array ( [0] => string ) ```
In this example, only the key ‘string’ is returned because strict is set to true, and only the string ‘1’ matches the value exactly.
Practical Applications
Checking for Duplicate Keys
You can use array_keys() to identify duplicate values in an array by comparing the number of keys.
```php <?php $names = [ 'John', 'Jane', 'John', 'Doe' ]; $keys = array_keys(array_count_values($names), 2); print_r($keys); ?> ```
Output:
``` Array ( [0] => John ) ```
This script finds that the name ‘John’ appears twice in the array.
Converting Object Properties to Array Keys
For working with objects, array_keys() can be combined with get_object_vars() to convert object properties into an array of keys.
```php <?php class Person { public $name; public $age; public $city; } $person = new Person(); $person->name = "Alice"; $person->age = 30; $person->city = "New York"; $keys = array_keys(get_object_vars($person)); print_r($keys); ?>
Output:
``` Array ( [0] => name [1] => age [2] => city ) ```
Conclusion
The array_keys() function is a versatile and powerful tool in PHP for handling array keys. Whether you need to extract all keys, filter them by values, or use strict comparison, this function provides a simple and efficient solution. By mastering array_keys(), you can enhance your array manipulation skills and write more efficient PHP code.
Further Reading
Table of Contents
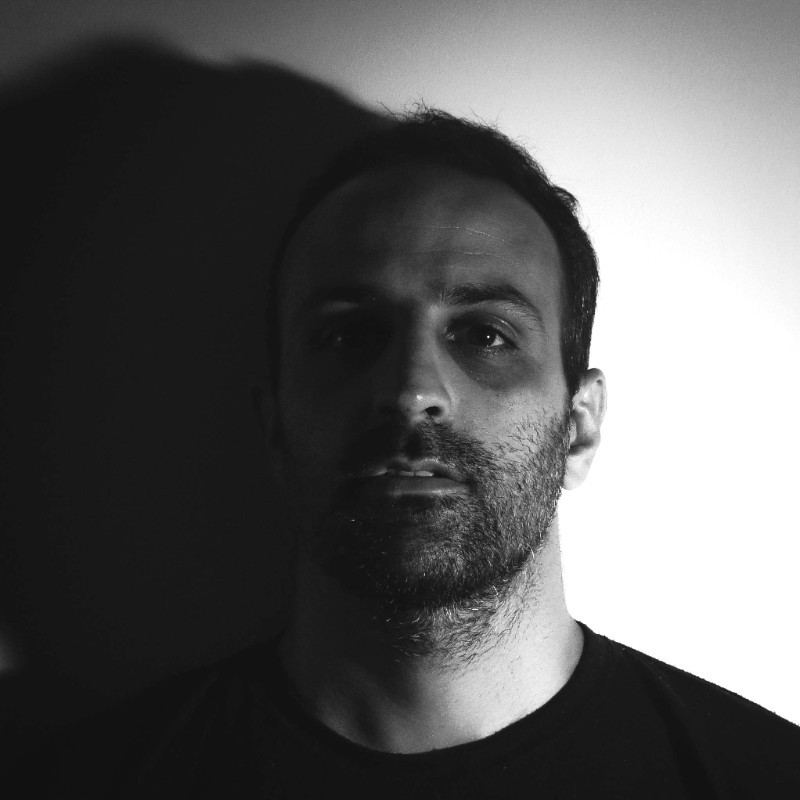
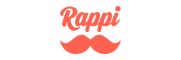