How to handle date and time?
Handling date and time in PHP is essential for a wide range of web applications, including scheduling events, displaying timestamps, or working with time-sensitive data. PHP offers a powerful set of functions and classes to manipulate and format dates and times.
- Current Date and Time:
– To retrieve the current date and time, you can use the `date()` function. It allows you to format the date and time in various ways.
```php $currentDate = date('Y-m-d'); // 2023-10-17 $currentTime = date('H:i:s'); // 14:30:00 ```
- Creating Date Objects:
– PHP’s `DateTime` class enables you to work with dates and times as objects, providing more flexibility and functionality. You can create a `DateTime` object and manipulate it using methods.
```php $date = new DateTime('2023-10-17'); $date->add(new DateInterval('P1D')); // Add one day echo $date->format('Y-m-d'); // 2023-10-18 ```
- Date Arithmetic:
– You can perform arithmetic operations on dates and times using the `DateTime` class. For example, calculating the difference between two dates:
```php $date1 = new DateTime('2023-10-17'); $date2 = new DateTime('2023-10-20'); $interval = $date1->diff($date2); echo $interval->format('%R%a days'); // +3 days ```
- Timezones:
– PHP supports handling timezones, ensuring that date and time operations are accurate. You can set the timezone using `date_default_timezone_set()` or directly on a `DateTime` object.
```php date_default_timezone_set('America/New_York'); $date = new DateTime('now'); ```
- Formatting:
– PHP provides robust formatting options for displaying dates and times in a human-readable format using `date()` or on `DateTime` objects with `format()`.
```php $formattedDate = $date->format('F j, Y - g:i A'); // October 17, 2023 - 2:30 PM ```
With these capabilities, PHP enables you to work with dates and times effectively, whether you need to display current date and time, perform date arithmetic, handle timezones, or format date and time information according to your application’s needs.
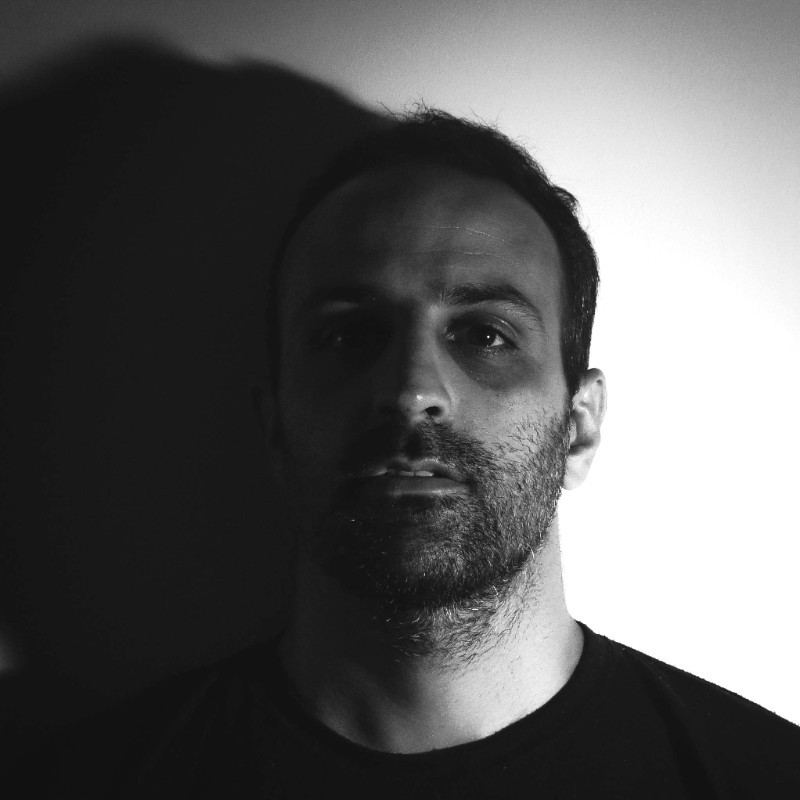
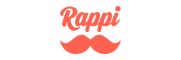