How to use cURL in PHP for HTTP requests?
cURL (Client URL) is a powerful library for making HTTP requests in PHP. It allows you to interact with web services, fetch data from remote servers, and perform various HTTP operations. Here’s a step-by-step guide on how to use cURL in PHP for HTTP requests:
- Initialize cURL:
To get started, you need to initialize a cURL session using the `curl_init()` function:
```php $ch = curl_init(); ```
- Set cURL Options:
Configure cURL with the necessary options, such as the target URL, request method, headers, and data to send. You can use functions like `curl_setopt()` to set these options:
```php curl_setopt($ch, CURLOPT_URL, 'https://example.com/api/resource'); curl_setopt($ch, CURLOPT_HTTPHEADER, ['Content-Type: application/json']); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Return the response instead of outputting it. curl_setopt($ch, CURLOPT_POST, true); // Use POST method. curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode(['key' => 'value'])); // JSON data to send. ```
- Execute the Request:
Use `curl_exec()` to execute the cURL request and retrieve the response:
```php $response = curl_exec($ch); ```
- Check for Errors:
It’s important to check for cURL errors after the request:
```php if (curl_errno($ch)) { echo 'cURL error: ' . curl_error($ch); } ```
- Close the cURL Session:
Always close the cURL session when you’re done:
```php curl_close($ch); ```
- Process the Response:
You can then process the response, which is stored in the `$response` variable, according to your application’s needs.
cURL in PHP is versatile and allows you to perform various types of HTTP requests, including GET, POST, PUT, DELETE, and more. It’s commonly used for integrating with RESTful APIs, fetching remote data, and interacting with web services. Make sure to handle errors and exceptions gracefully, and consider using try-catch blocks for more robust error handling in your PHP cURL code.
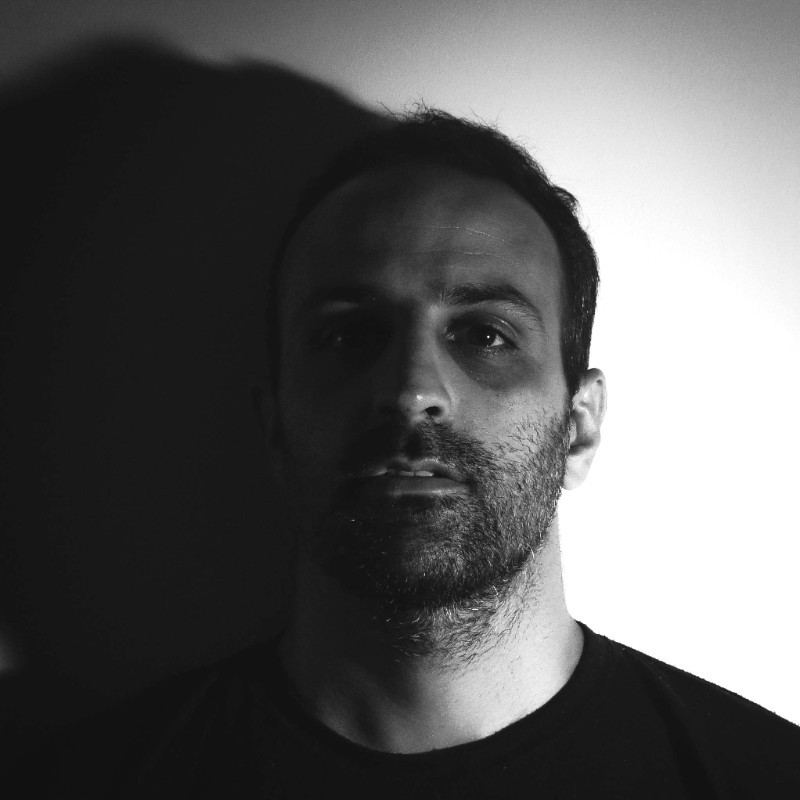
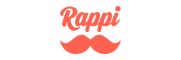