A Deep Dive into PHP’s end() Function
PHP, a popular server-side scripting language, provides numerous functions to manipulate arrays efficiently. Among these, the end() function is a particularly useful tool for developers who need to access the last element of an array. In this blog, we’ll explore the end() function in depth, understanding how it works, its use cases, and potential pitfalls.
What is the end() Function?
The end() function in PHP is designed to move the internal pointer of an array to its last element and return that element’s value. This function can be incredibly handy when working with arrays, especially in scenarios where you need to quickly access the last value without iterating through the entire array.
Syntax and Basic Usage
The basic syntax of the end() function is straightforward:
```php mixed end(array &$array) ```
- array: The array whose internal pointer should be moved to the last element.
- return value: The value of the last element in the array, or FALSE if the array is empty.
Example
```php $fruits = ['apple', 'banana', 'cherry']; $lastFruit = end($fruits); echo $lastFruit; // Outputs: cherry ```
In this example, end($fruits) moves the internal pointer to the last element of the $fruits array and returns its value, cherry.
How the end() Function Works
To fully grasp the end() function, it’s essential to understand PHP’s array internal pointer mechanism. PHP maintains an internal pointer for every array, which helps keep track of the current element. When end() is called, this pointer is moved to the last element of the array.
Use Cases for end()
Accessing the Last Element Efficiently
The most common use case for end() is when you need to access the last element of an array without knowing the number of elements beforehand. This is particularly useful in scenarios involving dynamic arrays where the size and content may vary.
```php $numbers = [1, 2, 3, 4, 5]; echo "The last number is " . end($numbers); // Outputs: The last number is 5 ```
Checking the Last Element for Conditional Logic
You can also use end() to conditionally check the value of the last element. For instance, in an e-commerce application, you might want to verify if the last item in a cart is eligible for a discount.
```php $cart = ['item1', 'item2', 'discounted_item']; if (end($cart) === 'discounted_item') { echo "You have a discounted item!"; } ```
Considerations and Pitfalls
end() Affects the Internal Pointer
It’s crucial to note that end() modifies the internal pointer of the array. If you subsequently use other functions that rely on the pointer’s position (e.g., current(), next()), they will now reference the last element. To avoid unexpected behavior, consider resetting the pointer with reset() if needed.
```php $colors = ['red', 'green', 'blue']; echo end($colors); // Outputs: blue echo current($colors); // Outputs: blue (since the pointer is now at the end) reset($colors); // Resets the pointer to the beginning echo current($colors); // Outputs: red ```
end() Returns FALSE for Empty Arrays
If end() is called on an empty array, it returns FALSE. Therefore, always validate the array content before relying on the function’s return value.
```php $emptyArray = []; if (end($emptyArray) === FALSE) { echo "The array is empty."; } ```
Conclusion
The end() function is a powerful yet simple tool in PHP’s array manipulation arsenal. It provides a quick and efficient way to access the last element of an array without the need for looping. However, developers should be mindful of its impact on the internal pointer and handle cases where the array might be empty.
By understanding and using the end() function correctly, you can streamline your PHP code and make array manipulations more intuitive and efficient.
Further Reading
Table of Contents
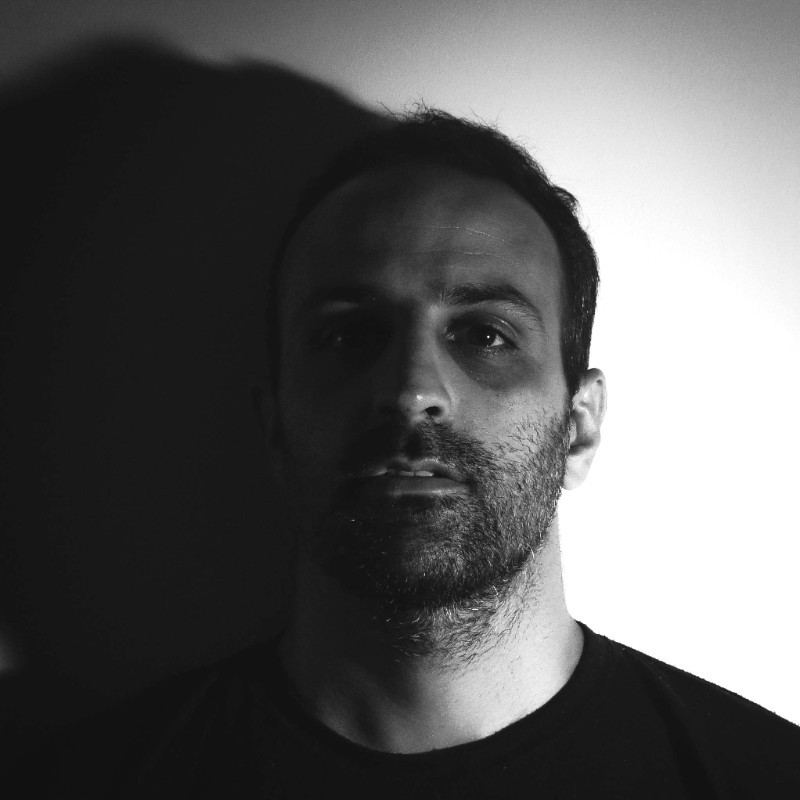
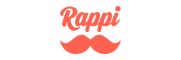