What is autoloading?
Autoloading in PHP is a mechanism that simplifies the process of including class files when they are needed. It automates the loading of classes, ensuring that the required class files are included only when they are used in the code. Autoloading is a critical feature for managing class dependencies in large PHP applications and helps keep code organized and efficient.
Key Concepts in Autoloading:
- Class-File Mapping:
– Autoloading relies on a mapping between class names and the corresponding file paths. This mapping is typically based on a specific naming convention, such as PSR-4 (PHP-FIG Standard Recommendation 4).
- Spl_autoload_register():
– PHP provides the `spl_autoload_register()` function, which allows you to register custom autoload functions. These functions are called automatically when an undefined class is encountered.
- PSR-4 Autoloading:
– The PSR-4 autoloading standard specifies a predictable class-to-file mapping. According to PSR-4, class namespaces correspond to directory structures, and class names map to file names.
- Composer:
– Composer, a popular PHP dependency manager, simplifies autoloading by generating an autoloader based on your project’s dependencies and PSR-4 conventions. It automatically manages the inclusion of class files for you.
Example:
```php // Register an autoloader function spl_autoload_register(function ($className) { // Convert class name to file path based on PSR-4 $filePath = str_replace('\\', DIRECTORY_SEPARATOR, $className) . '.php'; // Load the class file if it exists if (file_exists($filePath)) { include $filePath; } }); // Now, when you create an object, PHP will automatically load the class file if needed $obj = new \MyNamespace\MyClass(); ```
Autoloading simplifies the management of class dependencies, eliminates the need for manual `include` or `require` statements, and enhances code maintainability by ensuring that only necessary class files are loaded, improving the efficiency of your PHP applications.
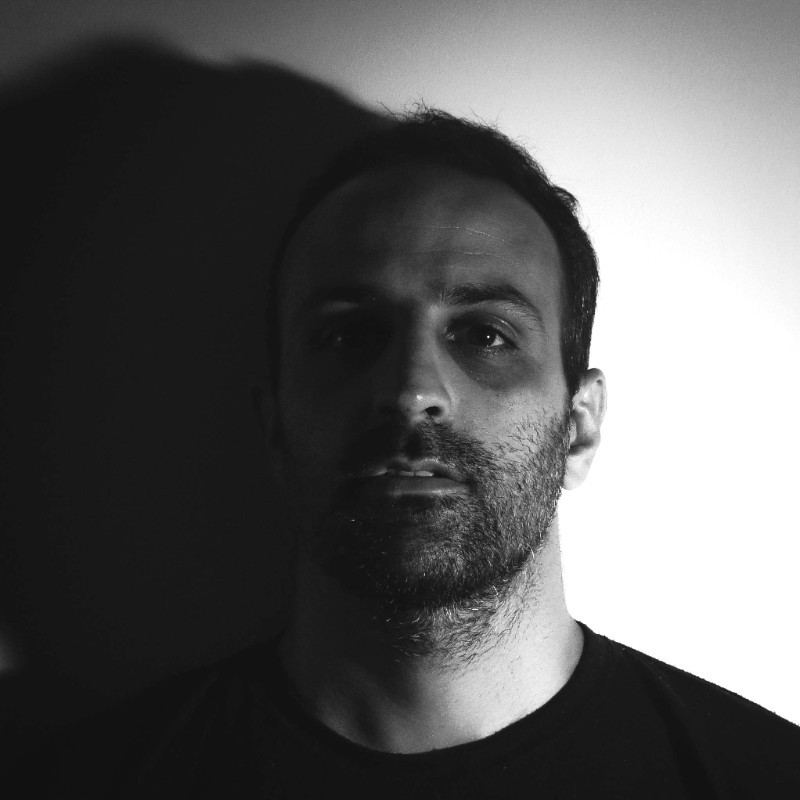
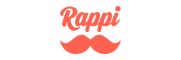